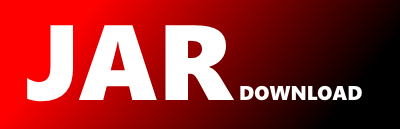
org.beangle.commons.transfer.csv.CsvItemWriter Maven / Gradle / Ivy
The newest version!
/*
* Beangle, Agile Development Scaffold and Toolkits.
*
* Copyright © 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.beangle.commons.transfer.csv;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import org.beangle.commons.csv.CsvFormat;
import org.beangle.commons.csv.CsvWriter;
import org.beangle.commons.lang.Charsets;
import org.beangle.commons.transfer.io.AbstractItemWriter;
import org.beangle.commons.transfer.io.TransferFormat;
/**
*
* CsvItemWriter class.
*
*
* @author chaostone
* @version $Id: $
*/
public class CsvItemWriter extends AbstractItemWriter {
protected CsvWriter csvr;
protected CsvFormat csvFormat;
/**
*
* Constructor for CsvItemWriter.
*
*/
public CsvItemWriter() {
super();
}
/**
*
* Constructor for CsvItemWriter.
*
*
* @param outputStream a {@link java.io.OutputStream} object.
*/
public CsvItemWriter(OutputStream outputStream) {
setOutputStream(outputStream);
}
public void write(Object obj) {
if (null == csvr) {
if (null == csvFormat) {
this.csvr = new CsvWriter(new OutputStreamWriter(outputStream, Charsets.UTF_8));
} else {
this.csvr = new CsvWriter(new OutputStreamWriter(outputStream, Charsets.UTF_8), csvFormat);
}
}
if (null == obj) return;
try {
if (obj.getClass().isArray()) {
Object[] values = (Object[]) obj;
String[] strValues = new String[values.length];
for (int i = 0; i < values.length; i++) {
strValues[i] = (null == values[i]) ? "" : values[i].toString();
}
csvr.write(strValues);
} else {
csvr.write(new String[] { obj.toString() });
}
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
}
/** {@inheritDoc} */
public void writeTitle(String titleName, Object data) {
write(data);
}
/**
*
* close.
*
*/
public void flush() {
try {
csvr.close();
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
}
/**
*
* getFormat.
*
*
* @return a {@link java.lang.String} object.
*/
public TransferFormat getFormat() {
return TransferFormat.Csv;
}
/** {@inheritDoc} */
public void setOutputStream(OutputStream outputStream) {
this.outputStream = outputStream;
}
/**
*
* Getter for the field csvFormat
.
*
*
* @return a {@link org.beangle.commons.csv.CsvFormat} object.
*/
public CsvFormat getCsvFormat() {
return csvFormat;
}
/**
*
* Setter for the field csvFormat
.
*
*
* @param csvFormat a {@link org.beangle.commons.csv.CsvFormat} object.
*/
public void setCsvFormat(CsvFormat csvFormat) {
this.csvFormat = csvFormat;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy