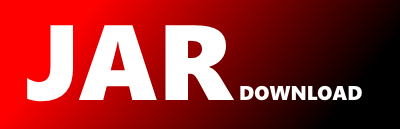
src-main.org.awakefw.sql.util.reflection.ReflectionCaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.util.reflection;
import java.sql.SQLException;
import org.awakefw.sql.api.server.AwakeSqlConfigurator;
/**
* @author Nicolas de Pomereu
*
* A class that allows to call AwakeCommonsConfigurator & AwakeSqlConfigurator methods per reflection using Invoker.
*/
public class ReflectionCaller {
/**
* Protected constructor
*/
protected ReflectionCaller() {
}
// KEEP A REFLECTION MODEL
// /**
// * Return the result of computeAuthToken(String username) method of AwakeCommonsConfigurator
// *
// * @param AwakeCommonsConfigurator the AwakeCommonsConfigurator instance
// * @return the list of banned IPs else null
// *
// * @throws SQLException if any Exception occurs, it is wrapped into an SQLException
// */
// public static char [] getEncryptionPassword(AwakeCommonsConfigurator awakeCommonsConfigurator) throws SQLException
// {
// String methodName = new Object() {
// }.getClass().getEnclosingMethod().getName();
//
// if (! Invoker.existsMethod(org.awakefw.commons.api.server.AwakeCommonsConfigurator.class.getName(), methodName)) {
// return null;
// }
//
// Object result = null;
//
// try {
// result = Invoker.getMethodResult(awakeCommonsConfigurator, methodName);
// } catch (Exception e) {
// throw new SQLException(e);
// }
//
// if (result == null) {
// return null;
// }
// else {
// return (char[]) result;
// }
// }
/**
* Return the result of getDelayBeforeNextLogin method of AwakeSqlConfigurator
*
* @param awakeSqlConfigurator the AwakeSqlConfigurator instance
* @return the maximum of attempts before login delay
*
* @throws SQLException if any Exception occurs, it is wrapped into an SQLException
*/
public static int getMaxLoginAttemptsBeforeDelay(AwakeSqlConfigurator awakeSqlConfigurator) throws SQLException
{
String methodName = new Object() {
}.getClass().getEnclosingMethod().getName();
if (! Invoker.existsMethod(org.awakefw.sql.api.server.AwakeSqlConfigurator.class.getName(), methodName)) {
return 0;
}
Object result = null;
try {
result = Invoker.getMethodResult(awakeSqlConfigurator, methodName);
} catch (Exception e) {
throw new SQLException(e);
}
if (result == null) {
return 0;
}
else {
return (Integer) result;
}
}
/**
* Return the result of getDelayBeforeNextLogin method of AwakeSqlConfigurator
*
* @param awakeSqlConfigurator the AwakeSqlConfigurator instance
* @return the delay in seconds before the next authorized login
*
* @throws SQLException if any Exception occurs, it is wrapped into an SQLException
*/
public static int getDelayBeforeNextLogin(AwakeSqlConfigurator awakeSqlConfigurator) throws SQLException
{
String methodName = new Object() {
}.getClass().getEnclosingMethod().getName();
if (! Invoker.existsMethod(org.awakefw.sql.api.server.AwakeSqlConfigurator.class.getName(), methodName)) {
return 0;
}
Object result = null;
try {
result = Invoker.getMethodResult(awakeSqlConfigurator, methodName);
} catch (Exception e) {
throw new SQLException(e);
}
if (result == null) {
return 0;
}
else {
return (Integer) result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy