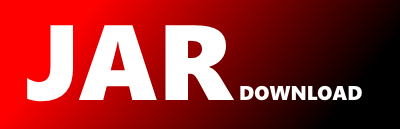
org.bdware.datanet.YPKPacker Maven / Gradle / Ivy
package org.bdware.datanet;
import java.io.File;
import java.io.FileFilter;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.*;
import java.util.regex.Pattern;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import java.util.zip.ZipOutputStream;
public class YPKPacker {
public static void staticPack(String source, String target) {
new YPKPacker().pack(source, target);
}
public static void mergeJar(String s1, String s2, String target) {
String[] all = new String[]{s1, s2};
try {
Set already = new HashSet<>();
System.out.println("Input:" + new File(s1).getAbsolutePath());
ZipOutputStream zout = new ZipOutputStream(new FileOutputStream(target, false));
for (String source : all) {
ZipInputStream zin = new ZipInputStream(new FileInputStream(source));
byte[] buff = new byte[10240];
for (ZipEntry entry; (entry = zin.getNextEntry()) != null; ) {
ZipEntry nEntry = new ZipEntry("/" + entry.getName());
if (already.contains("/" + entry.getName())) {
zin.closeEntry();
continue;
} else already.add("/" + entry.getName());
zout.putNextEntry(nEntry);
for (int k; (k = zin.read(buff)) > 0; ) {
zout.write(buff, 0, k);
}
zout.closeEntry();
}
}
zout.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static int grepJarByCPVersion(String target, CPVersion version) {
return deleteJarAtList(target, version.getFileList());
}
public static int grepJarByListFile(String target, String list) {
int ret = 0;
try {
Scanner sc = new Scanner(new FileInputStream(list));
List toDelete = new ArrayList<>();
for (; sc.hasNextLine(); ) {
toDelete.add(sc.nextLine());
}
ret = deleteJarAtList(target, toDelete);
} catch (Exception e) {
e.printStackTrace();
}
return ret;
}
public static int grepJarByDir(String target, String cpDir) {
int ret = 0;
try {
System.out.println(String.format("[SimpleYPKPacker] cp:%s, target:%s",
new File(cpDir).getAbsolutePath(), new File(target).getAbsolutePath()));
File[] cpJar = new File(cpDir).listFiles();
List cpJars = new ArrayList<>();
for (File f : cpJar) {
if (f.getName().endsWith(".jar"))
cpJars.add(f.getName());
if (f.isDirectory()) {
File[] subList = f.listFiles(new FileFilter() {
@Override
public boolean accept(File pathname) {
return pathname.getName().endsWith(".jar");
}
});
if (subList != null)
for (File subFile : subList)
cpJars.add(subFile.getName());
}
}
ret = deleteJarAtList(target, cpJars);
} catch (Exception e) {
e.printStackTrace();
}
return ret;
}
private static int deleteJarAtList(String target, List toDelete) {
int ret = 0;
try {
FileFilter jarFilter = new FileFilter() {
@Override
public boolean accept(File pathname) {
return toDelete.contains(pathname.getName());
}
};
File[] targetJar = new File(target).listFiles(jarFilter);
if (targetJar != null)
for (File f : targetJar) {
f.delete();
ret++;
}
targetJar = new File(target).listFiles();
if (targetJar != null) {
for (File f : targetJar)
if (f.getName().endsWith(".jar"))
diffVersion(f, toDelete);
}
} catch (Exception e) {
e.printStackTrace();
}
System.out.println(String.format("[SimpleYPKPacker] delete count:" + ret));
return ret;
}
private static boolean diffVersion(File f, List cpJar) {
for (String cp : cpJar) {
String f1 = f.getName();
String f2 = cp;
if (f1.length() > f2.length()) {
String temp = f1;
f1 = f2;
f2 = temp;
}
int start = 0;
int end = f1.length() - 1;
int delta = f2.length() - f1.length();
for (; start <= end && f1.charAt(start) == f2.charAt(start); )
start++;
for (; end >= start && f1.charAt(end) == f2.charAt(delta + end); )
end--;
if (start < end) {
String diff = f1.substring(start, end);
if (Pattern.matches("[0-9.]*", diff)) {
System.err.println("[SimpleYPKPacker] grep cp lib, similar:"
+ f.getName() + "-> cp:" + cp);
return true;
}
}
}
return false;
}
private static boolean inCP(File f, List cpJar) {
for (String cpName : cpJar)
if (cpName.equals(f.getName()))
return true;
return false;
}
public void pack(String source, String target) {
try {
ZipInputStream zin = new ZipInputStream(new FileInputStream(source));
ZipOutputStream zout = new ZipOutputStream(new FileOutputStream(target));
byte[] buff = new byte[10240];
for (ZipEntry entry; (entry = zin.getNextEntry()) != null; ) {
ZipEntry nEntry = new ZipEntry("/" + entry.getName());
zout.putNextEntry(nEntry);
for (int k; (k = zin.read(buff)) > 0; ) {
zout.write(buff, 0, k);
}
zin.closeEntry();
zout.closeEntry();
}
zout.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy