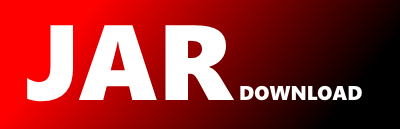
org.bdware.doip.endpoint.client.v3.Client Maven / Gradle / Ivy
/*
* Copyright (c) [2021] [Peking University]
* [BDWare DOIP SDK] is licensed under Mulan PSL v2.
* You can use this software according to the terms and conditions of the Mulan PSL v2.
* You may obtain a copy of Mulan PSL v2 at:
* http://license.coscl.org.cn/MulanPSL2
* THIS SOFTWARE IS PROVIDED ON AN "AS IS" BASIS, WITHOUT WARRANTIES OF ANY KIND, EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO NON-INFRINGEMENT, MERCHANTABILITY OR FIT FOR A PARTICULAR PURPOSE.
* See the Mulan PSL v2 for more details.
*/
package org.bdware.doip.endpoint.client.v3;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.bdware.doip.codec.exception.DoipConnectException;
import org.bdware.doip.codec.v3.DOIPV3Message;
import org.bdware.doip.codec.v3.V3MsgFactory;
import org.bdware.doip.endpoint.client.ClientConfig;
import java.io.ByteArrayOutputStream;
import java.io.PrintStream;
import java.net.URISyntaxException;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.TimeUnit;
public class Client {
TCPClientChannel clientChannel;
String serverURL = null;
Logger logger = LogManager.getLogger(Client.class);
V3MsgFactory factory = new V3MsgFactory();
int timeoutSeconds = 5;
public DOIPV3Message sendMessageSync(DOIPV3Message doipMessage, long timeout) {
CompletableFuture result = new CompletableFuture<>();
sendMessage(doipMessage, result::complete);
try {
return result.get(timeout, TimeUnit.MILLISECONDS);
} catch (Exception e) {
ByteArrayOutputStream bo = new ByteArrayOutputStream();
e.printStackTrace(new PrintStream(bo));
return factory.createErrorMessage(doipMessage.requestID, bo.toString());
}
}
public void sendMessage(DOIPV3Message msg, MessageCallback cb) {
if (!isConnected()) {
if (!tryReconnect()) {
logger.warn("channel not connect yet! " + (clientChannel == null) + " --> serverUrl:" + serverURL);
cb.onResult(factory.createErrorMessage(msg.requestID, "Failed to connect channel:" + serverURL));
return;
}
}
clientChannel.sendMessage(msg, cb);
}
public void close() {
clientChannel.close();
clientChannel = null;
}
public void connect(ClientConfig config) {
try {
serverURL = config.url;
clientChannel = new TCPClientChannel(config);
if (clientChannel == null) return;
clientChannel.connect(serverURL);
clientChannel.setTimeoutSecond(config.timeoutSeconds);
} catch (URISyntaxException e) {
logger.error("UUUUUURISyntaxException Exception!");
e.printStackTrace();
} catch (InterruptedException e) {
logger.error("IIIIInterruped Exception!");
throw new RuntimeException(e);
}
}
public void reconnect() throws DoipConnectException {
if (serverURL == null) throw (new DoipConnectException("target URL not set, use .connect(url) first"));
ClientConfig clientConfig = ClientConfig.fromUrl(serverURL);
if (clientChannel == null) clientChannel = new TCPClientChannel(clientConfig);
if (clientChannel == null) return;
try {
clientChannel.connect(serverURL);
} catch (URISyntaxException | InterruptedException e) {
e.printStackTrace();
}
}
public void reconnect(String url) throws DoipConnectException {
serverURL = url;
if (serverURL == null) throw (new DoipConnectException("target URL not set, use .connect(url) first"));
ClientConfig clientConfig = ClientConfig.fromUrl(serverURL);
if (clientChannel == null) clientChannel = new TCPClientChannel(clientConfig);
if (clientChannel == null) return;
try {
clientChannel.connect(serverURL);
} catch (URISyntaxException | InterruptedException e) {
e.printStackTrace();
}
}
public boolean isConnected() {
return clientChannel != null && clientChannel.isConnected();
}
public void waitForConnected() {
for (int i = 0; i < 100; i++) {
if (clientChannel == null || !clientChannel.isConnected()) {
try {
Thread.sleep(20);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
continue;
}
break;
}
}
private boolean tryReconnect() {
try {
reconnect();
} catch (DoipConnectException e) {
throw new RuntimeException(e);
}
return isConnected();
}
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy