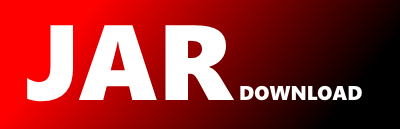
org.hibernate.annotations.FetchMode Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
/**
* Enumerates strategies for fetching an association from the database.
*
* The JPA-defined {@link jakarta.persistence.FetchType} enumerates the
* possibilities for when an association might be fetched. This
* annotation defines how it is fetched in terms of the actual
* SQL executed by the database.
*
* @author Steve Ebersole
* @author Emmanuel Bernard
*
* @see Fetch
* @see FetchProfile.FetchOverride#mode()
*/
public enum FetchMode {
/**
* Use a secondary select to load a single associated entity or
* collection, at some point after an initial query is executed.
*
* This is the default fetching strategy for any association
* or collection in Hibernate, unless the association or
* collection is {@linkplain jakarta.persistence.FetchType#EAGER
* explicitly marked for eager fetching}.
*
* This fetching strategy is vulnerable to the "N+1 selects"
* bugbear, though the impact may be alleviated somewhat via:
*
* - enabling batch fetching using {@link BatchSize}, or
*
- ensuring that the associated entity or collection may be
* retrieved from the {@linkplain Cache second-level cache}.
*
*
* This fetching strategy is, in principle, compatible with both
* {@linkplain jakarta.persistence.FetchType#EAGER eager} and
* {@linkplain jakarta.persistence.FetchType#LAZY lazy} fetching.
* On the other hand, performance considerations dictate that it
* should only be used in combination wih {@code EAGER} fetching
* when it is almost certain that the associated data will be
* available in the second-level cache.
*/
SELECT( org.hibernate.FetchMode.SELECT ),
/**
* Use an outer join to load all instances of the related entity
* or collection at once, as part of the execution of a query.
* No subsequent queries are executed.
*
* This is the default fetching strategy for an association or
* collection that is {@linkplain jakarta.persistence.FetchType#EAGER
* explicitly marked for eager fetching}.
*
* This fetching strategy is incompatible with
* {@linkplain jakarta.persistence.FetchType#LAZY lazy fetching}
* since the associated data is retrieved as part of the initial
* query.
*/
JOIN( org.hibernate.FetchMode.JOIN ),
/**
* Use a secondary select with a subselect that re-executes an
* initial query to load all instances of the related entity or
* collection at once, at some point after the initial query is
* executed. This fetching strategy is currently only available
* for collections and many-valued associations.
*
* This advanced fetching strategy is compatible with both
* {@linkplain jakarta.persistence.FetchType#EAGER eager} and
* {@linkplain jakarta.persistence.FetchType#LAZY lazy} fetching.
*
* Subselect fetching may be contrasted with {@linkplain BatchSize
* batch fetching}:
*
* - In batch fetching, a list of primary key values is sent to
* the database, bound within a SQL {@code in} condition.
*
- In subselect fetching, the primary keys are determined by
* re-execution of the initial query within a SQL subselect.
*
*/
SUBSELECT( org.hibernate.FetchMode.SELECT );
private final org.hibernate.FetchMode hibernateFetchMode;
FetchMode(org.hibernate.FetchMode hibernateFetchMode) {
this.hibernateFetchMode = hibernateFetchMode;
}
public org.hibernate.FetchMode getHibernateFetchMode() {
return hibernateFetchMode;
}
}