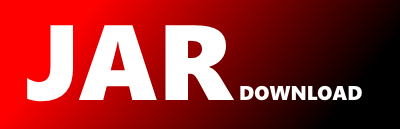
org.axonframework.boot.autoconfig.AxonAutoConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axon-spring-boot-autoconfigure Show documentation
Show all versions of axon-spring-boot-autoconfigure Show documentation
Module providing support for auto-configuration of Axon Framework through Spring Boot.
/*
* Copyright (c) 2010-2017. Axon Framework
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.axonframework.boot.autoconfig;
import org.axonframework.boot.DistributedCommandBusProperties;
import org.axonframework.boot.EventProcessorProperties;
import org.axonframework.boot.util.ConditionalOnMissingQualifiedBean;
import org.axonframework.commandhandling.CommandBus;
import org.axonframework.commandhandling.SimpleCommandBus;
import org.axonframework.commandhandling.distributed.DistributedCommandBus;
import org.axonframework.commandhandling.gateway.CommandGateway;
import org.axonframework.commandhandling.gateway.DefaultCommandGateway;
import org.axonframework.common.transaction.TransactionManager;
import org.axonframework.config.Configuration;
import org.axonframework.config.EventHandlingConfiguration;
import org.axonframework.eventhandling.*;
import org.axonframework.eventhandling.async.SequencingPolicy;
import org.axonframework.eventhandling.async.SequentialPerAggregatePolicy;
import org.axonframework.eventsourcing.eventstore.EmbeddedEventStore;
import org.axonframework.eventsourcing.eventstore.EventStorageEngine;
import org.axonframework.eventsourcing.eventstore.EventStore;
import org.axonframework.messaging.StreamableMessageSource;
import org.axonframework.messaging.SubscribableMessageSource;
import org.axonframework.messaging.correlation.CorrelationDataProvider;
import org.axonframework.messaging.correlation.MessageOriginProvider;
import org.axonframework.messaging.interceptors.CorrelationDataInterceptor;
import org.axonframework.queryhandling.QueryBus;
import org.axonframework.queryhandling.QueryInvocationErrorHandler;
import org.axonframework.queryhandling.SimpleQueryBus;
import org.axonframework.serialization.Serializer;
import org.axonframework.serialization.xml.XStreamSerializer;
import org.axonframework.spring.config.AxonConfiguration;
import org.springframework.beans.factory.BeanClassLoaderAware;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.autoconfigure.AutoConfigureAfter;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
import java.util.function.Function;
import org.springframework.context.annotation.Primary;
/**
* @author Allard Buijze
* @author Josh Long
*/
@org.springframework.context.annotation.Configuration
@AutoConfigureAfter(name = {"org.springframework.boot.autoconfigure.orm.jpa.HibernateJpaAutoConfiguration",
"org.axonframework.boot.autoconfig.JpaAutoConfiguration"})
@EnableConfigurationProperties(value = {
EventProcessorProperties.class,
DistributedCommandBusProperties.class
})
public class AxonAutoConfiguration implements BeanClassLoaderAware {
private final EventProcessorProperties eventProcessorProperties;
private ClassLoader beanClassLoader;
public AxonAutoConfiguration(EventProcessorProperties eventProcessorProperties) {
this.eventProcessorProperties = eventProcessorProperties;
}
@Bean
@Primary
@ConditionalOnMissingQualifiedBean(beanClass = Serializer.class, qualifier = "!eventSerializer")
public XStreamSerializer serializer() {
XStreamSerializer xStreamSerializer = new XStreamSerializer();
xStreamSerializer.getXStream().setClassLoader(beanClassLoader);
return xStreamSerializer;
}
@Bean
@ConditionalOnMissingBean
public CorrelationDataProvider messageOriginProvider() {
return new MessageOriginProvider();
}
@Qualifier("eventStore")
@Bean(name = "eventBus")
@ConditionalOnMissingBean(EventBus.class)
@ConditionalOnBean(EventStorageEngine.class)
public EmbeddedEventStore eventStore(EventStorageEngine storageEngine, AxonConfiguration configuration) {
return new EmbeddedEventStore(storageEngine, configuration.messageMonitor(EventStore.class, "eventStore"));
}
@ConditionalOnMissingBean
@Bean
public CommandGateway commandGateway(CommandBus commandBus) {
return new DefaultCommandGateway(commandBus);
}
@Bean
@ConditionalOnMissingBean({EventStorageEngine.class, EventBus.class})
public SimpleEventBus eventBus(AxonConfiguration configuration) {
return new SimpleEventBus(Integer.MAX_VALUE, configuration.messageMonitor(EventStore.class, "eventStore"));
}
@Autowired
public void configureEventHandling(EventHandlingConfiguration eventHandlingConfiguration,
ApplicationContext applicationContext) {
eventProcessorProperties.getProcessors().forEach((k, v) -> {
if (v.getMode() == EventProcessorProperties.Mode.TRACKING) {
TrackingEventProcessorConfiguration config = TrackingEventProcessorConfiguration
.forParallelProcessing(v.getThreadCount())
.andBatchSize(v.getBatchSize())
.andInitialSegmentsCount(v.getInitialSegmentCount());
Function>> sequencingPolicy = resolveSequencingPolicy(applicationContext, v);
Function>> messageSource = resolveMessageSource(applicationContext, v);
eventHandlingConfiguration.registerTrackingProcessor(k, messageSource, c -> config, sequencingPolicy);
} else {
if (v.getSource() == null) {
eventHandlingConfiguration.registerSubscribingEventProcessor(k);
} else {
eventHandlingConfiguration.registerSubscribingEventProcessor(k, c -> applicationContext
.getBean(v.getSource(), SubscribableMessageSource.class));
}
}
});
}
private Function>> resolveMessageSource(ApplicationContext applicationContext, EventProcessorProperties.ProcessorSettings v) {
Function>> messageSource;
if (v.getSource() == null) {
messageSource = Configuration::eventStore;
} else {
messageSource = c -> applicationContext.getBean(v.getSource(), StreamableMessageSource.class);
}
return messageSource;
}
private Function>> resolveSequencingPolicy(ApplicationContext applicationContext, EventProcessorProperties.ProcessorSettings v) {
Function>> sequencingPolicy;
if (v.getSequencingPolicy() != null) {
sequencingPolicy = c -> applicationContext.getBean(v.getSequencingPolicy(), SequencingPolicy.class);
} else {
sequencingPolicy = c -> new SequentialPerAggregatePolicy();
}
return sequencingPolicy;
}
@ConditionalOnMissingBean(ignored = {DistributedCommandBus.class}, value = CommandBus.class)
@Qualifier("localSegment")
@Bean
public SimpleCommandBus commandBus(TransactionManager txManager, AxonConfiguration axonConfiguration) {
SimpleCommandBus commandBus = new SimpleCommandBus(txManager, axonConfiguration.messageMonitor(CommandBus.class, "commandBus"));
commandBus.registerHandlerInterceptor(new CorrelationDataInterceptor<>(axonConfiguration.correlationDataProviders()));
return commandBus;
}
@ConditionalOnMissingBean(value = {QueryBus.class, QueryInvocationErrorHandler.class})
@Qualifier("localSegment")
@Bean
public SimpleQueryBus queryBus(AxonConfiguration axonConfiguration, TransactionManager transactionManager) {
return new SimpleQueryBus(axonConfiguration.messageMonitor(QueryBus.class, "queryBus"),
transactionManager,
axonConfiguration.getComponent(QueryInvocationErrorHandler.class));
}
@ConditionalOnBean(QueryInvocationErrorHandler.class)
@ConditionalOnMissingBean(value = QueryBus.class)
@Qualifier("localSegment")
@Bean
public SimpleQueryBus queryBus(AxonConfiguration axonConfiguration, TransactionManager transactionManager,
QueryInvocationErrorHandler eh) {
return new SimpleQueryBus(axonConfiguration.messageMonitor(QueryBus.class, "queryBus"),
transactionManager,
eh);
}
@Override
public void setBeanClassLoader(ClassLoader classLoader) {
this.beanClassLoader = classLoader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy