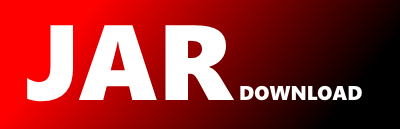
org.beangle.ems.app.log.BusinessLogProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-ems-app Show documentation
Show all versions of beangle-ems-app Show documentation
The Beangle EMS Application
The newest version!
/*
* Copyright (C) 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: src/main/resources/org/beangle/ems/app/log/model.proto
// Protobuf Java Version: 4.28.2
package org.beangle.ems.app.log;
public final class BusinessLogProto {
private BusinessLogProto() {}
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
BusinessLogProto.class.getName());
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface BusinessLogEventOrBuilder extends
// @@protoc_insertion_point(interface_extends:BusinessLogEvent)
com.google.protobuf.MessageOrBuilder {
/**
* string operator = 1;
* @return The operator.
*/
java.lang.String getOperator();
/**
* string operator = 1;
* @return The bytes for operator.
*/
com.google.protobuf.ByteString
getOperatorBytes();
/**
* int64 operateAt = 2;
* @return The operateAt.
*/
long getOperateAt();
/**
* string summary = 3;
* @return The summary.
*/
java.lang.String getSummary();
/**
* string summary = 3;
* @return The bytes for summary.
*/
com.google.protobuf.ByteString
getSummaryBytes();
/**
* string details = 4;
* @return The details.
*/
java.lang.String getDetails();
/**
* string details = 4;
* @return The bytes for details.
*/
com.google.protobuf.ByteString
getDetailsBytes();
/**
* string resources = 5;
* @return The resources.
*/
java.lang.String getResources();
/**
* string resources = 5;
* @return The bytes for resources.
*/
com.google.protobuf.ByteString
getResourcesBytes();
/**
* string ip = 6;
* @return The ip.
*/
java.lang.String getIp();
/**
* string ip = 6;
* @return The bytes for ip.
*/
com.google.protobuf.ByteString
getIpBytes();
/**
* string agent = 7;
* @return The agent.
*/
java.lang.String getAgent();
/**
* string agent = 7;
* @return The bytes for agent.
*/
com.google.protobuf.ByteString
getAgentBytes();
/**
* string entry = 8;
* @return The entry.
*/
java.lang.String getEntry();
/**
* string entry = 8;
* @return The bytes for entry.
*/
com.google.protobuf.ByteString
getEntryBytes();
/**
* int32 level = 9;
* @return The level.
*/
int getLevel();
/**
* string appName = 10;
* @return The appName.
*/
java.lang.String getAppName();
/**
* string appName = 10;
* @return The bytes for appName.
*/
com.google.protobuf.ByteString
getAppNameBytes();
}
/**
* Protobuf type {@code BusinessLogEvent}
*/
public static final class BusinessLogEvent extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:BusinessLogEvent)
BusinessLogEventOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
BusinessLogEvent.class.getName());
}
// Use BusinessLogEvent.newBuilder() to construct.
private BusinessLogEvent(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private BusinessLogEvent() {
operator_ = "";
summary_ = "";
details_ = "";
resources_ = "";
ip_ = "";
agent_ = "";
entry_ = "";
appName_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.beangle.ems.app.log.BusinessLogProto.internal_static_BusinessLogEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.beangle.ems.app.log.BusinessLogProto.internal_static_BusinessLogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.class, org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.Builder.class);
}
public static final int OPERATOR_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object operator_ = "";
/**
* string operator = 1;
* @return The operator.
*/
@java.lang.Override
public java.lang.String getOperator() {
java.lang.Object ref = operator_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operator_ = s;
return s;
}
}
/**
* string operator = 1;
* @return The bytes for operator.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOperatorBytes() {
java.lang.Object ref = operator_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPERATEAT_FIELD_NUMBER = 2;
private long operateAt_ = 0L;
/**
* int64 operateAt = 2;
* @return The operateAt.
*/
@java.lang.Override
public long getOperateAt() {
return operateAt_;
}
public static final int SUMMARY_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object summary_ = "";
/**
* string summary = 3;
* @return The summary.
*/
@java.lang.Override
public java.lang.String getSummary() {
java.lang.Object ref = summary_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
summary_ = s;
return s;
}
}
/**
* string summary = 3;
* @return The bytes for summary.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSummaryBytes() {
java.lang.Object ref = summary_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
summary_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DETAILS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object details_ = "";
/**
* string details = 4;
* @return The details.
*/
@java.lang.Override
public java.lang.String getDetails() {
java.lang.Object ref = details_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
details_ = s;
return s;
}
}
/**
* string details = 4;
* @return The bytes for details.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDetailsBytes() {
java.lang.Object ref = details_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
details_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCES_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object resources_ = "";
/**
* string resources = 5;
* @return The resources.
*/
@java.lang.Override
public java.lang.String getResources() {
java.lang.Object ref = resources_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resources_ = s;
return s;
}
}
/**
* string resources = 5;
* @return The bytes for resources.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourcesBytes() {
java.lang.Object ref = resources_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resources_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IP_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object ip_ = "";
/**
* string ip = 6;
* @return The ip.
*/
@java.lang.Override
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ip_ = s;
return s;
}
}
/**
* string ip = 6;
* @return The bytes for ip.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AGENT_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object agent_ = "";
/**
* string agent = 7;
* @return The agent.
*/
@java.lang.Override
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
agent_ = s;
return s;
}
}
/**
* string agent = 7;
* @return The bytes for agent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENTRY_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object entry_ = "";
/**
* string entry = 8;
* @return The entry.
*/
@java.lang.Override
public java.lang.String getEntry() {
java.lang.Object ref = entry_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entry_ = s;
return s;
}
}
/**
* string entry = 8;
* @return The bytes for entry.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEntryBytes() {
java.lang.Object ref = entry_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
entry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LEVEL_FIELD_NUMBER = 9;
private int level_ = 0;
/**
* int32 level = 9;
* @return The level.
*/
@java.lang.Override
public int getLevel() {
return level_;
}
public static final int APPNAME_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object appName_ = "";
/**
* string appName = 10;
* @return The appName.
*/
@java.lang.Override
public java.lang.String getAppName() {
java.lang.Object ref = appName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
appName_ = s;
return s;
}
}
/**
* string appName = 10;
* @return The bytes for appName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppNameBytes() {
java.lang.Object ref = appName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(operator_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, operator_);
}
if (operateAt_ != 0L) {
output.writeInt64(2, operateAt_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(summary_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, summary_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(details_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, details_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(resources_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, resources_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(ip_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, ip_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(agent_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, agent_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(entry_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 8, entry_);
}
if (level_ != 0) {
output.writeInt32(9, level_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(appName_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, appName_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(operator_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, operator_);
}
if (operateAt_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, operateAt_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(summary_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, summary_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(details_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, details_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(resources_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, resources_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(ip_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, ip_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(agent_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(7, agent_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(entry_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(8, entry_);
}
if (level_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, level_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(appName_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, appName_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent)) {
return super.equals(obj);
}
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent other = (org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent) obj;
if (!getOperator()
.equals(other.getOperator())) return false;
if (getOperateAt()
!= other.getOperateAt()) return false;
if (!getSummary()
.equals(other.getSummary())) return false;
if (!getDetails()
.equals(other.getDetails())) return false;
if (!getResources()
.equals(other.getResources())) return false;
if (!getIp()
.equals(other.getIp())) return false;
if (!getAgent()
.equals(other.getAgent())) return false;
if (!getEntry()
.equals(other.getEntry())) return false;
if (getLevel()
!= other.getLevel()) return false;
if (!getAppName()
.equals(other.getAppName())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPERATOR_FIELD_NUMBER;
hash = (53 * hash) + getOperator().hashCode();
hash = (37 * hash) + OPERATEAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOperateAt());
hash = (37 * hash) + SUMMARY_FIELD_NUMBER;
hash = (53 * hash) + getSummary().hashCode();
hash = (37 * hash) + DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getDetails().hashCode();
hash = (37 * hash) + RESOURCES_FIELD_NUMBER;
hash = (53 * hash) + getResources().hashCode();
hash = (37 * hash) + IP_FIELD_NUMBER;
hash = (53 * hash) + getIp().hashCode();
hash = (37 * hash) + AGENT_FIELD_NUMBER;
hash = (53 * hash) + getAgent().hashCode();
hash = (37 * hash) + ENTRY_FIELD_NUMBER;
hash = (53 * hash) + getEntry().hashCode();
hash = (37 * hash) + LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getLevel();
hash = (37 * hash) + APPNAME_FIELD_NUMBER;
hash = (53 * hash) + getAppName().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code BusinessLogEvent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:BusinessLogEvent)
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.beangle.ems.app.log.BusinessLogProto.internal_static_BusinessLogEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.beangle.ems.app.log.BusinessLogProto.internal_static_BusinessLogEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.class, org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.Builder.class);
}
// Construct using org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
operator_ = "";
operateAt_ = 0L;
summary_ = "";
details_ = "";
resources_ = "";
ip_ = "";
agent_ = "";
entry_ = "";
level_ = 0;
appName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.beangle.ems.app.log.BusinessLogProto.internal_static_BusinessLogEvent_descriptor;
}
@java.lang.Override
public org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent getDefaultInstanceForType() {
return org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.getDefaultInstance();
}
@java.lang.Override
public org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent build() {
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent buildPartial() {
org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent result = new org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.operator_ = operator_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.operateAt_ = operateAt_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.summary_ = summary_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.details_ = details_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.resources_ = resources_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.ip_ = ip_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.agent_ = agent_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.entry_ = entry_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.level_ = level_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.appName_ = appName_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent) {
return mergeFrom((org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent other) {
if (other == org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent.getDefaultInstance()) return this;
if (!other.getOperator().isEmpty()) {
operator_ = other.operator_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getOperateAt() != 0L) {
setOperateAt(other.getOperateAt());
}
if (!other.getSummary().isEmpty()) {
summary_ = other.summary_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getDetails().isEmpty()) {
details_ = other.details_;
bitField0_ |= 0x00000008;
onChanged();
}
if (!other.getResources().isEmpty()) {
resources_ = other.resources_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.getIp().isEmpty()) {
ip_ = other.ip_;
bitField0_ |= 0x00000020;
onChanged();
}
if (!other.getAgent().isEmpty()) {
agent_ = other.agent_;
bitField0_ |= 0x00000040;
onChanged();
}
if (!other.getEntry().isEmpty()) {
entry_ = other.entry_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.getLevel() != 0) {
setLevel(other.getLevel());
}
if (!other.getAppName().isEmpty()) {
appName_ = other.appName_;
bitField0_ |= 0x00000200;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
operator_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
operateAt_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
summary_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
details_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
resources_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
ip_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
agent_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
entry_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 66
case 72: {
level_ = input.readInt32();
bitField0_ |= 0x00000100;
break;
} // case 72
case 82: {
appName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 82
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object operator_ = "";
/**
* string operator = 1;
* @return The operator.
*/
public java.lang.String getOperator() {
java.lang.Object ref = operator_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operator_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string operator = 1;
* @return The bytes for operator.
*/
public com.google.protobuf.ByteString
getOperatorBytes() {
java.lang.Object ref = operator_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string operator = 1;
* @param value The operator to set.
* @return This builder for chaining.
*/
public Builder setOperator(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
operator_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string operator = 1;
* @return This builder for chaining.
*/
public Builder clearOperator() {
operator_ = getDefaultInstance().getOperator();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string operator = 1;
* @param value The bytes for operator to set.
* @return This builder for chaining.
*/
public Builder setOperatorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
operator_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private long operateAt_ ;
/**
* int64 operateAt = 2;
* @return The operateAt.
*/
@java.lang.Override
public long getOperateAt() {
return operateAt_;
}
/**
* int64 operateAt = 2;
* @param value The operateAt to set.
* @return This builder for chaining.
*/
public Builder setOperateAt(long value) {
operateAt_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int64 operateAt = 2;
* @return This builder for chaining.
*/
public Builder clearOperateAt() {
bitField0_ = (bitField0_ & ~0x00000002);
operateAt_ = 0L;
onChanged();
return this;
}
private java.lang.Object summary_ = "";
/**
* string summary = 3;
* @return The summary.
*/
public java.lang.String getSummary() {
java.lang.Object ref = summary_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
summary_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string summary = 3;
* @return The bytes for summary.
*/
public com.google.protobuf.ByteString
getSummaryBytes() {
java.lang.Object ref = summary_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
summary_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string summary = 3;
* @param value The summary to set.
* @return This builder for chaining.
*/
public Builder setSummary(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
summary_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string summary = 3;
* @return This builder for chaining.
*/
public Builder clearSummary() {
summary_ = getDefaultInstance().getSummary();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string summary = 3;
* @param value The bytes for summary to set.
* @return This builder for chaining.
*/
public Builder setSummaryBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
summary_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object details_ = "";
/**
* string details = 4;
* @return The details.
*/
public java.lang.String getDetails() {
java.lang.Object ref = details_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
details_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string details = 4;
* @return The bytes for details.
*/
public com.google.protobuf.ByteString
getDetailsBytes() {
java.lang.Object ref = details_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
details_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string details = 4;
* @param value The details to set.
* @return This builder for chaining.
*/
public Builder setDetails(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
details_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string details = 4;
* @return This builder for chaining.
*/
public Builder clearDetails() {
details_ = getDefaultInstance().getDetails();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string details = 4;
* @param value The bytes for details to set.
* @return This builder for chaining.
*/
public Builder setDetailsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
details_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object resources_ = "";
/**
* string resources = 5;
* @return The resources.
*/
public java.lang.String getResources() {
java.lang.Object ref = resources_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resources_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string resources = 5;
* @return The bytes for resources.
*/
public com.google.protobuf.ByteString
getResourcesBytes() {
java.lang.Object ref = resources_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resources_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string resources = 5;
* @param value The resources to set.
* @return This builder for chaining.
*/
public Builder setResources(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resources_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string resources = 5;
* @return This builder for chaining.
*/
public Builder clearResources() {
resources_ = getDefaultInstance().getResources();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string resources = 5;
* @param value The bytes for resources to set.
* @return This builder for chaining.
*/
public Builder setResourcesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resources_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object ip_ = "";
/**
* string ip = 6;
* @return The ip.
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ip_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string ip = 6;
* @return The bytes for ip.
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string ip = 6;
* @param value The ip to set.
* @return This builder for chaining.
*/
public Builder setIp(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ip_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* string ip = 6;
* @return This builder for chaining.
*/
public Builder clearIp() {
ip_ = getDefaultInstance().getIp();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* string ip = 6;
* @param value The bytes for ip to set.
* @return This builder for chaining.
*/
public Builder setIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ip_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object agent_ = "";
/**
* string agent = 7;
* @return The agent.
*/
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
agent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string agent = 7;
* @return The bytes for agent.
*/
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string agent = 7;
* @param value The agent to set.
* @return This builder for chaining.
*/
public Builder setAgent(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
agent_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* string agent = 7;
* @return This builder for chaining.
*/
public Builder clearAgent() {
agent_ = getDefaultInstance().getAgent();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* string agent = 7;
* @param value The bytes for agent to set.
* @return This builder for chaining.
*/
public Builder setAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
agent_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object entry_ = "";
/**
* string entry = 8;
* @return The entry.
*/
public java.lang.String getEntry() {
java.lang.Object ref = entry_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entry_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string entry = 8;
* @return The bytes for entry.
*/
public com.google.protobuf.ByteString
getEntryBytes() {
java.lang.Object ref = entry_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
entry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string entry = 8;
* @param value The entry to set.
* @return This builder for chaining.
*/
public Builder setEntry(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
entry_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* string entry = 8;
* @return This builder for chaining.
*/
public Builder clearEntry() {
entry_ = getDefaultInstance().getEntry();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
* string entry = 8;
* @param value The bytes for entry to set.
* @return This builder for chaining.
*/
public Builder setEntryBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
entry_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private int level_ ;
/**
* int32 level = 9;
* @return The level.
*/
@java.lang.Override
public int getLevel() {
return level_;
}
/**
* int32 level = 9;
* @param value The level to set.
* @return This builder for chaining.
*/
public Builder setLevel(int value) {
level_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* int32 level = 9;
* @return This builder for chaining.
*/
public Builder clearLevel() {
bitField0_ = (bitField0_ & ~0x00000100);
level_ = 0;
onChanged();
return this;
}
private java.lang.Object appName_ = "";
/**
* string appName = 10;
* @return The appName.
*/
public java.lang.String getAppName() {
java.lang.Object ref = appName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
appName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string appName = 10;
* @return The bytes for appName.
*/
public com.google.protobuf.ByteString
getAppNameBytes() {
java.lang.Object ref = appName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string appName = 10;
* @param value The appName to set.
* @return This builder for chaining.
*/
public Builder setAppName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
appName_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* string appName = 10;
* @return This builder for chaining.
*/
public Builder clearAppName() {
appName_ = getDefaultInstance().getAppName();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
* string appName = 10;
* @param value The bytes for appName to set.
* @return This builder for chaining.
*/
public Builder setAppNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
appName_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:BusinessLogEvent)
}
// @@protoc_insertion_point(class_scope:BusinessLogEvent)
private static final org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent();
}
public static org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BusinessLogEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.beangle.ems.app.log.BusinessLogProto.BusinessLogEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_BusinessLogEvent_descriptor;
private static final
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_BusinessLogEvent_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n6src/main/resources/org/beangle/ems/app" +
"/log/model.proto\"\266\001\n\020BusinessLogEvent\022\020\n" +
"\010operator\030\001 \001(\t\022\021\n\toperateAt\030\002 \001(\003\022\017\n\007su" +
"mmary\030\003 \001(\t\022\017\n\007details\030\004 \001(\t\022\021\n\tresource" +
"s\030\005 \001(\t\022\n\n\002ip\030\006 \001(\t\022\r\n\005agent\030\007 \001(\t\022\r\n\005en" +
"try\030\010 \001(\t\022\r\n\005level\030\t \001(\005\022\017\n\007appName\030\n \001(" +
"\tB+\n\027org.beangle.ems.app.logB\020BusinessLo" +
"gProtob\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_BusinessLogEvent_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_BusinessLogEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_BusinessLogEvent_descriptor,
new java.lang.String[] { "Operator", "OperateAt", "Summary", "Details", "Resources", "Ip", "Agent", "Entry", "Level", "AppName", });
descriptor.resolveAllFeaturesImmutable();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy