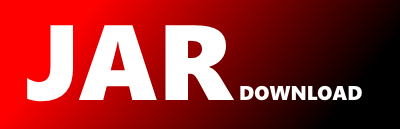
org.babyfish.jimmer.spring.repo.JavaRepository Maven / Gradle / Ivy
package org.babyfish.jimmer.spring.repo;
import org.babyfish.jimmer.Input;
import org.babyfish.jimmer.Page;
import org.babyfish.jimmer.Slice;
import org.babyfish.jimmer.View;
import org.babyfish.jimmer.meta.TypedProp;
import org.babyfish.jimmer.sql.ast.mutation.*;
import org.babyfish.jimmer.sql.fetcher.Fetcher;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* In earlier versions of Jimmer, type {@link org.babyfish.jimmer.spring.repository.JRepository}
* was used to support spring data style repository support.
*
* However, based on user feedback, this interface was rarely used. The root causes are:
*
* - Unlike JPA and MyBatis, which have lifecycle management objects like EntityManager/Session,
* Jimmer itself is already designed with a stateless API.
* Therefore, the stateless abstraction of spring dData style repository is meaningless for Jimmer.
* - Jimmer itself emphasizes type safety and strives to detect problems at compile-time.
* spring data's approach based on conventional method names and {@code @Query} annotations
* would lead to problems only being found at runtime (How Intellij helps certain solutions
* cheat is not discussed here), which goes against Jimmer's design philosophy.
*
* Therefore, developer can simply write a class and annotate it with
* {@link org.springframework.data.repository.Repository}. At this point, users can choose to implement this interface or extends class
* {@link org.babyfish.jimmer.spring.repo.support.AbstractJavaRepository}. Note, that this is optional, not mandatory.
*
* @param The entity type
* @param The entity id type
*/
public interface JavaRepository {
@Nullable default E findById(ID id) {
return findById(id, (Fetcher) null);
}
@Nullable E findById(ID id, @Nullable Fetcher fetcher);
@Nullable > V findById(ID id, Class viewType);
@NotNull default List findByIds(Collection ids) {
return findByIds(ids, (Fetcher) null);
}
@NotNull List findByIds(Collection ids, @Nullable Fetcher fetcher);
@NotNull > List findByIds(Collection ids, Class viewType);
@NotNull default Map findMapByIds(Collection ids) {
return findMapByIds(ids, (Fetcher) null);
}
@NotNull Map findMapByIds(Collection ids, Fetcher fetcher);
@NotNull > Map findMapByIds(Collection ids, Class viewType);
@NotNull default List findAll(TypedProp.Scalar, ?> ... sortedProps) {
return findAll((Fetcher) null, sortedProps);
}
@NotNull List findAll(@Nullable Fetcher fetcher, TypedProp.Scalar, ?> ... sortedProps);
@NotNull > List findAll(Class viewType, TypedProp.Scalar, ?> ... sortedProps);
@NotNull
default Page findPage(PageParam pageParam, TypedProp.Scalar, ?> ... sortedProps) {
return findPage(pageParam, (Fetcher) null, sortedProps);
}
@NotNull Page findPage(PageParam pageParam, @Nullable Fetcher fetcher, TypedProp.Scalar, ?> ... sortedProps);
@NotNull > Page findPage(
PageParam pageParam,
Class viewType,
TypedProp.Scalar, ?> ... sortedProps
);
@NotNull
default Slice findSlice(int limit, int offset, TypedProp.Scalar, ?> ... sortedProps) {
return findSlice(limit, offset, (Fetcher) null, sortedProps);
}
@NotNull Slice findSlice(int limit, int offset, @Nullable Fetcher fetcher, TypedProp.Scalar, ?> ... sortedProps);
@NotNull > Slice findSlice(
int limit,
int offset,
Class viewType,
TypedProp.Scalar, ?> ... sortedProps
);
default SimpleSaveResult save(E entity) {
return save(entity, SaveMode.UPSERT, AssociatedSaveMode.REPLACE);
}
default SimpleSaveResult save(E entity, SaveMode mode) {
return save(entity, mode, AssociatedSaveMode.REPLACE);
}
default SimpleSaveResult save(E entity, AssociatedSaveMode associatedMode) {
return save(entity, SaveMode.UPSERT, associatedMode);
}
SimpleSaveResult save(E entity, SaveMode mode, AssociatedSaveMode associatedMode);
default SimpleSaveResult insert(E entity) {
return save(entity, SaveMode.INSERT_ONLY, AssociatedSaveMode.APPEND);
}
default SimpleSaveResult insert(E entity, AssociatedSaveMode associatedSaveMode) {
return save(entity, SaveMode.INSERT_ONLY, associatedSaveMode);
}
default SimpleSaveResult insert(Input input) {
return save(input, SaveMode.INSERT_ONLY, AssociatedSaveMode.APPEND);
}
default SimpleSaveResult insert(Input input, AssociatedSaveMode associatedSaveMode) {
return save(input, SaveMode.INSERT_ONLY, associatedSaveMode);
}
default SimpleSaveResult insertIfAbsent(E entity) {
return save(entity, SaveMode.INSERT_IF_ABSENT, AssociatedSaveMode.APPEND_IF_ABSENT);
}
default SimpleSaveResult insertIfAbsent(E entity, AssociatedSaveMode associatedSaveMode) {
return save(entity, SaveMode.INSERT_IF_ABSENT, associatedSaveMode);
}
default SimpleSaveResult insertIfAbsent(Input input) {
return save(input, SaveMode.INSERT_IF_ABSENT, AssociatedSaveMode.APPEND_IF_ABSENT);
}
default SimpleSaveResult insertIfAbsent(Input input, AssociatedSaveMode associatedSaveMode) {
return save(input, SaveMode.INSERT_IF_ABSENT, associatedSaveMode);
}
default SimpleSaveResult update(E entity) {
return save(entity, SaveMode.UPDATE_ONLY, AssociatedSaveMode.UPDATE);
}
default SimpleSaveResult update(E entity, AssociatedSaveMode associatedSaveMode) {
return save(entity, SaveMode.UPDATE_ONLY, associatedSaveMode);
}
default SimpleSaveResult update(Input input) {
return save(input, SaveMode.UPDATE_ONLY, AssociatedSaveMode.UPDATE);
}
default SimpleSaveResult update(Input input, AssociatedSaveMode associatedSaveMode) {
return save(input, SaveMode.UPDATE_ONLY, associatedSaveMode);
}
default SimpleSaveResult merge(E entity) {
return save(entity, SaveMode.UPSERT, AssociatedSaveMode.MERGE);
}
default SimpleSaveResult merge(E entity, AssociatedSaveMode associatedSaveMode) {
return save(entity, SaveMode.UPSERT, associatedSaveMode);
}
default SimpleSaveResult merge(Input input) {
return save(input, SaveMode.UPSERT, AssociatedSaveMode.MERGE);
}
default SimpleSaveResult merge(Input input, AssociatedSaveMode associatedSaveMode) {
return save(input, SaveMode.UPSERT, associatedSaveMode);
}
default BatchSaveResult saveEntities(Collection entities) {
return saveEntities(entities, SaveMode.UPSERT, AssociatedSaveMode.REPLACE);
}
default BatchSaveResult saveEntities(Collection entities, SaveMode mode) {
return saveEntities(entities, mode, AssociatedSaveMode.REPLACE);
}
default BatchSaveResult saveEntities(Collection entities, AssociatedSaveMode associatedMode) {
return saveEntities(entities, SaveMode.UPSERT, associatedMode);
}
BatchSaveResult saveEntities(
Collection entities,
SaveMode rootSaveMode,
AssociatedSaveMode associatedSaveMode
);
default SimpleSaveResult save(Input input) {
return save(input, SaveMode.UPSERT, AssociatedSaveMode.REPLACE);
}
default SimpleSaveResult save(Input input, SaveMode mode) {
return save(input, mode, AssociatedSaveMode.REPLACE);
}
default SimpleSaveResult save(Input input, AssociatedSaveMode associatedMode) {
return save(input, SaveMode.UPSERT, associatedMode);
}
SimpleSaveResult save(
Input input,
SaveMode rootSaveMode,
AssociatedSaveMode associatedSaveMode
);
default BatchSaveResult saveInputs(Collection> inputs) {
return saveInputs(inputs, SaveMode.UPSERT, AssociatedSaveMode.REPLACE);
}
default BatchSaveResult saveInputs(Collection> inputs, SaveMode mode) {
return saveInputs(inputs, mode, AssociatedSaveMode.REPLACE);
}
default BatchSaveResult saveInputs(Collection> inputs, AssociatedSaveMode associatedMode) {
return saveInputs(inputs, SaveMode.UPSERT, associatedMode);
}
BatchSaveResult saveInputs(
Collection> inputs,
SaveMode rootSaveMode,
AssociatedSaveMode associatedSaveMode
);
default long deleteById(ID id) {
return deleteById(id, DeleteMode.AUTO);
}
long deleteById(ID id, DeleteMode deleteMode);
default long deleteByIds(Collection ids) {
return deleteByIds(ids, DeleteMode.AUTO);
}
long deleteByIds(Collection ids, DeleteMode deleteMode);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy