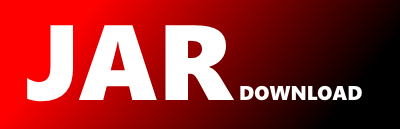
org.beangle.commons.dao.EntityDao Maven / Gradle / Ivy
The newest version!
/*
* Beangle, Agile Development Scaffold and Toolkits.
*
* Copyright © 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.beangle.commons.dao;
import java.io.InputStream;
import java.io.Serializable;
import java.sql.Blob;
import java.sql.Clob;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.beangle.commons.collection.page.PageLimit;
import org.beangle.commons.dao.query.Query;
import org.beangle.commons.dao.query.QueryBuilder;
import org.beangle.commons.entity.Entity;
/**
* dao 查询辅助类
*
* @author chaostone
*/
public interface EntityDao {
/**
* 查询指定id的对象
*
* @param clazz 类型
* @param id 唯一标识
* @return null
*/
, ID extends Serializable> T get(Class clazz, ID id);
/**
* Returns model by identifier,null when not found.
*
* @param entityName
* @param id
*/
T get(String entityName, Serializable id);
/**
* Returns a list of all entity of clazz.
*
* @param clazz
*/
> List getAll(Class clazz);
/**
* 根据属性列举实体
*
* @param entityClass
* @param values
*/
, ID extends Serializable> List get(Class entityClass, ID[] values);
/**
* 根据属性列举实体
*
* @param entityClass
* @param values
*/
, ID extends Serializable> List get(Class entityClass, Collection values);
/**
* 根据属性列举实体
*
* @param clazz
* @param keyName
* @param values
*/
> List get(Class clazz, String keyName, Object... values);
/**
* 根据属性列举实体
*
* @param
* @param clazz
* @param keyName
* @param values
*/
> List get(Class clazz, String keyName, Collection values);
/**
* @param
* @param clazz
* @param attrs
* @param values
*/
> List get(Class clazz, String[] attrs, Object... values);
/**
* @param
* @param clazz
* @param parameterMap
*/
> List get(Class clazz, Map parameterMap);
/**
* 根据属性列举实体
*
* @param entityName
* @param keyName
* @param values
*/
List get(String entityName, String keyName, Object... values);
/**
* 执行查询
*
* @param query
*/
List search(Query query);
/**
* 查询hql语句
*
* @param
* @param builder
*/
List search(QueryBuilder builder);
/**
* 查询hql语句
*
* @param
* @param builder
*/
T uniqueResult(QueryBuilder builder);
/**
* JPQL/NamedQuery
* query语句中使用?1表示参数.NamedQuery使用@Named-Query-Name
*
* @param query
* @param params
*/
List search(final String query, final Object... params);
/**
* JPQL/NamedQuery
*
* @param query
* @param params
*/
List search(final String query, final Map params);
/**
* 支持缓存的JPQL/NamedQuery
* 查询
*
* @param query
* @param params
* @param limit
* @param cacheable
*/
List search(String query, final Map params, PageLimit limit, boolean cacheable);
/**
* 执行JPQL/NamedQuery 进行更新或者删除
*
* @param query
* @param arguments
*/
int executeUpdate(String query, Object... arguments);
/**
* 重复执行单个JPQL/NamedQuery语句
*
* @param query
* @param arguments
*/
int[] executeUpdateRepeatly(String query, Collection