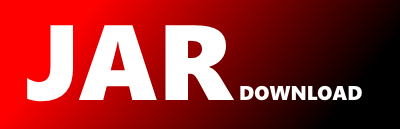
org.hibernate.query.Query Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.query;
import java.time.Instant;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Stream;
import org.hibernate.CacheMode;
import org.hibernate.FlushMode;
import org.hibernate.Incubating;
import org.hibernate.LockMode;
import org.hibernate.LockOptions;
import org.hibernate.NonUniqueResultException;
import org.hibernate.ScrollMode;
import org.hibernate.ScrollableResults;
import org.hibernate.SharedSessionContract;
import org.hibernate.dialect.Dialect;
import org.hibernate.graph.GraphSemantic;
import org.hibernate.graph.RootGraph;
import org.hibernate.query.spi.QueryOptions;
import org.hibernate.transform.ResultTransformer;
import jakarta.persistence.CacheRetrieveMode;
import jakarta.persistence.CacheStoreMode;
import jakarta.persistence.FlushModeType;
import jakarta.persistence.LockModeType;
import jakarta.persistence.Parameter;
import jakarta.persistence.TemporalType;
import jakarta.persistence.TypedQuery;
/**
* Within the context of an active {@linkplain org.hibernate.Session session},
* an instance of this type represents an executable query, either:
*
* - a query written in HQL,
*
- a named query written in HQL or native SQL, or
*
- a {@linkplain jakarta.persistence.criteria.CriteriaBuilder criteria query}.
*
*
* The subtype {@link NativeQuery} represents a query written in native SQL.
*
* This type simply mixes the {@link TypedQuery} interface defined by JPA with
* {@link SelectionQuery} and {@link MutationQuery}. Unfortunately, JPA does
* not distinguish between {@linkplain SelectionQuery selection queries} and
* {@linkplain MutationQuery mutation queries}, so we lose that distinction here.
* However, every {@code Query} may logically be classified as one or the other.
*
* A {@code Query} may be obtained from the {@link org.hibernate.Session} by
* calling:
*
* - {@link QueryProducer#createQuery(String, Class)}, passing the HQL as a
* string,
*
- {@link QueryProducer#createQuery(jakarta.persistence.criteria.CriteriaQuery)},
* passing a {@linkplain jakarta.persistence.criteria.CriteriaQuery criteria
* object}, or
*
- {@link QueryProducer#createNamedQuery(String, Class)} passing the name
* of a query defined using {@link jakarta.persistence.NamedQuery} or
* {@link jakarta.persistence.NamedNativeQuery}.
*
*
* A {@code Query} controls how a query is executed, and allows arguments to be
* bound to its parameters.
*
* - Selection queries are usually executed using {@link #getResultList()} or
* {@link #getSingleResult()}.
*
- The methods {@link #setMaxResults(int)} and {@link #setFirstResult(int)}
* control limits and pagination.
*
- The various overloads of {@link #setParameter(String, Object)} and
* {@link #setParameter(int, Object)} allow arguments to be bound to named
* and ordinal parameters defined by the query.
*
*
* Note that this interface offers no real advantages over {@link SelectionQuery}
* except for compatibility with the JPA-defined {@link TypedQuery} interface.
*
* @author Gavin King
* @author Steve Ebersole
*
* @param The result type, for typed queries, or {@link Object} for untyped queries
*
* @see QueryProducer
*/
@Incubating
public interface Query extends SelectionQuery, MutationQuery, TypedQuery {
/**
* Execute the query and return the query results as a {@link List}.
* If the query contains multiple items in the selection list, then
* by default each result in the list is packaged in an array of type
* {@code Object[]}.
*
* @return the result list
*/
@Override
List list();
/**
* Execute the query and return the query results as a {@link List}.
* If the query contains multiple items in the selection list, then
* by default each result in the list is packaged in an array of type
* {@code Object[]}.
*
* @implNote Delegates to {@link #list()}
*
* @return the results as a list
*/
@Override
default List getResultList() {
return list();
}
/**
* Execute the query and return the results in a
* {@linkplain ScrollableResults scrollable form}.
*
* This overload simply calls {@link #scroll(ScrollMode)} using the
* {@linkplain Dialect#defaultScrollMode() dialect default scroll mode}.
*
* @apiNote The exact behavior of this method depends somewhat
* on the level of JDBC driver support for scrollable
* {@link java.sql.ResultSet}s, and so is not very
* portable between database.
*/
@Override
ScrollableResults scroll();
/**
* Execute the query and return the results in a
* {@linkplain ScrollableResults scrollable form}. The capabilities
* of the returned {@link ScrollableResults} depend on the specified
* {@link ScrollMode}.
*
* @apiNote The exact behavior of this method depends somewhat
* on the level of JDBC driver support for scrollable
* {@link java.sql.ResultSet}s, and so is not very
* portable between database.
*/
@Override
ScrollableResults scroll(ScrollMode scrollMode);
/**
* Execute the query and return the query results as a {@link Stream}.
* If the query contains multiple items in the selection list, then
* by default each result in the stream is packaged in an array of
* type {@code Object[]}.
*
* The client should call {@link Stream#close()} after processing the
* stream so that resources are freed as soon as possible.
*
* @implNote Delegates to {@link #stream()}, which in turn delegates
* to this method. Implementors should implement at least
* one of these methods.
*
* @return The results as a {@link Stream}
*/
@Override
default Stream getResultStream() {
return stream();
}
/**
* Execute the query and return the query results as a {@link Stream}.
* If the query contains multiple items in the selection list, then
* by default each result in the stream is packaged in an array of type
* {@code Object[]}.
*
* The client should call {@link Stream#close()} after processing the
* stream so that resources are freed as soon as possible.
*
* @return The results as a {@link Stream}
*
* @since 5.2
*/
@Override
default Stream stream() {
return getResultStream();
}
/**
* Execute the query and return the single result of the query, or
* {@code null} if the query returns no results.
*
* @return the single result or {@code null} if there is no result to return
*
* @throws NonUniqueResultException if there is more than one matching result
*/
@Override
R uniqueResult();
/**
* Execute the query and return the single result of the query,
* throwing an exception if the query returns no results.
*
* @return the single result, only if there is exactly one
*
* @throws jakarta.persistence.NonUniqueResultException if there is more than one matching result
* @throws jakarta.persistence.NoResultException if there is no result to return
*/
@Override
R getSingleResult();
/**
* Execute the query and return the single result of the query as
* an instance of {@link Optional}.
*
* @return the single result as an {@code Optional}
*
* @throws NonUniqueResultException if there is more than one matching result
*/
@Override
Optional uniqueResultOptional();
/**
* Execute an insert, update, or delete statement, and return the
* number of affected entities.
*
* For use with instances of {@link MutationQuery} created using
* {@link QueryProducer#createMutationQuery(String)},
* {@link QueryProducer#createNamedMutationQuery(String)},
* {@link QueryProducer#createNativeMutationQuery(String)},
* {@link QueryProducer#createQuery(jakarta.persistence.criteria.CriteriaUpdate)}, or
* {@link QueryProducer#createQuery(jakarta.persistence.criteria.CriteriaDelete)}.
*
* @return the number of affected entity instances
* (may differ from the number of affected rows)
*
* @see QueryProducer#createMutationQuery
* @see QueryProducer#createMutationQuery(String)
* @see QueryProducer#createNamedMutationQuery(String)
* @see QueryProducer#createNativeMutationQuery(String)
*
* @see jakarta.persistence.Query#executeUpdate()
*
* @apiNote This method is needed because this interface extends
* {@link jakarta.persistence.Query}, which defines this method.
* See {@link MutationQuery} and {@link SelectionQuery}.
*
* @see QueryProducer#createMutationQuery
*/
@Override
int executeUpdate();
/**
* Get the {@link QueryProducer} which produced this {@code Query},
* that is, the {@link org.hibernate.Session} or
* {@link org.hibernate.StatelessSession} that was used to create
* this {@code Query} instance.
*
* @return The producer of this query
*/
SharedSessionContract getSession();
/**
* The query as a string, or {@code null} in the case of a criteria query.
*/
String getQueryString();
/**
* Apply the given graph using the given semantic
*
* @param graph The graph to apply.
* @param semantic The semantic to use when applying the graph
*/
Query applyGraph(@SuppressWarnings("rawtypes") RootGraph graph, GraphSemantic semantic);
/**
* Apply the given graph using {@linkplain GraphSemantic#FETCH fetch semantics}.
*
* @apiNote This method calls {@link #applyGraph(RootGraph, GraphSemantic)}
* using {@link GraphSemantic#FETCH} as the semantic.
*/
default Query applyFetchGraph(@SuppressWarnings("rawtypes") RootGraph graph) {
return applyGraph( graph, GraphSemantic.FETCH );
}
/**
* Apply the given graph using {@linkplain GraphSemantic#LOAD load semantics}.
*
* @apiNote This method calls {@link #applyGraph(RootGraph, GraphSemantic)}
* using {@link GraphSemantic#LOAD} as the semantic.
*/
@SuppressWarnings("UnusedDeclaration")
default Query applyLoadGraph(@SuppressWarnings("rawtypes") RootGraph graph) {
return applyGraph( graph, GraphSemantic.LOAD );
}
/**
* Obtain the comment currently associated with this query.
*
* If SQL commenting is enabled, the comment will be added to the SQL
* query sent to the database, which may be useful for identifying the
* source of troublesome queries.
*
* SQL commenting may be enabled using the configuration property
* {@value org.hibernate.cfg.AvailableSettings#USE_SQL_COMMENTS}.
*
* @return The comment.
*/
String getComment();
/**
* Set the comment for this query.
*
* If SQL commenting is enabled, the comment will be added to the SQL
* query sent to the database, which may be useful for identifying the
* source of troublesome queries.
*
* SQL commenting may be enabled using the configuration property
* {@value org.hibernate.cfg.AvailableSettings#USE_SQL_COMMENTS}.
*
* @param comment The human-readable comment
*
* @return {@code this}, for method chaining
*
* @see #getComment()
*/
Query setComment(String comment);
/**
* Add a database query hint to the SQL query.
*
* A database hint is a completely different concept to a JPA hint
* specified using {@link jakarta.persistence.QueryHint} or
* {@link #getHints()}. These are hints to the JPA provider.
*
* Multiple query hints may be specified. The operation
* {@link Dialect#getQueryHintString(String, List)} determines how
* the hint is actually added to the SQL query.
*
* @param hint The database specific query hint to add.
*/
Query addQueryHint(String hint);
/**
* Obtains the {@link LockOptions} in effect for this query.
*
* @return The {@link LockOptions} currently in effect
*
* @see LockOptions
*/
@Override
LockOptions getLockOptions();
/**
* Apply the given {@linkplain LockOptions lock options} to this
* query. Alias-specific lock modes in the given lock options are
* merged with any alias-specific lock mode which have already been
* {@linkplain #setAliasSpecificLockMode(String, LockMode) set}. If
* a lock mode has already been specified for an alias that is among
* the aliases in the given lock options, the lock mode specified in
* the given lock options overrides the lock mode that was already
* set.
*
* @param lockOptions The lock options to apply to the query.
*
* @return {@code this}, for method chaining
*
* @see #getLockOptions()
*/
Query setLockOptions(LockOptions lockOptions);
/**
* Set the {@link LockMode} to use for particular alias defined in
* the {@code FROM} clause of the query.
*
* The alias-specific lock modes specified here are added to the
* {@link #getLockOptions() LockOption}s.
*
* The effect of alias-specific locking is quite dependent on the
* driver and database. For maximum portability, the given lock
* mode should be {@link LockMode#PESSIMISTIC_WRITE}.
*
* @param alias A query alias
* @param lockMode The lock mode to apply
*
* @return {@code this}, for method chaining
*
* @see #getLockOptions()
*/
@Override
Query setLockMode(String alias, LockMode lockMode);
/**
* Set a {@link TupleTransformer}.
*/
Query setTupleTransformer(TupleTransformer transformer);
/**
* Set a {@link ResultListTransformer}.
*/
Query setResultListTransformer(ResultListTransformer transformer);
/**
* Get the execution options for this {@code Query}. Many of the setters
* of this object update the state of the returned {@link QueryOptions}.
* This is useful because it gives access to s primitive value in its
* (nullable) wrapper form, rather than the primitive form as required
* by JPA. This allows us to distinguish whether a value has been
* explicitly set by the client.
*
* @return Return the encapsulation of this query's options.
*/
QueryOptions getQueryOptions();
/**
* Access to information about query parameters.
*
* @return information about query parameters.
*/
ParameterMetadata getParameterMetadata();
/**
* Bind the given argument to a named query parameter.
*
* If the type of the parameter cannot be inferred from the context
* in which it occurs, use one of the forms accept a "type".
*
* @see #setParameter(String, Object, Class)
* @see #setParameter(String, Object, BindableType)
*/
@Override
Query setParameter(String parameter, Object argument);
/**
* Bind the given argument to a named query parameter using the given
* Class reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameter(String, Object)} is used.
*
* @see #setParameter(String, Object, BindableType)
*/
Query setParameter(String parameter, P argument, Class type);
/**
* Bind the given argument to a named query parameter using the given
* {@link BindableType}.
*/
Query setParameter(String parameter, P argument, BindableType type);
/**
* Bind an {@link Instant} value to the named query parameter using
* just the portion indicated by the given {@link TemporalType}.
*/
Query setParameter(String parameter, Instant argument, TemporalType temporalType);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(String parameter, Calendar argument, TemporalType temporalType);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(String parameter, Date argument, TemporalType temporalType);
/**
* Bind the given argument to an ordinal query parameter.
*
* If the type of the parameter cannot be inferred from the context in
* which it occurs, use one of the forms which accepts a "type".
*
* @see #setParameter(int, Object, Class)
* @see #setParameter(int, Object, BindableType)
*/
@Override
Query setParameter(int parameter, Object argument);
/**
* Bind the given argument to an ordinal query parameter using the given
* Class reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameter(int, Object)} is used.
*
* @see #setParameter(int, Object, BindableType)
*/
Query setParameter(int parameter, P argument, Class type);
/**
* Bind the given argument to an ordinal query parameter using the given
* {@link BindableType}.
*/
Query setParameter(int parameter, P argument, BindableType type);
/**
* Bind an {@link Instant} value to the ordinal query parameter using
* just the portion indicated by the given {@link TemporalType}.
*/
Query setParameter(int parameter, Instant argument, TemporalType temporalType);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(int parameter, Date argument, TemporalType temporalType);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(int parameter, Calendar argument, TemporalType temporalType);
/**
* Bind an argument to the query parameter represented by the given
* {@link QueryParameter}.
*
* If the type of the parameter cannot be inferred from the context in
* which it occurs, use one of the forms which accepts a "type".
*
* @see #setParameter(QueryParameter, Object, BindableType)
*
* @param parameter the query parameter memento
* @param argument the argument, which might be null
*
* @return {@code this}, for method chaining
*/
Query setParameter(QueryParameter parameter, T argument);
/**
* Bind an argument to the query parameter represented by the given
* {@link QueryParameter} using the given Class reference to attempt to
* determine the {@link BindableType} to use. If unable to determine
* an appropriate {@link BindableType}, {@link #setParameter(QueryParameter, Object)} is used
*
* @param parameter the query parameter memento
* @param argument the argument, which might be null
* @param type a {@link BindableType} representing the type of the parameter
*
* @return {@code this}, for method chaining
*
* @see #setParameter(QueryParameter, Object, BindableType)
*/
Query setParameter(QueryParameter parameter, P argument, Class
type);
/**
* Bind an argument to the query parameter represented by the given
* {@link QueryParameter} using the given {@link BindableType}.
*
* @param parameter the query parameter memento
* @param argument the argument, which might be null
* @param type an {@link BindableType} representing the type of the parameter
*
* @return {@code this}, for method chaining
*/
Query setParameter(QueryParameter parameter, P argument, BindableType
type);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(Parameter parameter, T argument);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(Parameter parameter, Calendar argument, TemporalType temporalType);
/**
* {@link jakarta.persistence.Query} override
*/
@Override
Query setParameter(Parameter parameter, Date argument, TemporalType temporalType);
/**
* Bind multiple arguments to a named query parameter.
*
* The "type mapping" for the binding is inferred from the type of
* the first collection element.
*
* @see #setParameterList(java.lang.String, java.util.Collection, BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, @SuppressWarnings("rawtypes") Collection arguments);
/**
* Bind multiple arguments to a named query parameter using the given
* Class reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameterList(String, Collection)} is used.
*
* @see #setParameterList(java.lang.String, java.util.Collection, BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, Collection extends P> arguments, Class javaType);
/**
* Bind multiple arguments to a named query parameter using the given
* {@link BindableType}.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, Collection extends P> arguments, BindableType type);
/**
* Bind multiple arguments to a named query parameter.
*
* The "type mapping" for the binding is inferred from the type of
* the first collection element.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, Object[] values);
/**
* Bind multiple arguments to a named query parameter using the given
* Class reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameterList(String, Collection)} is used.
*
* @see #setParameterList(java.lang.String, Object[], BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, P[] arguments, Class javaType);
/**
* Bind multiple arguments to a named query parameter using the given
* {@link BindableType}.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(String parameter, P[] arguments, BindableType type);
/**
* Bind multiple arguments to an ordinal query parameter.
*
* The "type mapping" for the binding is inferred from the type of
* the first collection element.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, @SuppressWarnings("rawtypes") Collection arguments);
/**
* Bind multiple arguments to an ordinal query parameter using the given
* Class reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameterList(String, Collection)} is used.
*
* @see #setParameterList(int, Collection, BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, Collection extends P> arguments, Class javaType);
/**
* Bind multiple arguments to an ordinal query parameter using the given
* {@link BindableType}.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, Collection extends P> arguments, BindableType type);
/**
* Bind multiple arguments to an ordinal query parameter.
*
* The "type mapping" for the binding is inferred from the type of the
* first collection element.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, Object[] arguments);
/**
* Bind multiple arguments to an ordinal query parameter using the given
* {@link Class} reference to attempt to determine the {@link BindableType}
* to use. If unable to determine an appropriate {@link BindableType},
* {@link #setParameterList(String, Collection)} is used.
*
* @see #setParameterList(int, Object[], BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, P[] arguments, Class javaType);
/**
* Bind multiple arguments to an ordinal query parameter using the given
* {@link BindableType}.
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(int parameter, P[] arguments, BindableType type);
/**
* Bind multiple arguments to the query parameter represented by the given
* {@link QueryParameter}.
*
* The type of the parameter is inferred from the context in which it occurs,
* and from the type of the first given argument.
*
* @param parameter the parameter memento
* @param arguments a collection of arguments
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, Collection extends P> arguments);
/**
* Bind multiple arguments to the query parameter represented by the given
* {@link QueryParameter} using the given Class reference to attempt to
* determine the {@link BindableType} to use. If unable to determine an
* appropriate {@link BindableType}, {@link #setParameterList(String, Collection)}
* is used.
*
* @see #setParameterList(QueryParameter, java.util.Collection, BindableType)
*
* @apiNote This is used for binding a list of values to an expression such
* as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, Collection extends P> arguments, Class
javaType);
/**
* Bind multiple arguments to the query parameter represented by the given
* {@link QueryParameter}, inferring the {@link BindableType}.
*
* The "type mapping" for the binding is inferred from the type of the first
* collection element.
*
* @apiNote This is used for binding a list of values to an expression such
* as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, Collection extends P> arguments, BindableType
type);
/**
* Bind multiple arguments to the query parameter represented by the
* given {@link QueryParameter}.
*
* The type of the parameter is inferred between the context in which it
* occurs, the type associated with the QueryParameter and the type of
* the first given argument.
*
* @param parameter the parameter memento
* @param arguments a collection of arguments
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, P[] arguments);
/**
* Bind multiple arguments to the query parameter represented by the
* given {@link QueryParameter} using the given {@code Class} reference
* to attempt to determine the {@link BindableType} to use. If unable to
* determine an appropriate {@link BindableType},
* {@link #setParameterList(String, Collection)} is used.
*
* @see #setParameterList(QueryParameter, Object[], BindableType)
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, P[] arguments, Class
javaType);
/**
* Bind multiple arguments to the query parameter represented by the
* given {@link QueryParameter}, inferring the {@link BindableType}.
*
* The "type mapping" for the binding is inferred from the type of
* the first collection element
*
* @apiNote This is used for binding a list of values to an expression
* such as {@code entity.field in (:values)}.
*
* @return {@code this}, for method chaining
*/
Query setParameterList(QueryParameter parameter, P[] arguments, BindableType
type);
/**
* Bind the property values of the given bean to named parameters of the query,
* matching property names with parameter names and mapping property types to
* Hibernate types using heuristics.
*
* @param bean any JavaBean or POJO
*
* @return {@code this}, for method chaining
*/
Query setProperties(Object bean);
/**
* Bind the values of the given {@code Map} for each named parameters of
* the query, matching key names with parameter names and mapping value
* types to Hibernate types using heuristics.
*
* @param bean a {@link Map} of names to arguments
*
* @return {@code this}, for method chaining
*/
Query setProperties(@SuppressWarnings("rawtypes") Map bean);
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// covariant overrides - CommonQueryContract
@Override
Query setHibernateFlushMode(FlushMode flushMode);
@Override
Query setCacheable(boolean cacheable);
@Override
Query setCacheRegion(String cacheRegion);
@Override
Query setCacheMode(CacheMode cacheMode);
@Override
Query setCacheStoreMode(CacheStoreMode cacheStoreMode);
@Override
Query setCacheRetrieveMode(CacheRetrieveMode cacheRetrieveMode);
@Override
Query setTimeout(int timeout);
@Override
Query setFetchSize(int fetchSize);
@Override
Query setReadOnly(boolean readOnly);
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// covariant overrides - jakarta.persistence.Query/TypedQuery
@Override
Query setMaxResults(int maxResult);
@Override
Query setFirstResult(int startPosition);
@Override
Query setHint(String hintName, Object value);
@Override
Query setFlushMode(FlushModeType flushMode);
@Override
Query setLockMode(LockModeType lockMode);
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// deprecated methods
/**
* @deprecated Use {@link #setTupleTransformer} or {@link #setResultListTransformer}
*/
@Deprecated(since = "5.2")
default Query setResultTransformer(ResultTransformer transformer) {
return setTupleTransformer( transformer ).setResultListTransformer( transformer );
}
}