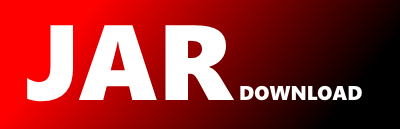
org.hibernate.annotations.Where Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.ElementType.TYPE;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
/**
* Specifies a restriction written in native SQL to add to the generated
* SQL for entities or collections.
*
* For example, {@code @Where} could be used to hide entity instances which
* have been soft-deleted, either for the entity class itself:
*
* @Entity
* @Where(clause = "status <> 'DELETED'")
* class Document {
* ...
* @Enumerated(STRING)
* Status status;
* ...
* }
*
*
* or, at the level of an association to the entity:
*
* @OneToMany(mappedBy = "owner")
* @Where(clause = "status <> 'DELETED'")
* List<Document> documents;
*
*
* The {@link WhereJoinTable} annotation lets a restriction be applied to
* an {@linkplain jakarta.persistence.JoinTable association table}:
*
* @ManyToMany
* @JoinTable(name = "collaborations")
* @Where(clause = "status <> 'DELETED'")
* @WhereJoinTable(clause = "status = 'ACTIVE'")
* List<Document> documents;
*
*
* By default, {@code @Where} restrictions declared for an entity are
* applied when loading associations of that entity type. This behavior can
* be disabled using the setting {@value org.hibernate.cfg.AvailableSettings#USE_ENTITY_WHERE_CLAUSE_FOR_COLLECTIONS};
* note, however, that setting is disabled.
*
* Note that {@code @Where} restrictions are always applied and cannot be
* disabled. Nor may they be parameterized. They're therefore much
* less flexible than {@linkplain Filter filters}.
*
* @see Filter
* @see DialectOverride.Where
* @see org.hibernate.cfg.AvailableSettings#USE_ENTITY_WHERE_CLAUSE_FOR_COLLECTIONS
* @see WhereJoinTable
*
* @author Emmanuel Bernard
*/
@Target({TYPE, METHOD, FIELD})
@Retention(RUNTIME)
public @interface Where {
/**
* A predicate, written in native SQL.
*/
String clause();
}