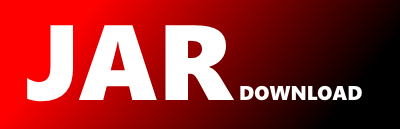
org.hibernate.IdentifierLoadAccess Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate;
import java.util.Optional;
import org.hibernate.graph.GraphSemantic;
import org.hibernate.graph.RootGraph;
/**
* Loads an entity by its primary identifier.
*
* @author Eric Dalquist
* @author Steve Ebersole
*/
public interface IdentifierLoadAccess {
/**
* Specify the {@link LockOptions} to use when retrieving the entity.
*
* @param lockOptions The lock options to use.
*
* @return {@code this}, for method chaining
*/
IdentifierLoadAccess with(LockOptions lockOptions);
/**
* Specify the {@link CacheMode} to use when retrieving the entity.
*
* @param cacheMode The CacheMode to use.
*
* @return {@code this}, for method chaining
*/
IdentifierLoadAccess with(CacheMode cacheMode);
/**
* Specify whether the entity should be loaded in read-only mode.
*
* @see Session#setDefaultReadOnly(boolean)
*/
IdentifierLoadAccess withReadOnly(boolean readOnly);
default IdentifierLoadAccess with(RootGraph graph) {
return with( graph, GraphSemantic.LOAD );
}
IdentifierLoadAccess with(RootGraph graph, GraphSemantic semantic);
/**
* Return the persistent instance with the given identifier, assuming that the instance exists. This method
* might return a proxied instance that is initialized on-demand, when a non-identifier method is accessed.
*
* You should not use this method to determine if an instance exists; to check for existence, use {@link #load}
* instead. Use this only to retrieve an instance that you assume exists, where non-existence would be an
* actual error.
*
* @param id The identifier for which to obtain a reference
*
* @return the persistent instance or proxy
*/
T getReference(Object id);
/**
* Return the persistent instance with the given identifier, or null if there is no such persistent instance.
* If the instance is already associated with the session, return that instance, initializing it if needed. This
* method never returns an uninitialized instance.
*
* @param id The identifier
*
* @return The persistent instance or {@code null}
*/
T load(Object id);
/**
* Same semantic as {@link #load} except that here {@link Optional} is returned to
* handle nullability.
*
* @param id The identifier
*
* @return The persistent instance, if one, wrapped in Optional
*/
Optional loadOptional(Object id);
}