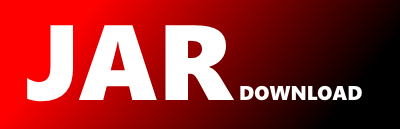
org.hibernate.annotations.ManyToAny Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import jakarta.persistence.FetchType;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
/**
* Maps a to-many cardinality association taking values over several
* entity types which are not related by the usual entity
* inheritance, using a discriminator value stored in an
* {@linkplain jakarta.persistence.JoinTable association table}.
*
* The annotated property should be of type {@link java.util.List},
* {@link java.util.Set}, {@link java.util.Collection}, or
* {@link java.util.Map}, and the elements of the collection must be
* entities.
*
* For example:
*
* @ManyToAny
* @Column(name = "property_type")
* @AnyKeyJavaClass(Long.class)
* @AnyDiscriminatorValue(discriminator = "S", entity = StringProperty.class)
* @AnyDiscriminatorValue(discriminator = "I", entity = IntegerProperty.class)
* @JoinTable(name = "repository_properties",
* joinColumns = @JoinColumn(name = "repository_id"),
* inverseJoinColumns = @JoinColumn(name = "property_id"))
* @Cascade(PERSIST)
* private List<Property<?>> properties = new ArrayList<>();
*
*
* In this example, {@code Property} is not required to be an entity type,
* it might even just be an interface, but its subtypes {@code StringProperty}
* and {@code IntegerProperty} must be entity classes with the same identifier
* type.
*
* This is just the many-valued form of {@link Any}, and the mapping
* options are similar, except that the
* {@link jakarta.persistence.JoinTable @JoinTable} annotation is used
* to specify the association table.
*
* - {@link AnyDiscriminator}, {@link JdbcType}, or {@link JdbcTypeCode}
* specifies the type of the discriminator,
*
- {@link AnyDiscriminatorValue} specifies how discriminator values
* map to entity types.
*
- {@link AnyKeyJavaType}, {@link AnyKeyJavaClass}, {@link AnyKeyJdbcType},
* or {@link AnyKeyJdbcTypeCode} specifies the type of the foreign key.
*
- {@link jakarta.persistence.JoinTable} specifies the name of the
* association table and its foreign key columns.
*
- {@link jakarta.persistence.Column} specifies the column of the
* association table in which the discriminator value is stored.
*
*
* @see Any
*
* @author Emmanuel Bernard
* @author Steve Ebersole
*/
@Target({METHOD, FIELD})
@Retention(RUNTIME)
public @interface ManyToAny {
/**
* Specifies whether the value of the field or property should be fetched
* lazily or eagerly:
*
* - {@link FetchType#EAGER}, the default, requires that the association
* be fetched immediately, but
*
- {@link FetchType#LAZY} is a hint which has no effect unless bytecode
* enhancement is enabled.
*
*/
FetchType fetch() default FetchType.EAGER;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy