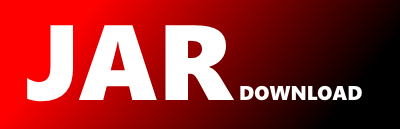
src-main.org.awakefw.sql.util.crypto.CallableStatementHolderCrypto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.util.crypto;
import java.sql.SQLException;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.crypto.Cipher;
import org.apache.commons.lang3.StringUtils;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.util.convert.Pbe;
import org.awakefw.sql.json.no_obfuscation.CallableStatementHolder;
/**
* @author Alexandre Becquereau
*/
public class CallableStatementHolderCrypto {
/** The callable statement holder to crypt/decrypt */
private CallableStatementHolder callableStatementHolder = null;
/** The encryption password */
private char[] password = null;
/**
* Constructor
*
* @param callableStatementHolder
* the callable statement holder to crypt/decrypt
* @param password
* The encryption password
*/
public CallableStatementHolderCrypto(CallableStatementHolder callableStatementHolder,
char[] password) {
this.callableStatementHolder = callableStatementHolder;
this.password = password;
}
/**
* Encrypt the CallableStatementHolder
*
* @param encryptParameters
* if true, encrypt the String parameters
* @return the encrypted the CallableStatementHolder
* @throws Exception
*/
public CallableStatementHolder encrypt(boolean encryptParameters) throws Exception {
callableStatementHolder.setParamatersEncrypted(encryptParameters);
cipher(Cipher.ENCRYPT_MODE);
return callableStatementHolder;
}
/**
* Decrypt the CallableStatementHolder
*
* @return the decrypted CallableStatementHolder
* @throws Exception
*/
public CallableStatementHolder decrypt() throws Exception {
cipher(Cipher.DECRYPT_MODE);
return callableStatementHolder;
}
/**
* Encrypt the CallableStatementHolder
*
* @param mode
* @throws Exception
*/
private void cipher(int mode) throws Exception {
if (mode != Cipher.ENCRYPT_MODE && mode != Cipher.DECRYPT_MODE) {
throw new IllegalArgumentException("Invalid Cipher Mode: " + mode);
}
String sqlOrder = this.callableStatementHolder.getSqlOrder();
// do nothing on empty sql order
if (sqlOrder == null || sqlOrder.isEmpty()) {
return;
}
Pbe pbe = new Pbe();
if (mode == Cipher.ENCRYPT_MODE) {
// Need to re-Html Convert sqlOrder
sqlOrder = HtmlConverter.toHtml(sqlOrder);
sqlOrder = pbe.encryptToHexa(sqlOrder, password);
this.callableStatementHolder.setSqlOrder(Pbe.AWAKE_ENCRYPTED + sqlOrder);
} else {
if (sqlOrder.startsWith(Pbe.AWAKE_ENCRYPTED)) {
sqlOrder = StringUtils.substringAfter(sqlOrder,
Pbe.AWAKE_ENCRYPTED);
sqlOrder = pbe.decryptFromHexa(sqlOrder, password);
this.callableStatementHolder.setSqlOrder(sqlOrder);
} else {
return; // do nothing if it was not encrypted at start
}
}
if (callableStatementHolder.isParamatersEncrypted()) {
Map parmValues = this.callableStatementHolder
.getParameterStringValues();
Set keys = parmValues.keySet();
for (Iterator iterator = keys.iterator(); iterator
.hasNext();) {
Integer key = iterator.next();
String value = parmValues.get(key);
if (value != null) {
if (mode == Cipher.ENCRYPT_MODE) {
value = pbe.encryptToHexa(value, password);
} else {
value = pbe.decryptFromHexa(value, password);
}
// This will automatically refresh the inside value
parmValues.put(key, value);
}
}
}
}
/**
* Decrypt a StatementHolder List
*
* @param statementHolderList
* the StatementHolder List to decrypt
* @param password
* the decryption password
* @throws SQLException
*/
public static void decrypt(List statementHolderList,
char[] password) throws SQLException {
try {
for (int i = 0; i < statementHolderList.size(); i++) {
CallableStatementHolder statementHolder = statementHolderList.get(i);
CallableStatementHolderCrypto statementHolderCrypto = new CallableStatementHolderCrypto(
statementHolder, password);
statementHolder = statementHolderCrypto.decrypt();
}
} catch (Exception e) {
throw new SQLException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy