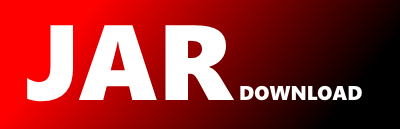
src-main.org.awakefw.sql.servlet.AwakeSqlManagerDoGetTester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
import static org.awakefw.file.servlet.AwakeFileManager.AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.StringReader;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.commons.server.util.embed.TomcatEmbeddedStore;
import org.awakefw.file.api.server.AwakeFileConfigurator;
import org.awakefw.file.api.server.fileaction.AwakeFileActionManager;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.sql.api.server.AwakeSqlConfigurator;
import org.awakefw.sql.version.Version;
/**
* @author Nicolas de Pomereu Test AwakeSqlManager doGet method
*/
public class AwakeSqlManagerDoGetTester {
private static boolean DEBUG = AwakeDebug.isSet(AwakeSqlManager.class);
public static String CR_LF = System.getProperty("line.separator");
public static final String AWAKE_SQL_CONFIGURATOR_CLASS_NAME = "awakeSqlConfiguratorClassName";
/** The init error message trapped */
private String initErrrorMesage = null;
public void doGetTest(String servletName, PrintWriter out,
Exception exception,
AwakeCommonsConfigurator awakeCommonsConfigurator,
AwakeFileConfigurator awakeFileConfigurator,
AwakeSqlConfigurator awakeSqlConfigurator, AwakeFileActionManager awakeFileActionManager
) {
try {
// ok for servlet display
String status = ""
+ "OK & Running.";
// Test configurators, only if not already thrown Exception:
if (exception == null) {
debug("before ConfiguratorMethodsTester call");
ConfiguratorMethodsTester configuratorMethodsTester = new ConfiguratorMethodsTester(
awakeCommonsConfigurator, awakeFileConfigurator);
configuratorMethodsTester.testMethods();
exception = configuratorMethodsTester.getException();
initErrrorMesage = configuratorMethodsTester
.getInitErrrorMesage();
}
if (exception != null) {
String stackTrace = ExceptionUtils.getStackTrace(exception);
if (TomcatEmbeddedStore.isTomcatEmbedded()) {
status = initErrrorMesage + CR_LF + stackTrace;
out.println(status);
return;
} else {
BufferedReader bufferedReader = new BufferedReader(
new StringReader(stackTrace));
StringBuffer sb = new StringBuffer();
String line = null;
while ((line = bufferedReader.readLine()) != null) {
// All subsequent lines contain the result
sb.append(line);
sb.append("
");
}
status = ""
+ initErrrorMesage + "
" + sb.toString();
}
}
// ok for tomcat embededed display
if (TomcatEmbeddedStore.isTomcatEmbedded()) {
// Tomcat is embedded & running OK
status = "OK";
out.println(status);
TomcatEmbeddedStore.setAwakeSQLManagerStatusOk(true);
return;
}
String awakeCommonsConfiguratorClassName = null;
if (awakeCommonsConfigurator == null) {
awakeCommonsConfiguratorClassName = AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
} else {
awakeCommonsConfiguratorClassName = awakeCommonsConfigurator
.getClass().getName();
}
String awakeSqlConfiguratorClassName = null;
if (awakeSqlConfigurator == null) {
awakeSqlConfiguratorClassName = AWAKE_SQL_CONFIGURATOR_CLASS_NAME;
} else {
awakeSqlConfiguratorClassName = awakeSqlConfigurator.getClass()
.getName();
}
String awakeFileConfiguratorClassName = null;
if (awakeFileConfigurator == null) {
awakeFileConfiguratorClassName = AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
} else {
awakeFileConfiguratorClassName = awakeFileConfigurator
.getClass().getName();
}
String awakeFileActionManagerClassName = null;
if (awakeFileActionManager == null) {
awakeFileActionManagerClassName = AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
} else {
awakeFileActionManagerClassName = awakeFileActionManager
.getClass().getName();
}
out.println("");
out.println("");
out.println("" + Version.getVersion()
+ "");
out.println("
");
out.println("
");
out.println(servletName
+ " Servlet Configuration");
out.println("");
out.println("
");
out.println("");
out.println("");
out.println(" Configurator Parameter ");
out.println(" Configurator Value ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME
+ " ");
out.println(" " + awakeCommonsConfiguratorClassName + " ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_SQL_CONFIGURATOR_CLASS_NAME + " ");
out.println(" " + awakeSqlConfiguratorClassName + " ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_FILE_CONFIGURATOR_CLASS_NAME + " ");
out.println(" " + awakeFileConfiguratorClassName + " ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_FILE_ACTION_MANAGER_CLASS_NAME
+ " ");
out.println(" " + awakeFileActionManagerClassName + " ");
out.println(" ");
out.println("
");
out.println("
");
out.println("");
out.println("");
out.println(" Awake SQL Configuration Status ");
out.println(" ");
out.println("");
out.println(" " + status + " ");
out.println(" ");
out.println("
");
out.println("");
} catch (Exception e) {
e.printStackTrace(out);
}
}
/**
* debug
*/
public static void debug(String s) {
if (DEBUG) {
try {
AwakeServerLogger.log(s);
} catch (Exception e1) {
System.out.println(s);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy