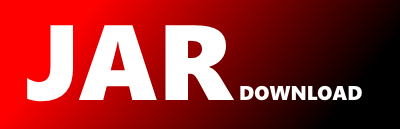
org.aya.util.Arg Maven / Gradle / Ivy
// Copyright (c) 2020-2023 Tesla (Yinsen) Zhang.
// Use of this source code is governed by the MIT license that can be found in the LICENSE.md file.
package org.aya.util;
import org.jetbrains.annotations.NotNull;
import java.util.function.Function;
import java.util.function.UnaryOperator;
/**
* @param the type of expressions.
* In Aya, it is either core term, core pattern, concrete term, or concrete pattern.
*/
public record Arg(@Override @NotNull T term, @Override boolean explicit) implements BinOpElem {
public static @NotNull Arg ofExplicitly(@NotNull T term) {
return new Arg<>(term, true);
}
public static @NotNull Arg ofImplicitly(@NotNull T term) {
return new Arg<>(term, false);
}
public @NotNull Arg implicitify() {
return new Arg<>(term, false);
}
public @NotNull Arg map(@NotNull Function mapper) {
return new Arg<>(mapper.apply(term), explicit);
}
public @NotNull Arg update(@NotNull T term) {
return term == term() ? this : new Arg<>(term, explicit);
}
public @NotNull Arg descent(@NotNull UnaryOperator<@NotNull T> f) {
return update(f.apply(term));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy