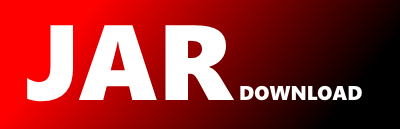
org.beangle.ems.security.helper.ProfileHelper Maven / Gradle / Ivy
/*
* Beangle, Agile Development Scaffold and Toolkit
*
* Copyright (c) 2005-2016, Beangle Software.
*
* Beangle is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Beangle is distributed in the hope that it will be useful.
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Beangle. If not, see .
*/
package org.beangle.ems.security.helper;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.beangle.commons.bean.PropertyUtils;
import org.beangle.commons.collection.CollectUtils;
import org.beangle.commons.dao.EntityDao;
import org.beangle.commons.lang.Strings;
import org.beangle.commons.lang.functor.Predicate;
import org.beangle.security.blueprint.Dimension;
import org.beangle.security.blueprint.Profile;
import org.beangle.security.blueprint.Property;
import org.beangle.security.blueprint.User;
import org.beangle.security.blueprint.service.ProfileService;
import org.beangle.security.blueprint.service.UserDataResolver;
import org.beangle.struts2.helper.ContextHelper;
import org.beangle.struts2.helper.Params;
public class ProfileHelper {
final EntityDao entityDao;
final ProfileService profileService;
UserDataResolver identifierDataResolver;
public ProfileHelper(EntityDao entityDao, ProfileService profileService) {
super();
this.entityDao = entityDao;
this.profileService = profileService;
}
/**
* 查看限制资源界面
*/
public void populateInfo(List extends Profile> profiles) {
Map> fieldMaps = CollectUtils.newHashMap();
for (Profile profile : profiles) {
Map aoDimensions = CollectUtils.newHashMap();
for (Property property : profile.getProperties()) {
String value = property.getValue();
String fieldName = property.getDimension().getName();
if (Strings.isNotEmpty(value)) {
if (null == property.getDimension().getSource()) {
aoDimensions.put(fieldName, value);
} else if (value.equals("*")) {
aoDimensions.put(fieldName, "不限");
} else {
aoDimensions.put(fieldName, profileService.getProperty(profile, property.getDimension()));
}
}
}
fieldMaps.put(PropertyUtils.getProperty(profile, "id").toString(), aoDimensions);
}
ContextHelper.put("profiles", profiles);
ContextHelper.put("fieldMaps", fieldMaps);
}
public void fillEditInfo(Profile profile, Long userId, boolean isAdmin) {
Map mngDimensions = CollectUtils.newHashMap();
Map aoDimensions = CollectUtils.newHashMap();
List myProfiles = entityDao.get(User.class, userId).getProfiles();
Set ignores = getIgnoreDimensions(myProfiles);
ContextHelper.put("ignoreDimensions", ignores);
Set holderIgnoreDimensions = CollectUtils.newHashSet();
ContextHelper.put("holderIgnoreDimensions", holderIgnoreDimensions);
List fields = entityDao.getAll(Dimension.class);
ContextHelper.put("fields", fields);
for (Dimension field : fields) {
List> mngDimensionValues = profileService.getDimensionValues(field);
if (!isAdmin) mngDimensionValues.retainAll(getMyProfileValues(myProfiles, field));
else ignores.add(field);
String fieldValue = "";
Property property = profile.getProperty(field);
if (null != property) fieldValue = property.getValue();
if ("*".equals(fieldValue)) holderIgnoreDimensions.add(field);
mngDimensions.put(field.getName(), mngDimensionValues);
if (null == field.getSource()) {
aoDimensions.put(field.getName(), fieldValue);
} else {
aoDimensions.put(field.getName(), profileService.getProperty(profile, field));
}
}
ContextHelper.put("mngDimensions", mngDimensions);
ContextHelper.put("aoDimensions", aoDimensions);
ContextHelper.put("profile", profile);
}
@SuppressWarnings("unchecked")
public void populateSaveInfo(Profile profile, Long userId, Boolean isAdmin) {
List myProfiles = entityDao.get(User.class, userId).getProfiles();
Set ignoreDimensions = getIgnoreDimensions(myProfiles);
for (final Dimension field : entityDao.getAll(Dimension.class)) {
String[] values = (String[]) Params.getAll(field.getName());
if ((ignoreDimensions.contains(field) || isAdmin)
&& Params.getBool("ignoreDimension" + field.getId())) {
profile.setProperty(field, "*");
} else {
if (null == values || values.length == 0) {
profile.setProperty(field, null);
} else {
String storedValue = null;
if (null != field.getKeyName()) {
final Set keys = CollectUtils.newHashSet(values);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy