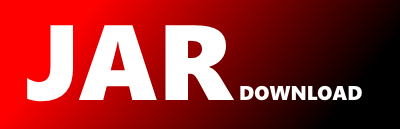
org.hibernate.annotations.SQLInsert Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.ElementType.TYPE;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
/**
* Specifies a custom SQL DML statement to be used in place of the default SQL
* generated by Hibernate when an entity or collection row is inserted in the
* database.
*
* The given {@linkplain #sql SQL statement} must have exactly the number of JDBC
* {@code ?} parameters that Hibernate expects, that is, one for each column
* mapped by the entity, in the exact order Hibernate expects. In particular,
* the {@linkplain jakarta.persistence.Id primary key} columns must come last.
*
* If a column should not be written as part of the insert statement,
* and has no corresponding JDBC parameter in the custom SQL, it must be mapped
* using {@link jakarta.persistence.Column#insertable insertable=false}.
*
* A custom SQL insert statement might assign a value to a mapped column as it
* is written. In this case, the corresponding property of the entity remains
* unassigned after the insert is executed unless
* {@link Generated @Generated} is specified, forcing Hibernate to reread the
* state of the entity after each insert.
*
* Similarly, a custom insert statement might transform a mapped column value
* as it is written. In this case, the state of the entity held in memory
* loses synchronization with the database after the insert is executed unless
* {@link Generated @Generated(writable=true)} is specified, again forcing
* Hibernate to reread the state of the entity after each insert.
*
* @author Laszlo Benke
*/
@Target({TYPE, FIELD, METHOD})
@Retention(RUNTIME)
@Repeatable(SQLInserts.class)
public @interface SQLInsert {
/**
* Procedure name or SQL {@code INSERT} statement.
*/
String sql();
/**
* Is the statement callable (aka a {@link java.sql.CallableStatement})?
*/
boolean callable() default false;
/**
* For persistence operation what style of determining results (success/failure) is to be used.
*/
ResultCheckStyle check() default ResultCheckStyle.NONE;
/**
* The name of the table in the case of an entity with {@link jakarta.persistence.SecondaryTable
* secondary tables}, defaults to the primary table.
*
* @return the name of the secondary table
*
* @since 6.2
*/
String table() default "";
}