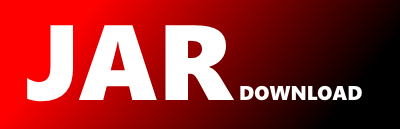
org.babyfish.jimmer.sql.kt.KSqlClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jimmer-sql-kotlin Show documentation
Show all versions of jimmer-sql-kotlin Show documentation
A revolutionary ORM framework for both java and kotlin
package org.babyfish.jimmer.sql.kt
import org.babyfish.jimmer.View
import org.babyfish.jimmer.lang.NewChain
import org.babyfish.jimmer.sql.JSqlClient
import org.babyfish.jimmer.sql.ast.mutation.DeleteMode
import org.babyfish.jimmer.sql.event.binlog.BinLog
import org.babyfish.jimmer.sql.exception.EmptyResultException
import org.babyfish.jimmer.sql.exception.TooManyResultsException
import org.babyfish.jimmer.sql.fetcher.DtoMetadata
import org.babyfish.jimmer.sql.fetcher.Fetcher
import org.babyfish.jimmer.sql.kt.ast.KExecutable
import org.babyfish.jimmer.sql.kt.ast.mutation.*
import org.babyfish.jimmer.sql.kt.ast.query.KConfigurableRootQuery
import org.babyfish.jimmer.sql.kt.ast.query.KMutableRootQuery
import org.babyfish.jimmer.sql.kt.cfg.KSqlClientDsl
import org.babyfish.jimmer.sql.kt.filter.KFilterDsl
import org.babyfish.jimmer.sql.kt.filter.KFilters
import org.babyfish.jimmer.sql.kt.impl.KSqlClientImpl
import org.babyfish.jimmer.sql.runtime.EntityManager
import org.babyfish.jimmer.sql.runtime.Executor
import org.babyfish.jimmer.sql.runtime.JSqlClientImplementor
import java.sql.Connection
import kotlin.reflect.KClass
import kotlin.reflect.KProperty1
interface KSqlClient : KSaver {
fun createQuery(
entityType: KClass,
block: KMutableRootQuery.() -> KConfigurableRootQuery
): KConfigurableRootQuery =
queries.forEntity(entityType, block)
fun createUpdate(
entityType: KClass,
block: KMutableUpdate.() -> Unit
): KExecutable
fun createDelete(
entityType: KClass,
block: KMutableDelete.() -> Unit
): KExecutable
fun executeQuery(
entityType: KClass,
limit: Int? = null,
con: Connection? = null,
block: KMutableRootQuery.() -> KConfigurableRootQuery
): List = queries
.forEntity(entityType, block)
.let { q ->
limit?.let { q.limit(it) } ?: q
}
.execute(con)
fun executeUpdate(
entityType: KClass,
con: Connection? = null,
block: KMutableUpdate.() -> Unit
): Int = createUpdate(entityType, block).execute(con)
fun executeDelete(
entityType: KClass,
con: Connection? = null,
block: KMutableDelete.() -> Unit
): Int = createDelete(entityType, block).execute(con)
val queries: KQueries
val entities: KEntities
val caches: KCaches
/**
* This property is equivalent to `getTriggers(false)`
*/
val triggers: KTriggers
/**
*
* -
* If trigger type is 'BINLOG_ONLY'
*
* - If `transaction` is true, throws exception
* - If `transaction` is false, return binlog trigger
*
*
* -
* If trigger type is 'TRANSACTION_ONLY', returns transaction trigger
* no matter what the `transaction` is
*
* -
* If trigger type is 'BOTH'
*
* - If `transaction` is true, return transaction trigger
* - If `transaction` is false, return binlog trigger
*
* Note that the objects returned by different parameters are independent of each other.
*
*
* @param transaction
* @return Trigger
*/
fun getTriggers(transaction: Boolean): KTriggers
val filters: KFilters
fun getAssociations(prop: KProperty1<*, *>): KAssociations
@NewChain
fun caches(block: KCacheDisableDsl.() -> Unit): KSqlClient
@NewChain
fun filters(block: KFilterDsl.() -> Unit): KSqlClient
@NewChain
fun disableSlaveConnectionManager(): KSqlClient
@NewChain
fun executor(executor: Executor?): KSqlClient
val entityManager: EntityManager
val binLog: BinLog
/**
* @param [T] Entity type or output DTO type
*/
fun findById(type: KClass, id: Any): T? =
entities.findById(type, id)
fun findById(fetcher: Fetcher, id: Any): E? =
entities.findById(fetcher, id)
/**
* @param [T] Entity type or output DTO type
*/
fun findByIds(type: KClass, ids: Iterable<*>): List =
entities.findByIds(type, ids)
fun findByIds(fetcher: Fetcher, ids: Iterable<*>): List =
entities.findByIds(fetcher, ids)
/**
* @param [T] Entity type or output DTO type
*/
fun findMapByIds(type: KClass, ids: Iterable): Map =
entities.findMapByIds(type, ids)
fun findMapByIds(fetcher: Fetcher, ids: Iterable): Map =
entities.findMapByIds(fetcher, ids)
/**
* @param [T] Entity type or output DTO type
*/
fun findOneById(type: KClass, id: Any): T =
entities.findOneById(type, id)
fun findOneById(fetcher: Fetcher, id: Any): E =
entities.findOneById(fetcher, id)
fun findAll(
fetcher: Fetcher,
limit: Int? = null,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit = {}
): List = executeQuery(fetcher.javaClass.kotlin, limit, con) {
block()
select(table.fetch(fetcher))
}
fun findOne(
fetcher: Fetcher,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit
): E = findAll(fetcher, 2, null, block).let {
when (it.size) {
0 -> throw EmptyResultException()
1 -> it[0]
else -> throw TooManyResultsException()
}
}
fun findOneOrNull(
fetcher: Fetcher,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit
): E? = findAll(fetcher, 2, con, block).let {
when (it.size) {
0 -> null
1 -> return it[0]
else -> throw TooManyResultsException()
}
}
fun > findAll(
viewType: KClass,
limit: Int? = null,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit = {}
): List {
val metadata = DtoMetadata.of(viewType.java)
return findAll(metadata.fetcher, limit, con, block).map(metadata.converter::apply)
}
fun > findOne(
viewType: KClass,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit
): V {
val metadata = DtoMetadata.of(viewType.java)
return findOne(metadata.fetcher, con, block).let(metadata.converter::apply)
}
fun > findOneOrNull(
viewType: KClass,
con: Connection? = null,
block: KMutableRootQuery.() -> Unit
): V? {
val metadata = DtoMetadata.of(viewType.java)
return findOneOrNull(metadata.fetcher, con, block)?.let(metadata.converter::apply)
}
override fun save(
entity: E,
con: Connection?,
block: (KSaveCommandDsl.() -> Unit)?
): KSimpleSaveResult =
entities.save(entity, con, block)
override fun saveEntities(
entities: Iterable,
con: Connection?,
block: (KSaveCommandDsl.() -> Unit)?
): KBatchSaveResult =
this.entities.saveEntities(entities, con, block)
fun deleteById(type: KClass, id: Any, mode: DeleteMode = DeleteMode.AUTO): KDeleteResult =
entities.delete(type, id) {
setMode(mode)
}
fun deleteById(type: KClass, id: Any, block: KDeleteCommandDsl.() -> Unit): KDeleteResult =
entities.delete(type, id, block = block)
fun deleteByIds(type: KClass, ids: Iterable<*>, mode: DeleteMode = DeleteMode.AUTO): KDeleteResult =
entities.deleteAll(type, ids) {
setMode(mode)
}
fun deleteByIds(type: KClass, ids: Iterable<*>, block: KDeleteCommandDsl.() -> Unit): KDeleteResult =
entities.deleteAll(type, ids, block = block)
val javaClient: JSqlClientImplementor
}
fun newKSqlClient(block: KSqlClientDsl.() -> Unit): KSqlClient {
val javaBuilder = JSqlClient.newBuilder()
val dsl = KSqlClientDsl(javaBuilder)
dsl.block()
return dsl.buildKSqlClient()
}
fun JSqlClient.toKSqlClient(): KSqlClient =
KSqlClientImpl(this as JSqlClientImplementor)
© 2015 - 2024 Weber Informatics LLC | Privacy Policy