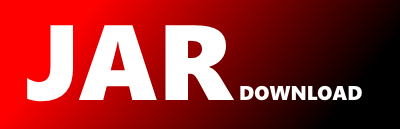
org.bdware.doip.codec.v3.DOIPV3Codec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doip-sdk Show documentation
Show all versions of doip-sdk Show documentation
doip sdk developed by bdware
package org.bdware.doip.codec.v3;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.ByteBufUtil;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ByteToMessageCodec;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.List;
public class DOIPV3Codec extends ByteToMessageCodec {
static Logger LOGGER = LogManager.getLogger(DOIPV3Message.class);
@Override
protected void encode(ChannelHandlerContext ctx, DOIPV3Message msg, ByteBuf out) throws Exception {
LOGGER.info("[DOIPV3Codec Send] " + msg.prettyPrint() + " from:" + ctx.channel().remoteAddress().toString());
msg.writeTo(out);
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy