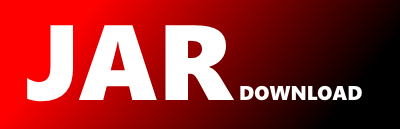
src-main.org.awakefw.sql.servlet.connection.ConnectionInfoUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet.connection;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Array;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Properties;
import javax.servlet.http.HttpServletRequest;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.util.TransferStatus;
import org.awakefw.file.util.parms.Parameter;
import org.awakefw.sql.api.util.SqlUtil;
import org.awakefw.sql.json.ObjectArrayTransport;
import org.awakefw.sql.json.PropertiesTransportGson;
import org.awakefw.sql.servlet.sql.oracle.OracleArrayBuilder;
import org.awakefw.sql.util.ConnectionParms;
import org.awakefw.sql.util.SqlAction;
import org.awakefw.sql.util.SqlReturnCode;
/**
* @author Nicolas de Pomereu
*
*/
public class ConnectionInfoUtil {
private static boolean DEBUG = AwakeDebug.isSet(ConnectionInfoUtil.class);
/**
*
*/
protected ConnectionInfoUtil() {
}
/**
*
* @param request
* @param awakeCommonsConfigurator
* @param out
* @param action
*/
public static void connectionInfoExecute(HttpServletRequest request,
AwakeCommonsConfigurator awakeCommonsConfigurator, PrintWriter out,
String action) throws IOException, SQLException, IllegalArgumentException {
String connectionId = request
.getParameter(ConnectionParms.CONNECTION_ID);
String username = request.getParameter(Parameter.USERNAME);
ConnectionStore connectionStore = new ConnectionStore(username,
connectionId);
Connection connection = connectionStore.get();
debug("");
debug("connectionInfoExecute");
debug("action :" + action + ":");
debug("connectionId :" + connectionId + ":");
debug("username :" + username+ ":");
debug("connection :" + connection + ":");
if (connection == null) {
out.println(TransferStatus.SEND_OK);
out.println(SqlReturnCode.SESSION_INVALIDATED);
return;
}
if (action.equals(SqlAction.ACTION_SQL_IS_VALID)) {
String timeoutStr = request
.getParameter(ConnectionParms.TIMEOUT);
int timeout = Integer.parseInt(timeoutStr);
boolean isValid = connection.isValid(timeout);
out.println(TransferStatus.SEND_OK);
out.println(isValid + "");
return;
}
else if (action.equals(SqlAction.ACTION_SQL_SET_CLIENT_INFO_NAME)) {
String name = request
.getParameter(ConnectionParms.NAME);
String value = request
.getParameter(ConnectionParms.VALUE);
name = HtmlConverter.fromHtml(name);
value = HtmlConverter.fromHtml(value);
connection.setClientInfo(name, value);
out.println(TransferStatus.SEND_OK);
return;
}
else if (action.equals(SqlAction.ACTION_SQL_SET_CLIENT_INFO_PROP)) {
String jsonString = request
.getParameter(ConnectionParms.PROPERTIES);
jsonString = HtmlConverter.fromHtml(jsonString);
Properties prop = PropertiesTransportGson.fromJson(jsonString);
connection.setClientInfo(prop);
out.println(TransferStatus.SEND_OK);
return;
}
else if (action.equals(SqlAction.ACTION_SQL_GET_CLIENT_INFO)) {
Properties prop = connection.getClientInfo();
String jsonString = PropertiesTransportGson.toJson(prop);
jsonString = HtmlConverter.toHtml(jsonString);
out.println(TransferStatus.SEND_OK);
out.println(jsonString);
return;
}
else if (action.equals(SqlAction.ACTION_SQL_GET_CLIENT_INFO_NAME)) {
String name = request
.getParameter(ConnectionParms.NAME);
String value = connection.getClientInfo(name);
value = HtmlConverter.toHtml(value);
out.println(TransferStatus.SEND_OK);
out.println(value);
return;
}
else if (action.equals(SqlAction.ACTION_SQL_CREATE_ARRAY_OF)) {
String typeName = request
.getParameter(ConnectionParms.TYPENAME);
String jsonString = request
.getParameter(ConnectionParms.ELEMENTS);
jsonString = HtmlConverter.fromHtml(jsonString);
Object[] elements = ObjectArrayTransport.fromJson(jsonString);
if (new SqlUtil(connection).isOracle()) {
// Specific code for Oracle
Array array = OracleArrayBuilder.create(connection, typeName, elements);
connectionStore.put(array);
out.println(TransferStatus.SEND_OK);
out.println("" + array.hashCode());
return;
}
else {
Array array = connection.createArrayOf(typeName, elements);
connectionStore.put(array);
out.println(TransferStatus.SEND_OK);
out.println("" + array.hashCode());
return;
}
}
else {
throw new IllegalArgumentException(
"Invalid Sql Action for connectionInfoExecute(): " + action);
}
}
/**
* Method called by children Servlet for debug purpose println is done only
* if class name name is in awake-debug.ini
*/
public static void debug(String s) {
if (DEBUG) {
AwakeServerLogger.log(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy