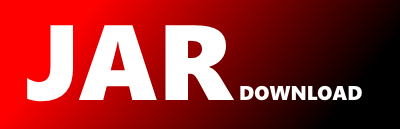
org.openmrs.module.rulesengine.rule.BSABasedDosageRule Maven / Gradle / Ivy
package org.openmrs.module.rulesengine.rule;
import org.openmrs.Patient;
import org.openmrs.module.rulesengine.domain.DosageRequest;
import org.openmrs.module.rulesengine.domain.Dose;
import org.openmrs.module.rulesengine.domain.RuleName;
import org.openmrs.module.rulesengine.service.ObservationService;
import org.openmrs.module.rulesengine.service.PatientService;
import org.openmrs.module.rulesengine.util.BahmniMath;
import org.openmrs.module.rulesengine.util.Validator;
import java.util.Date;
@RuleName(name = "mg/m2")
public class BSABasedDosageRule implements DosageRule {
public static Double calculateBSA(Double height, Double weight, Integer patientAgeInYears) throws Exception {
if (patientAgeInYears <= 15 && weight <= 40) {
return Math.sqrt(weight * height / 3600);
}
return Math.pow(weight, 0.425) * Math.pow(height, 0.725) * 0.007184;
}
public Dose calculateDose(DosageRequest request) throws Exception {
String visitUuid = request.getVisitUuid();
Patient patient = PatientService.getPatientByUuid(request.getPatientUuid());
Double height = ObservationService.getLatestObsValueNumeric(patient, ObservationService.ConceptRepo.HEIGHT, visitUuid);
Double weight = ObservationService.getLatestObsValueNumeric(patient, ObservationService.ConceptRepo.WEIGHT, visitUuid);
Validator.validateHeightAndWeight(height, weight, ObservationService.ConceptRepo.HEIGHT, ObservationService.ConceptRepo.WEIGHT);
Date asOfDate = new Date();
Integer ageInYears = BahmniMath.ageInYears(patient.getBirthdate(), asOfDate);
Double bsa = calculateBSA(height, weight, ageInYears);
double roundedUpValue = BahmniMath.getTwoDigitRoundUpValue(request.getBaseDose() * bsa);
return new Dose(request.getDrugName(),roundedUpValue, Dose.DoseUnit.mg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy