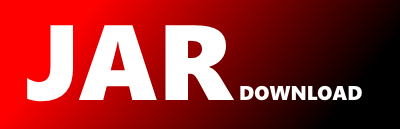
src-main.org.awakefw.sql.json.StatementHolderTransportJsonSimple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.json;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.Vector;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.sql.jsontypes.StaParms;
import org.json.simple.JSONObject;
import org.json.simple.JSONValue;
/**
*
* Method fromJson/toJson to transport with Json a structure with Json Simple
*
* @author Nicolas de Pomereu
*
*/
public class StatementHolderTransportJsonSimple {
private static final String SEPARATOR = "!--sth--!";
/** Debug flag */
private static boolean DEBUG = AwakeDebug
.isSet(StatementHolderTransportJsonSimple.class);
/**
* Convert to Json a unique of StatementHolder
*
* @return the instance converted to Json
*/
public static String toJson(StatementHolder statementHolder) {
int[] stP = statementHolder.getStP();
List list = new Vector();
for (int i = 0; i < stP.length; i++) {
list.add(stP[i]);
}
List columnIndexesAutogenerateKeys = new Vector();
int [] columnIndexesAutogenerateKeysArray = statementHolder.getColumnIndexesAutogenerateKeys();
for (int i = 0; i < columnIndexesAutogenerateKeysArray.length; i++) {
columnIndexesAutogenerateKeys.add(columnIndexesAutogenerateKeysArray[i]);
}
List columnNamesAutogenerateKeys = new Vector();
String [] columnNamesAutogenerateKeysArray = statementHolder.getColumnNamesAutogenerateKeys();
for (int i = 0; i < columnNamesAutogenerateKeysArray.length; i++) {
columnNamesAutogenerateKeys.add(columnNamesAutogenerateKeysArray[i]);
}
// Put all in map
Map map = new HashMap();
map.put("sql", statementHolder.getSql());
map.put("stP", list);
map.put("parmsT", statementHolder.getParmsT());
map.put("parmsV", statementHolder.getParmsV());
map.put("paramatersEncrypted", statementHolder.isParamatersEncrypted());
map.put("htmlEncodingOn", statementHolder.isHtmlEncodingOn());
map.put("autoGeneratedKeys", statementHolder.getAutoGeneratedKeys());
map.put("columnIndexesAutogenerateKeys", columnIndexesAutogenerateKeys);
map.put("columnNamesAutogenerateKeys", columnNamesAutogenerateKeys);
String theString = JSONValue.toJSONString(map);
debug("toJson():");
debug("sql : " + statementHolder.getSql());
debug("stP : " + list);
debug("parmsT: " + statementHolder.getParmsT());
debug("parmsV: " + statementHolder.getParmsV());
debug("columnIndexesAutogenerateKeys: " + columnIndexesAutogenerateKeys);
debug("columnNamesAutogenerateKeys : " + columnNamesAutogenerateKeys);
debug("");
// Clean all to release memory for GC
stP = null;
statementHolder = null;
map = null;
return theString;
}
/**
* Convert from a Json string a List of StatementHolder
*
* @return the StatementHolder list converted from Json
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public static StatementHolder fromJson(String jsonString) {
// Revert it
Object obj = JSONValue.parse(jsonString);
JSONObject mapBack = (JSONObject) obj;
String sql = (String) mapBack.get("sql");
List stPraw = (List) mapBack.get("stP");
Map parmsTraw = (Map) mapBack.get("parmsT");
Map parmsVraw = (Map) mapBack.get("parmsV");
Boolean paramatersEncrypted = (Boolean) mapBack
.get("paramatersEncrypted");
Boolean htmlEncodingOn = (Boolean) mapBack.get("htmlEncodingOn");
Long autoGeneratedKeys = (Long)mapBack.get("autoGeneratedKeys");
List columnIndexesAutogenerateKeys = (List) mapBack.get("columnIndexesAutogenerateKeys");
List columnNamesAutogenerateKeys = (List) mapBack.get("columnNamesAutogenerateKeys");
mapBack = null;
debug("toJson():");
debug("stP : " + stPraw);
debug("parmsTraw: " + parmsTraw);
debug("parmsVraw: " + parmsVraw);
int[] stP = new int[StaParms.NUM_PARMS];
for (int i = 0; i < stPraw.size(); i++) {
long myLong = (Long) stPraw.get(i);
stP[i] = (int) myLong;
}
Map parmsT = new TreeMap();
Set set = parmsTraw.keySet();
for (Iterator iterator = set.iterator(); iterator.hasNext();) {
String key = (String) iterator.next();
long value = (Long) parmsTraw.get(key);
parmsT.put(Integer.parseInt(key), (int) value);
}
Map parmsV = new TreeMap();
set = parmsVraw.keySet();
for (Iterator iterator = set.iterator(); iterator.hasNext();) {
String key = (String) iterator.next();
String value = (String) parmsVraw.get(key);
parmsV.put(Integer.parseInt(key), value);
}
debug("");
debug("stP : " + stPraw);
debug("parmsT:" + parmsT);
debug("parmsT:" + parmsV);
debug("columnIndexesAutogenerateKeys: " + columnIndexesAutogenerateKeys);
debug("columnNamesAutogenerateKeys : " + columnNamesAutogenerateKeys);
// Clean all to release memory for GC
obj = null;
StatementHolder statementHolder = new StatementHolder();
statementHolder.setSql(sql);
statementHolder.setStP(stP);
statementHolder.setParmsT(parmsT);
statementHolder.setParmsV(parmsV);
statementHolder.setParamatersEncrypted(paramatersEncrypted);
statementHolder.setHtmlEncodingOn(htmlEncodingOn);
statementHolder.setAutoGeneratedKeys(autoGeneratedKeys.intValue());
statementHolder.setColumnIndexesAutogenerateKeys(columnIndexesAutogenerateKeys);
statementHolder.setColumnNamesAutogenerateKeys(columnNamesAutogenerateKeys);
return statementHolder;
}
/**
* Convert to Json a List of StatementHolder
*
* @return the instance converted to Json
*/
public static String toJson(
List preparedStatementHolderList) {
debug("in StatementHolderTransport.toJson(List");
StringBuffer stringBuffer = new StringBuffer();
for (StatementHolder statementHolder : preparedStatementHolderList) {
String jsonString = toJson(statementHolder);
stringBuffer.append(SEPARATOR);
stringBuffer.append(jsonString);
}
String finalJson = stringBuffer.toString();
debug("finalJson: " + finalJson);
return finalJson;
}
/**
* Convert to Json a List of StatementHolder
*
* @return the instance converted to Json
*/
public static List fromJsonList(String jsonString) {
List statementHolders = new Vector();
debug("jsonString :" + jsonString + ":");
String[] result = jsonString.split(SEPARATOR);
for (int i = 0; i < result.length; i++) {
String theJsonString = result[i];
if (theJsonString.isEmpty()) {
continue;
}
debug("theJsonString :" + theJsonString + ":");
statementHolders.add(fromJson(theJsonString));
}
return statementHolders;
}
/**
* Debug
*
* @param s
*/
public static void debug(String s) {
if (DEBUG) {
System.out.println(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy