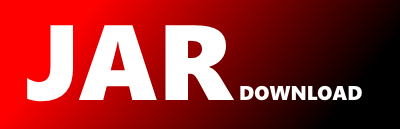
javax.persistence.criteria.Path Maven / Gradle / Ivy
The newest version!
package javax.persistence.criteria;
import java.util.Collection;
import java.util.Map;
import javax.persistence.metamodel.Bindable;
import javax.persistence.metamodel.MapAttribute;
import javax.persistence.metamodel.PluralAttribute;
import javax.persistence.metamodel.SingularAttribute;
/**
* Represents a simple or compound attribute path from a bound type or collection, and is a "primitive" expression.
*
* @param
* the type referenced by the path
*/
public interface Path extends Expression {
/**
* Create a path corresponding to the referenced map-valued attribute.
*
* @param map
* map-valued attribute
* @param
* the type of the key
* @param
* the type of the value
* @param
* the type of the map
* @return expression corresponding to the referenced attribute
*/
> Expression get(MapAttribute super X, K, V> map);
/**
* Create a path corresponding to the referenced collection-valued attribute.
*
* @param collection
* collection-valued attribute
* @param
* the type of the element
* @param
* the type of the collection
* @return expression corresponding to the referenced attribute
*/
> Expression get(PluralAttribute super X, C, E> collection);
/**
* Create a path corresponding to the referenced single-valued attribute.
*
* @param attribute
* single-valued attribute
* @param
* the type of the path
* @return path corresponding to the referenced attribute
*/
Path get(SingularAttribute super X, Y> attribute);
/**
* Create a path corresponding to the referenced attribute.
*
*
* Note: Applications using the string-based API may need to specify the type resulting from the get operation in order to avoid the use
* of Path variables.
*
*
* For example:
*
* CriteriaQuery<Person> q = cb.createQuery(Person.class);
* Root<Person> p = q.from(Person.class);
* q.select(p)
* .where(cb.isMember("joe",
* p.<Set<String>>get("nicknames")));
*
* rather than:
*
* CriteriaQuery<Person> q = cb.createQuery(Person.class);
* Root<Person> p = q.from(Person.class);
* Path<Set<String>> nicknames = p.get("nicknames");
* q.select(p)
* .where(cb.isMember("joe", nicknames));
*
*
* @param attributeName
* name of the attribute
* @param
* the type of the path
* @return path corresponding to the referenced attribute
* @throws IllegalStateException
* if invoked on a path that corresponds to a basic type
* @throws IllegalArgumentException
* if attribute of the given name does not otherwise exist
*/
Path get(String attributeName);
/**
* Return the bindable object that corresponds to the path expression.
*
* @return bindable object corresponding to the path
*/
Bindable getModel();
/**
* Return the parent "node" in the path or null if no parent.
*
* @return parent
*/
Path> getParentPath();
/**
* Create an expression corresponding to the type of the path.
*
* @return expression corresponding to the type of the path
*/
Expression> type();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy