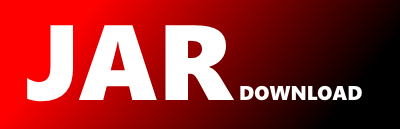
org.babyfish.jimmer.client.runtime.impl.MetadataImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jimmer-client Show documentation
Show all versions of jimmer-client Show documentation
A revolutionary ORM framework for both java and kotlin
package org.babyfish.jimmer.client.runtime.impl;
import org.babyfish.jimmer.client.runtime.*;
import java.util.*;
public class MetadataImpl implements Metadata {
private final boolean isGenericSupported;
private final Map> pathMap;
private final List services;
private final List fetchedTypes;
private final List dynamicTypes;
private final List staticTypes;
private final List enumTypes;
private final Map, Type> typeMap;
public MetadataImpl(
boolean isGenericSupported,
List services,
List fetchedTypes,
List dynamicTypes,
List staticTypes,
List enumTypes
) {
this.isGenericSupported = isGenericSupported;
this.pathMap = getPathMap(services);
this.services = services;
this.fetchedTypes = fetchedTypes;
this.dynamicTypes = dynamicTypes;
this.staticTypes = staticTypes;
this.enumTypes = enumTypes;
Map, Type> typeMap = new HashMap();
for (ObjectType fetchedType : fetchedTypes) {
typeMap.put(fetchedType.getJavaType(), fetchedType);
}
for (ObjectType dynamicType : dynamicTypes) {
typeMap.put(dynamicType.getJavaType(), dynamicType);
}
for (ObjectType staticType : staticTypes) {
typeMap.put(staticType.getJavaType(), staticType);
}
for (EnumType enumType : enumTypes) {
typeMap.put(enumType.getJavaType(), enumType);
}
this.typeMap = typeMap;
}
private static Map> getPathMap(List services) {
Map> map = new TreeMap<>();
for (Service service : services) {
for (Operation operation : service.getOperations()) {
Map subMap =
map.computeIfAbsent(operation.getUri(), it ->new TreeMap<>());
for (Operation.HttpMethod method : operation.getHttpMethods()) {
Operation conflictOperation = subMap.put(method, operation);
if (conflictOperation != null) {
throw new IllegalApiException(
"Conflict HTTP endpoint \"" +
method +
":" +
operation.getUri() +
"\" which is shared by \"" +
conflictOperation.getJavaMethod() +
"\" and \"" +
operation.getJavaMethod() +
"\""
);
}
}
}
}
Map> pathMap = new TreeMap<>();
for (Map.Entry> e : map.entrySet()) {
pathMap.put(e.getKey(), Collections.unmodifiableList(new ArrayList<>(e.getValue().values())));
}
return Collections.unmodifiableMap(pathMap);
}
@Override
public boolean isGenericSupported() {
return isGenericSupported;
}
@Override
public Map> getPathMap() {
return pathMap;
}
@Override
public List getServices() {
return services;
}
@Override
public List getFetchedTypes() {
return fetchedTypes;
}
@Override
public List getDynamicTypes() {
return dynamicTypes;
}
@Override
public List getStaticTypes() {
return staticTypes;
}
@Override
public List getEnumTypes() {
return enumTypes;
}
@Override
public Type getType(Class> type) {
return typeMap.get(type);
}
@Override
public String toString() {
return "MetadataImpl{" +
"services=" + services +
", fetchedTypes=" + fetchedTypes +
", dynamicTypes=" + dynamicTypes +
", staticTypes=" + staticTypes +
", enumTypes=" + enumTypes +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy