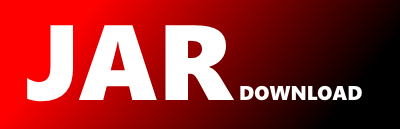
org.babyfish.jimmer.sql.kt.impl.KEntitiesImpl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jimmer-sql-kotlin Show documentation
Show all versions of jimmer-sql-kotlin Show documentation
A revolutionary ORM framework for both java and kotlin
package org.babyfish.jimmer.sql.kt.impl
import org.babyfish.jimmer.Input
import org.babyfish.jimmer.View
import org.babyfish.jimmer.meta.ImmutableType
import org.babyfish.jimmer.sql.Entities
import org.babyfish.jimmer.sql.ast.Selection
import org.babyfish.jimmer.sql.ast.impl.EntitiesImpl
import org.babyfish.jimmer.sql.ast.impl.query.FilterLevel
import org.babyfish.jimmer.sql.ast.impl.query.MutableRootQueryImpl
import org.babyfish.jimmer.sql.ast.impl.table.FetcherSelectionImpl
import org.babyfish.jimmer.sql.ast.impl.table.TableImplementor
import org.babyfish.jimmer.sql.ast.mutation.BatchEntitySaveCommand
import org.babyfish.jimmer.sql.ast.mutation.SimpleEntitySaveCommand
import org.babyfish.jimmer.sql.ast.table.Table
import org.babyfish.jimmer.sql.fetcher.Fetcher
import org.babyfish.jimmer.sql.fetcher.DtoMetadata
import org.babyfish.jimmer.sql.kt.KEntities
import org.babyfish.jimmer.sql.kt.ast.mutation.*
import org.babyfish.jimmer.sql.kt.ast.mutation.impl.*
import org.babyfish.jimmer.sql.kt.ast.query.KExample
import org.babyfish.jimmer.sql.kt.ast.query.SortDsl
import org.babyfish.jimmer.sql.runtime.ExecutionPurpose
import java.sql.Connection
import java.util.function.Function
import kotlin.reflect.KClass
import kotlin.reflect.full.isSubclassOf
internal class KEntitiesImpl(
private val javaEntities: Entities
): KEntities {
override fun forUpdate(): KEntities =
javaEntities.forUpdate().let {
if (javaEntities === it) {
this
} else {
KEntitiesImpl(it)
}
}
override fun forConnection(con: Connection?): KEntities =
javaEntities.forConnection(con).let {
if (javaEntities === it) {
this
} else {
KEntitiesImpl(it)
}
}
override fun findById(type: KClass, id: Any): E? =
javaEntities.findById(type.java, id)
override fun findOneById(type: KClass, id: Any): E =
javaEntities.findOneById(type.java, id)
override fun findById(fetcher: Fetcher, id: Any): E? =
javaEntities.findById(fetcher, id)
override fun findOneById(fetcher: Fetcher, id: Any): E =
javaEntities.findOneById(fetcher, id)
override fun findByIds(
type: KClass,
ids: Iterable<*>
): List =
javaEntities.findByIds(type.java, ids)
override fun findByIds(
fetcher: Fetcher,
ids: Iterable<*>
): List =
javaEntities.findByIds(fetcher, ids)
override fun findMapByIds(
type: KClass,
ids: Iterable
): Map =
javaEntities.findMapByIds(type.java, ids)
override fun findMapByIds(
fetcher: Fetcher,
ids: Iterable
): Map =
javaEntities.findMapByIds(fetcher, ids)
@Suppress("UNCHECKED_CAST")
override fun findAll(type: KClass): List =
if (type.isSubclassOf(View::class)) {
find(DtoMetadata.of(type.java as Class>), null, null) as List
} else {
find(ImmutableType.get(type.java), null, null, null)
}
override fun findAll(type: KClass, block: (SortDsl.() -> Unit)?): List =
if (type.isSubclassOf(View::class)) {
throw IllegalArgumentException("The argument cannot be view type, please call `findAllViews`")
} else {
find(ImmutableType.get(type.java), null, null, block)
}
@Suppress("UNCHECKED_CAST")
override fun > findAllViews(viewType: KClass, block: (SortDsl.() -> Unit)?): List =
find(DtoMetadata.of(viewType.java), null, block as (SortDsl<*>.() -> Unit)?)
override fun findAll(fetcher: Fetcher, block: (SortDsl.() -> Unit)?): List =
find(fetcher.immutableType, fetcher, null, block)
override fun findByExample(
example: KExample,
fetcher: Fetcher?,
block: (SortDsl.() -> Unit)?
): List =
find(example.type, fetcher, example, block)
@Suppress("UNCHECKED_CAST")
override fun > findByExample(
viewType: KClass,
example: KExample,
block: (SortDsl.() -> Unit)?
): List =
find(DtoMetadata.of(viewType.java), example, block as (SortDsl<*>.() -> Unit)?)
@Suppress("UNCHECKED_CAST")
private fun find(
type: ImmutableType,
fetcher: Fetcher?,
example: KExample?,
block: (SortDsl.() -> Unit)?
): List {
if (fetcher !== null && fetcher.immutableType !== type) {
throw IllegalArgumentException(
"The type \"${fetcher.immutableType}\" of fetcher does not match the query type \"$type\""
)
}
if (example !== null && example.type !== type) {
throw IllegalArgumentException(
"The type \"${example.type}\" of example does not match the query type \"$type\""
)
}
val entities = javaEntities as EntitiesImpl
val query = MutableRootQueryImpl>(entities.sqlClient, type, ExecutionPurpose.QUERY, FilterLevel.DEFAULT)
val table = query.tableImplementor as TableImplementor
query.where(example?.toPredicate(query.tableImplementor))
if (block !== null) {
val dsl = SortDsl()
dsl.block()
dsl.applyTo(query)
}
return query.select(
if (fetcher !== null) {
table.fetch(fetcher)
} else {
table
}
).execute(entities.con)
}
@Suppress("UNCHECKED_CAST")
private fun > find(
metadata: DtoMetadata<*, V>,
example: KExample<*>?,
block: (SortDsl<*>.() -> Unit)?
): List {
val fetcher = metadata.getFetcher()
val converter = metadata.getConverter() as Function<*, V>
val type = fetcher.immutableType
val entities = javaEntities as EntitiesImpl
val query = MutableRootQueryImpl>(entities.sqlClient, type, ExecutionPurpose.QUERY, FilterLevel.DEFAULT)
val table = query.tableImplementor
if (example !== null) {
query.where(example.toPredicate(table))
}
if (block !== null) {
val dsl = SortDsl()
dsl.block()
dsl.applyTo(query)
}
return query.select(
if (fetcher !== null) {
FetcherSelectionImpl(table, fetcher, converter)
} else {
table as Selection
}
).execute(entities.con)
}
@Suppress("UNCHECKED_CAST")
override fun save(
entity: E,
con: Connection?,
block: (KSaveCommandDsl.() -> Unit)?
): KSimpleSaveResult =
javaEntities
.saveCommand(entity)
.let {
if (block === null) {
it
} else {
val dsl = KSaveCommandDslImpl(it)
dsl.block()
dsl.javaCommand as SimpleEntitySaveCommand
}
}
.execute(con)
.let { KSimpleSaveResultImpl(it) }
override fun save(
input: Input,
con: Connection?,
block: (KSaveCommandDsl.() -> Unit)?
): KSimpleSaveResult =
save(input.toEntity(), con, block)
@Suppress("UNCHECKED_CAST")
override fun saveEntities(
entities: Iterable,
con: Connection?,
block: (KSaveCommandDsl.() -> Unit)?
): KBatchSaveResult =
javaEntities
.saveEntitiesCommand(entities)
.let {
if (block === null) {
it
} else {
val dsl = KSaveCommandDslImpl(it)
dsl.block()
dsl.javaCommand as BatchEntitySaveCommand
}
}
.execute(con)
.let { KBatchSaveResultImpl(it) }
override fun delete(
type: KClass<*>,
id: Any,
con: Connection?,
block: (KDeleteCommandDsl.() -> Unit)?
): KDeleteResult =
javaEntities
.deleteCommand(type.java, id)
.let {
if (block === null) {
it
} else {
val dsl = KDeleteCommandDslImpl(it)
dsl.block()
dsl.javaCommand
}
}
.execute(con)
.let { KDeleteResultImpl(it) }
override fun deleteAll(
type: KClass<*>,
ids: Iterable<*>,
con: Connection?,
block: (KDeleteCommandDsl.() -> Unit)?
): KDeleteResult =
javaEntities
.deleteAllCommand(type.java, ids)
.let {
if (block === null) {
it
} else {
val dsl = KDeleteCommandDslImpl(it)
dsl.block()
dsl.javaCommand
}
}
.execute(con)
.let { KDeleteResultImpl(it) }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy