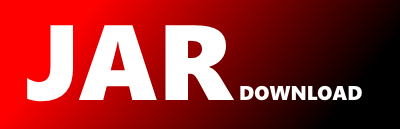
org.beangle.commons.transfer.exporter.Context Maven / Gradle / Ivy
The newest version!
/*
* Beangle, Agile Development Scaffold and Toolkits.
*
* Copyright © 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.beangle.commons.transfer.exporter;
import java.util.Map;
import org.beangle.commons.collection.CollectUtils;
/**
*
* Context class.
*
*
* @author chaostone
* @version $Id: $
*/
public class Context {
Map datas = CollectUtils.newHashMap();
/** Constant KEYS="keys"
*/
public static final String KEYS = "keys";
/** Constant TITLES="titles"
*/
public static final String TITLES = "titles";
/** Constant EXTRACTOR="extractor"
*/
public static final String EXTRACTOR = "extractor";
/**
*
* Getter for the field datas
.
*
*
* @return a {@link java.util.Map} object.
*/
public Map getDatas() {
return datas;
}
/**
*
* Setter for the field datas
.
*
*
* @param datas a {@link java.util.Map} object.
*/
public void setDatas(Map datas) {
this.datas = datas;
}
/**
*
* put.
*
*
* @param key a {@link java.lang.String} object.
* @param obj a {@link java.lang.Object} object.
*/
public void put(String key, Object obj) {
datas.put(key, obj);
}
/**
*
* get.
*
*
* @param key a {@link java.lang.String} object.
* @return a {@link java.lang.Object} object.
*/
public Object get(String key) {
return datas.get(key);
}
/**
*
* get.
*
*
* @param key a {@link java.lang.String} object.
* @param clazz a {@link java.lang.Class} object.
* @param a T object.
* @return a T object.
*/
@SuppressWarnings("unchecked")
public T get(String key, Class clazz) {
return (T) datas.get(key);
}
}