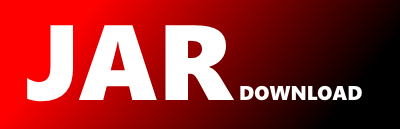
src-main.org.awakefw.sql.util.MapCopier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.util;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TreeMap;
/**
*
* Utility class to build a defensive copy of a Map, backed by a Hashmap,
* Hashtable , LinkedHashMap or TreeMap.
*
* @author Nicolas de Pomereu
*
*/
public class MapCopier {
/**
* Protected constructor
*/
public MapCopier() {
}
/**
* Copy a Map to another Map
*
* @param map
* the map to copy
* @return the copied map
*/
public Map copy(Map map) {
Map mapCopy = null;
if (map instanceof HashMap) {
mapCopy = new HashMap(map);
} else if (map instanceof Hashtable) {
mapCopy = new Hashtable(map);
} else if (map instanceof LinkedHashMap) {
mapCopy = new LinkedHashMap(map);
} else if (map instanceof TreeMap) {
mapCopy = new TreeMap(map);
} else {
throw new IllegalArgumentException(
"copy implementation not supported for Map class: "
+ map.getClass());
}
/*
* Set keys = map.keySet();
*
* for (Iterator iterator = keys.iterator(); iterator.hasNext();) { K
* key = (K) iterator.next(); V value = map.get(key);
*
* mapCopy.put(key, value); }
*/
return mapCopy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy