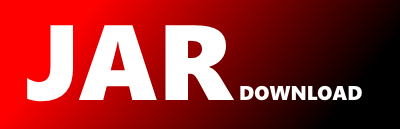
src-main.org.awakefw.sql.servlet.AwakeSqlConfiguratorCall Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import org.awakefw.sql.api.server.AwakeSqlConfigurator;
/**
*
* Calls AwakeSqlConfigurator methods using reflection
*
* @author Nicolas de Pomereu
*
*/
public class AwakeSqlConfiguratorCall {
/**
* Returns the result of AwakeSqlConfigurator.allowExecute
*
* @param awakeSqlConfigurator
* the AwakeSqlConfigurator instance
* @param username
* the client username
* @param connection
* The JDBC Connection
* @return the result of AwakeSqlConfigurator.allowExecute
* @throws IOException
* @throws SQLException
*/
public static boolean allowExecute(AwakeSqlConfigurator awakeSqlConfigurator,
String username, Connection connection) throws IOException,
SQLException {
return awakeSqlConfigurator.allowExecute(username, connection);
}
/**
* Returns the result of AwakeSqlConfigurator.allowExecuteUpdate
*
* @param awakeSqlConfigurator
* the AwakeSqlConfigurator instance
* @param username
* the client username
* @param connection
* The JDBC Connection
* @return the result of AwakeSqlConfigurator.allowExecuteUpdate
* @throws IOException
* @throws SQLException
*/
public static boolean allowExecuteUpdate(
AwakeSqlConfigurator awakeSqlConfigurator, String username,
Connection connection) throws IOException, SQLException {
return awakeSqlConfigurator.allowExecuteUpdate(username, connection);
}
/**
* Returns the result of AwakeSqlConfigurator.allowStatementClass
*
* @param awakeSqlConfigurator
* the AwakeSqlConfigurator instance
* @param username
* the client username
* @param connection
* The JDBC Connection
* @return the result of AwakeSqlConfigurator.allowStatementClass
* @throws IOException
* @throws SQLException
*/
public static boolean allowStatementClass(
AwakeSqlConfigurator awakeSqlConfigurator, String username,
Connection connection) throws IOException, SQLException {
return awakeSqlConfigurator.allowStatementClass(username, connection);
}
/**
* Returns the result of AwakeSqlConfigurator.allowGetMetaData
*
* @param awakeSqlConfigurator
* the AwakeSqlConfigurator instance
* @param username
* the client username
* @param connection
* The JDBC Connection
* @return the result of AwakeSqlConfigurator.allowGetMetaData
* @throws IOException
* @throws SQLException
*/
public static boolean allowGetMetaData(AwakeSqlConfigurator awakeSqlConfigurator,
String username, Connection connection) throws IOException,
SQLException {
return awakeSqlConfigurator.allowGetMetaData(username, connection);
}
/**
* Returns the result of AwakeSqlConfigurator.allowResultSetGetMetaData
*
* @param awakeSqlConfigurator
* the AwakeSqlConfigurator instance
* @param username
* the client username
* @param connection
* The JDBC Connection
* @return the result of AwakeSqlConfigurator.allowResultSetGetMetaData
* @throws IOException
* @throws SQLException
*/
public static boolean allowResultSetGetMetaData(AwakeSqlConfigurator awakeSqlConfigurator,
String username, Connection connection) throws IOException,
SQLException {
return awakeSqlConfigurator.allowResultSetGetMetaData(username, connection);
}
/*
public void runIfStatementRefused(String username, Connection connection,
String ipAddress, String sql, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy