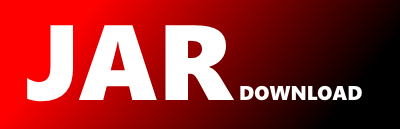
org.hibernate.internal.NaturalIdMultiLoadAccessStandard Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.internal;
import java.util.List;
import java.util.Set;
import org.hibernate.CacheMode;
import org.hibernate.LockOptions;
import org.hibernate.NaturalIdMultiLoadAccess;
import org.hibernate.engine.spi.EffectiveEntityGraph;
import org.hibernate.engine.spi.LoadQueryInfluencers;
import org.hibernate.graph.GraphSemantic;
import org.hibernate.graph.RootGraph;
import org.hibernate.graph.spi.RootGraphImplementor;
import org.hibernate.internal.util.collections.CollectionHelper;
import org.hibernate.loader.ast.spi.MultiNaturalIdLoadOptions;
import org.hibernate.persister.entity.EntityPersister;
/**
* @author Steve Ebersole
*/
public class NaturalIdMultiLoadAccessStandard implements NaturalIdMultiLoadAccess, MultiNaturalIdLoadOptions {
private final EntityPersister entityDescriptor;
private final SessionImpl session;
private LockOptions lockOptions;
private CacheMode cacheMode;
private RootGraphImplementor rootGraph;
private GraphSemantic graphSemantic;
private Integer batchSize;
private boolean returnOfDeletedEntitiesEnabled;
private boolean orderedReturnEnabled = true;
public NaturalIdMultiLoadAccessStandard(EntityPersister entityDescriptor, SessionImpl session) {
this.entityDescriptor = entityDescriptor;
this.session = session;
}
@Override
public NaturalIdMultiLoadAccess with(LockOptions lockOptions) {
this.lockOptions = lockOptions;
return this;
}
@Override
public NaturalIdMultiLoadAccess with(CacheMode cacheMode) {
this.cacheMode = cacheMode;
return this;
}
@Override
public NaturalIdMultiLoadAccess with(RootGraph graph, GraphSemantic semantic) {
this.rootGraph = (RootGraphImplementor) graph;
this.graphSemantic = semantic;
return this;
}
@Override
public NaturalIdMultiLoadAccess withBatchSize(int batchSize) {
this.batchSize = batchSize;
return this;
}
@Override
public NaturalIdMultiLoadAccess enableReturnOfDeletedEntities(boolean enabled) {
returnOfDeletedEntitiesEnabled = enabled;
return this;
}
@Override
public NaturalIdMultiLoadAccess enableOrderedReturn(boolean enabled) {
orderedReturnEnabled = enabled;
return this;
}
@Override
@SuppressWarnings( "unchecked" )
public List multiLoad(Object... ids) {
final CacheMode sessionCacheMode = session.getCacheMode();
boolean cacheModeChanged = false;
if ( cacheMode != null ) {
// naive check for now...
// todo : account for "conceptually equal"
if ( cacheMode != sessionCacheMode ) {
session.setCacheMode( cacheMode );
cacheModeChanged = true;
}
}
session.autoFlushIfRequired( (Set) CollectionHelper.setOf( entityDescriptor.getQuerySpaces() ) );
final LoadQueryInfluencers loadQueryInfluencers = session.getLoadQueryInfluencers();
try {
final EffectiveEntityGraph effectiveEntityGraph = loadQueryInfluencers.getEffectiveEntityGraph();
final GraphSemantic initialGraphSemantic = effectiveEntityGraph.getSemantic();
final RootGraphImplementor> initialGraph = effectiveEntityGraph.getGraph();
final boolean hadInitialGraph = initialGraphSemantic != null;
if ( graphSemantic != null ) {
if ( rootGraph == null ) {
throw new IllegalArgumentException( "Graph semantic specified, but no RootGraph was supplied" );
}
effectiveEntityGraph.applyGraph( rootGraph, graphSemantic );
}
try {
return (List) entityDescriptor.getMultiNaturalIdLoader().multiLoad( ids, this, session );
}
finally {
if ( graphSemantic != null ) {
if ( hadInitialGraph ) {
effectiveEntityGraph.applyGraph( initialGraph, initialGraphSemantic );
}
else {
effectiveEntityGraph.clear();
}
}
}
}
finally {
if ( cacheModeChanged ) {
// change it back
session.setCacheMode( sessionCacheMode );
}
}
}
@Override
public List multiLoad(List> ids) {
return multiLoad( ids.toArray( new Object[ 0 ] ) );
}
@Override
public boolean isReturnOfDeletedEntitiesEnabled() {
return returnOfDeletedEntitiesEnabled;
}
@Override
public boolean isOrderReturnEnabled() {
return orderedReturnEnabled;
}
@Override
public LockOptions getLockOptions() {
return lockOptions;
}
@Override
public Integer getBatchSize() {
return batchSize;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy