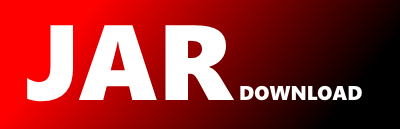
org.beangle.commons.dao.query.builder.Conditions Maven / Gradle / Ivy
The newest version!
/*
* Beangle, Agile Development Scaffold and Toolkits.
*
* Copyright © 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.beangle.commons.dao.query.builder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.beangle.commons.bean.PropertyUtils;
import org.beangle.commons.collection.CollectUtils;
import org.beangle.commons.entity.Component;
import org.beangle.commons.entity.Entity;
import org.beangle.commons.entity.util.ValidEntityKeyPredicate;
import org.beangle.commons.lang.Assert;
import org.beangle.commons.lang.Strings;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* 条件提取辅助类
*
* @author chaostone
*/
public final class Conditions {
private static final Logger logger = LoggerFactory.getLogger(Conditions.class);
private Conditions() {
super();
}
public static Condition and(Condition... conditions) {
return concat(Arrays.asList(conditions), "and");
}
public static Condition and(List conditions) {
return concat(conditions, "and");
}
public static Condition or(Condition... conditions) {
return concat(Arrays.asList(conditions), "or");
}
public static Condition or(List conditions) {
return concat(conditions, "or");
}
static Condition concat(List conditions, String andor) {
Assert.isTrue(!conditions.isEmpty(), "conditions shouldn't be empty!");
if (conditions.size() == 1) return conditions.get(0);
StringBuffer sb = new StringBuffer();
List