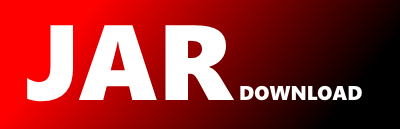
org.bdware.doip.event.verified.VerifiedEventQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doip-audit-tool Show documentation
Show all versions of doip-audit-tool Show documentation
doip audit tool developed by bdware
The newest version!
package org.bdware.doip.event.verified;
import org.bdware.doip.codec.doipMessage.DoipMessageFactory;
import org.bdware.doip.codec.operations.BasicOperations;
import org.bdware.doip.endpoint.event.SubscribeMessageType;
import java.util.Comparator;
import java.util.PriorityQueue;
import java.util.concurrent.atomic.AtomicInteger;
public class VerifiedEventQueue {
String topicId;
String publisherId;
VerifiedSubscriber verifiedSubscriber;
PriorityQueue priorityQueue = new PriorityQueue();
//是否为从磁盘里读取的值?
AtomicInteger count = new AtomicInteger(0);
public VerifiedEventQueue() {
new Thread() {
@Override
public void run() {
for (; ; ) {
Pack pack = priorityQueue.peek();
if (pack.order - 1 == count.get()) {
appendEvent(pack.order, pack.hash, pack.content);
priorityQueue.poll();
} else if (pack.order - 1 < count.get()) {
priorityQueue.poll();
} else if (pack.order - 1 > count.get()) {
synchronized (priorityQueue) {
try {
priorityQueue.wait();
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
}
}
}.start();
}
static class Pack implements Comparator {
int order;
String hash;
String content;
public Pack(int order, String hash, String content) {
this.order = order;
this.hash = hash;
this.content = content;
}
@Override
public int compare(Pack o1, Pack o2) {
return o1.order - o2.order;
}
}
public synchronized void appendEvent(int order, String hash, String content) {
int nextOrder = count.get() + 1;
if (!HashUtil.verify(content, hash)) {
resend(order, 1);
return;
}
if (order == nextOrder) {
DoipMessageFactory.DoipMessageBuilder builder = new DoipMessageFactory.DoipMessageBuilder();
builder.createRequest(verifiedSubscriber.getId(), BasicOperations.Publish.getName());
builder.setBody(content.getBytes());
verifiedSubscriber.commit(topicId, publisherId, builder.create());
count.getAndIncrement();
triggerConsumeCache();
} else if (order > nextOrder) {
saveInCache(order, hash, content);
resend(nextOrder, order - nextOrder);
} else if (order < nextOrder) {
}
return;
}
private void triggerConsumeCache() {
synchronized (priorityQueue) {
priorityQueue.notify();
}
}
private void saveInCache(int order, String hash, String content) {
priorityQueue.add(new Pack(order, hash, content));
}
private void resend(int offset, int count) {
DoipMessageFactory.DoipMessageBuilder builder = new DoipMessageFactory.DoipMessageBuilder();
builder.createRequest(publisherId, BasicOperations.Subscribe.getName());
builder.addAttributes("topicId", topicId);
builder.addAttributes("subscribeType", SubscribeMessageType.DataInRange.name());
builder.addAttributes("subscirberId", verifiedSubscriber.getId());
builder.addAttributes("offset", offset);
builder.addAttributes("count", count);
verifiedSubscriber.client.sendRawMessageSync(builder.create(), 5000);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy