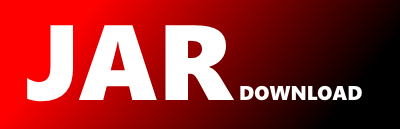
src-main.org.awakefw.sql.servlet.AwakeSqlManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.util.logging.Logger;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.api.server.DefaultAwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.commons.server.util.embed.TomcatEmbeddedStore;
import org.awakefw.file.api.server.AwakeFileConfigurator;
import org.awakefw.file.api.server.DefaultAwakeFileConfigurator;
import org.awakefw.file.api.server.fileaction.AwakeFileActionManager;
import org.awakefw.file.api.server.fileaction.DefaultAwakeFileActionManager;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.servlet.AwakeFileManager;
import org.awakefw.file.servlet.RequestInfoStore;
import org.awakefw.file.servlet.convert.HttpServletRequestConvertor;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.TransferStatus;
import org.awakefw.sql.api.server.AwakeSqlConfigurator;
import org.awakefw.sql.api.server.DefaultAwakeSqlConfigurator;
import org.awakefw.sql.version.Version;
/**
* Http JDBC Server
*
* @author Nicolas de Pomereu
*/
@SuppressWarnings("serial")
public class AwakeSqlManager extends HttpServlet {
private static boolean DEBUG = AwakeDebug.isSet(AwakeSqlManager.class);
public static String CR_LF = System.getProperty("line.separator");
private static final String AWAKE_SQL_CONFIGURATOR_CLASS_NAME = "awakeSqlConfiguratorClassName";
private AwakeCommonsConfigurator awakeCommonsConfigurator = null;
private AwakeFileConfigurator awakeFileConfigurator = null;
private AwakeSqlConfigurator awakeSqlConfigurator = null;
private AwakeFileActionManager awakeFileActionManager = null;
/** Awake SQL EE License info */
private String awakeLicenseKey = null;
private String email = null;
private String name = null;
private String company = null;
private String siteCompany = null;
/** Says that the AwakeServerLogger is ok and we can log the exceptions */
private boolean awakeServerLoggerOk = false;
/** The Exception thrown at init */
private Exception exception = null;
/* The init error message trapped */
private String initErrrorMesage = null;
/**
* Init
*/
public void init(ServletConfig config) throws ServletException {
super.init(config);
// Variable use to store the current name when loading, used to
// detail
// the exception in the catch clauses
String classNameToLoad = null;
String awakeCommonsConfiguratorClassName;
String awakeFileConfiguratorClassName;
String awakeSqlConfiguratorClassName;
String awakeFileActionManagerClassName;
if (TomcatEmbeddedStore.isTomcatEmbedded()) {
awakeCommonsConfiguratorClassName = TomcatEmbeddedStore
.getAwakeCommonsConfiguratorClassName();
awakeFileConfiguratorClassName = TomcatEmbeddedStore
.getAwakeFileConfiguratorClassName();
awakeSqlConfiguratorClassName = TomcatEmbeddedStore
.getAwakeSqlConfiguratorClassName();
awakeFileActionManagerClassName = TomcatEmbeddedStore
.getAwakeFileActionManagerClassName();
} else {
awakeCommonsConfiguratorClassName = config
.getInitParameter(AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME);
awakeFileConfiguratorClassName = config
.getInitParameter(AWAKE_FILE_CONFIGURATOR_CLASS_NAME);
awakeSqlConfiguratorClassName = config
.getInitParameter(AWAKE_SQL_CONFIGURATOR_CLASS_NAME);
awakeFileActionManagerClassName = config
.getInitParameter(AWAKE_FILE_ACTION_MANAGER_CLASS_NAME);
}
if (TomcatEmbeddedStore.isTomcatEmbedded()) {
awakeLicenseKey = TomcatEmbeddedStore.getAwakeLicenseKey();
email = TomcatEmbeddedStore.getEmail();
name = TomcatEmbeddedStore.getName();
company = TomcatEmbeddedStore.getCompany();
siteCompany = TomcatEmbeddedStore.getSiteCompany();
} else {
// Licenses info
awakeLicenseKey = config.getInitParameter("awakeLicenseKey");
email = config.getInitParameter("email");
name = config.getInitParameter("name");
company = config.getInitParameter("company");
siteCompany = config.getInitParameter("site");
}
try {
// Check spelling with first letter capitalized
if (awakeLicenseKey == null || awakeLicenseKey.isEmpty()) {
String capitalized = StringUtils.capitalize("awakeLicenseKey");
awakeLicenseKey = config.getInitParameter(capitalized);
}
if (email == null || email.isEmpty()) {
String capitalized = StringUtils.capitalize("email");
email = config.getInitParameter(capitalized);
}
if (name == null || name.isEmpty()) {
String capitalized = StringUtils.capitalize("name");
name = config.getInitParameter(capitalized);
}
if (company == null || company.isEmpty()) {
String capitalized = StringUtils.capitalize("company");
company = config.getInitParameter(capitalized);
}
if (siteCompany == null || siteCompany.isEmpty()) {
String capitalized = StringUtils.capitalize("companySite");
siteCompany = config.getInitParameter(capitalized);
}
if (awakeCommonsConfiguratorClassName == null
|| awakeCommonsConfiguratorClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AwakeFileManager.AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME);
awakeCommonsConfiguratorClassName = config
.getInitParameter(capitalized);
}
if (awakeFileConfiguratorClassName == null
|| awakeFileConfiguratorClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AwakeFileManager.AWAKE_FILE_CONFIGURATOR_CLASS_NAME);
awakeFileConfiguratorClassName = config
.getInitParameter(capitalized);
}
if (awakeSqlConfiguratorClassName == null
|| awakeSqlConfiguratorClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AWAKE_SQL_CONFIGURATOR_CLASS_NAME);
awakeSqlConfiguratorClassName = config
.getInitParameter(capitalized);
}
if (awakeFileActionManagerClassName == null
|| awakeFileActionManagerClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AwakeFileManager.AWAKE_FILE_ACTION_MANAGER_CLASS_NAME);
awakeFileActionManagerClassName = config
.getInitParameter(capitalized);
}
// Call the specific Awake SQL Configurator class to use
classNameToLoad = awakeCommonsConfiguratorClassName;
if (awakeCommonsConfiguratorClassName != null
&& !awakeCommonsConfiguratorClassName.isEmpty()) {
Class> c = Class.forName(awakeCommonsConfiguratorClassName);
awakeCommonsConfigurator = (AwakeCommonsConfigurator) c
.newInstance();
} else {
awakeCommonsConfigurator = new DefaultAwakeCommonsConfigurator();
}
// Immediately create the AwakeLogger
Logger logger = null;
try {
logger = awakeCommonsConfigurator.getLogger();
AwakeServerLogger.createLogger(logger);
AwakeServerLogger.log("Starting Awake SQL...");
awakeServerLoggerOk = true;
} catch (Exception e) {
exception = e;
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to create the Logger: " + logger
+ ". Reason: " + e.getMessage();
}
classNameToLoad = awakeFileConfiguratorClassName;
if (awakeFileConfiguratorClassName != null
&& !awakeFileConfiguratorClassName.isEmpty()) {
Class> c = Class.forName(awakeFileConfiguratorClassName);
awakeFileConfigurator = (AwakeFileConfigurator) c.newInstance();
} else {
awakeFileConfigurator = new DefaultAwakeFileConfigurator();
}
if (awakeSqlConfiguratorClassName != null
&& !awakeSqlConfiguratorClassName.isEmpty()) {
Class> c = Class.forName(awakeSqlConfiguratorClassName);
awakeSqlConfigurator = (AwakeSqlConfigurator) c.newInstance();
} else {
awakeSqlConfigurator = new DefaultAwakeSqlConfigurator();
}
classNameToLoad = awakeFileActionManagerClassName;
if (awakeFileActionManagerClassName != null
&& !awakeFileActionManagerClassName.isEmpty()) {
Class> c = Class.forName(awakeFileActionManagerClassName);
awakeFileActionManager = (AwakeFileActionManager) c
.newInstance();
} else {
awakeFileActionManager = new DefaultAwakeFileActionManager();
}
} catch (ClassNotFoundException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (ClassNotFoundException) Awake Configurator class: "
+ classNameToLoad;
exception = e;
} catch (InstantiationException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (InstantiationException) Awake Configurator class: "
+ classNameToLoad;
exception = e;
} catch (IllegalAccessException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (IllegalAccessException) Awake File Configurator class: "
+ classNameToLoad;
exception = e;
} catch (Exception e) {
initErrrorMesage = Tag.AWAKE_PRODUCT_FAIL
+ "Please contact support [email protected]";
exception = e;
}
if (awakeCommonsConfigurator == null) {
awakeCommonsConfiguratorClassName = AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
} else {
awakeCommonsConfiguratorClassName = awakeCommonsConfigurator
.getClass().getName();
}
if (awakeFileConfigurator == null) {
awakeFileConfiguratorClassName = AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
} else {
awakeFileConfiguratorClassName = awakeFileConfigurator.getClass()
.getName();
}
if (awakeFileActionManager == null) {
awakeFileActionManagerClassName = AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
} else {
awakeFileActionManagerClassName = awakeFileActionManager.getClass()
.getName();
}
if (awakeSqlConfigurator == null) {
awakeSqlConfiguratorClassName = AWAKE_SQL_CONFIGURATOR_CLASS_NAME;
} else {
awakeSqlConfiguratorClassName = awakeSqlConfigurator.getClass()
.getName();
}
System.out.println(Tag.AWAKE_START + Version.getVersion());
// System.out.println(Tag.AWAKE_START + this.getClass().getSimpleName()
// + " Servlet:");
System.out.println(Tag.AWAKE_START + this.getServletName()
+ " Servlet:");
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeCommonsConfiguratorClassName: "
+ CR_LF + Tag.AWAKE_START + " "
+ awakeCommonsConfiguratorClassName);
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeSqlConfiguratorClassName: " + CR_LF
+ Tag.AWAKE_START + " " + awakeSqlConfiguratorClassName);
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeFileConfiguratorClassName: " + CR_LF
+ Tag.AWAKE_START + " " + awakeFileConfiguratorClassName);
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeFileActionManagerClassName:" + CR_LF
+ Tag.AWAKE_START + " " + awakeFileActionManagerClassName);
if (exception == null) {
System.out.println(Tag.AWAKE_START
+ "Awake SQL Configurator Status: OK.");
} else {
String errorMessage1 = Tag.AWAKE_START
+ "Awake SQL Configurator Status: KO.";
String errorMessage2 = initErrrorMesage;
String errorMessage3 = ExceptionUtils.getStackTrace(exception);
System.out.println(errorMessage1);
System.out.println(errorMessage2);
System.out.println(errorMessage3);
if (awakeServerLoggerOk) {
AwakeServerLogger.log(errorMessage1);
AwakeServerLogger.log(errorMessage2);
AwakeServerLogger.log(errorMessage3);
}
}
}
/**
* Test the configurators main methods to see if they throw Exceptions
*/
/**
* Get request.
*/
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
String servletName = this.getClass().getSimpleName();
AwakeSqlManagerDoGetTester awakeSqlManagerDoGetTester = new AwakeSqlManagerDoGetTester();
awakeSqlManagerDoGetTester.doGetTest(servletName, out, exception,
awakeCommonsConfigurator, awakeFileConfigurator,
awakeSqlConfigurator, awakeFileActionManager);
}
/**
* Post request.
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws IOException {
// If init fail, say it cleanly to client, instead of bad 500 Servlet
// Error
if (exception != null) {
OutputStream out = response.getOutputStream();
writeLine(out, TransferStatus.SEND_FAILED);
writeLine(out, exception.getClass().getName()); // Exception class
// name
writeLine(out,
initErrrorMesage + " Reason: " + exception.getMessage()); // Exception
// message
writeLine(out, ExceptionUtils.getStackTrace(exception)); // stack
// trace
return;
}
// Store the host info in RequestInfoStore
RequestInfoStore.init(request);
debug("after RequestInfoStore.init(request);");
// Configure a repository (to ensure a secure temp location is used)
ServletContext servletContext = this.getServletConfig()
.getServletContext();
File servletContextTempDir = (File) servletContext
.getAttribute("javax.servlet.context.tempdir");
// Wrap the HttpServletRequest with HttpServletRequestEncrypted for
// parameters decryption
HttpServletRequestConvertor requestEncrypted = new HttpServletRequestConvertor(
request, awakeCommonsConfigurator);
ServerAwakeSqlDispatch dispatch = new ServerAwakeSqlDispatch();
debug("before dispatch.executeRequest()");
dispatch.executeRequest(requestEncrypted, response, servletContextTempDir,
awakeCommonsConfigurator, awakeFileConfigurator,
awakeSqlConfigurator, awakeFileActionManager);
}
/**
* Write a line of string on the servlet output stream. Will add the
* necessary CR_LF
*
* @param out
* the servlet output stream
* @param s
* the string to write
* @throws IOException
*/
private void writeLine(OutputStream out, String s) throws IOException {
out.write((s + CR_LF).getBytes());
}
/**
* Method called by children Servlet for debug purpose Println is done only
* if class name name is in awake-debug.ini
*/
public static void debug(String s) {
if (DEBUG) {
try {
AwakeServerLogger.log(s);
} catch (Exception e1) {
System.out.println(s);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy