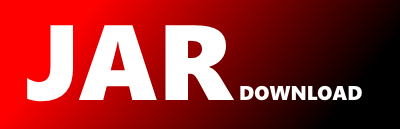
src-main.org.awakefw.sql.transport.SavepointHttp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.transport;
import java.sql.SQLException;
import java.sql.Savepoint;
import org.apache.commons.lang3.StringUtils;
/**
* @author Nicolas de Pomereu
*
*/
public class SavepointHttp implements Savepoint {
private int id = 0;
private String name = null;
/**
* Constructor
* @param id the Savepoint Id
* @param name the Savepoint Name
*/
public SavepointHttp(int id, String name) {
super();
this.id = id;
this.name = name;
}
/**
* Retrieves the generated ID for the savepoint that this
* Savepoint
object represents.
* @return the numeric ID of this savepoint
* @exception SQLException if this is a named savepoint
* @since 1.4
*/
@Override
public int getSavepointId() throws SQLException {
return id;
}
/**
* Retrieves the name of the savepoint that this Savepoint
* object represents.
* @return the name of this savepoint
* @exception SQLException if this is an un-named savepoint
* @since 1.4
*/
@Override
public String getSavepointName() throws SQLException {
return name;
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return "[id=" + id + ", name=" + name + "]";
}
/**
* Builds a Savepoint from a String
* @param savepointStrthe String containing a SavepointHttp.toString()
* @return a SavepointHttp
*/
public static Savepoint buildFromString(String savepointStr) {
if (savepointStr == null) {
throw new IllegalArgumentException("Savepoint can not be null!");
}
if (! savepointStr.contains("[id=") || ! savepointStr.contains(", name") || ! savepointStr.endsWith("]")) {
throw new IllegalArgumentException("Savepoint String is not conform to pattern [id=theId, name=theName]: " + savepointStr);
}
String idStr = StringUtils.substringBetween(savepointStr, "[id=" , ", name=");
if (idStr == null) {
throw new IllegalArgumentException("id as String can not be null!");
}
idStr = idStr.trim();
int id = Integer.parseInt(idStr);
String name = StringUtils.substringBetween(savepointStr, ", name=", "]");
if (name == null) {
throw new IllegalArgumentException("name can not be null!");
}
name = name.trim();
SavepointHttp savepointHttp = new SavepointHttp(id, name);
return savepointHttp;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy