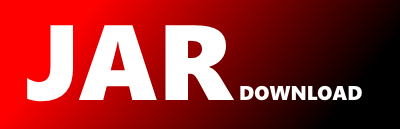
org.hibernate.graph.RootGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.graph;
import java.util.List;
import jakarta.persistence.AttributeNode;
import jakarta.persistence.EntityGraph;
import jakarta.persistence.Subgraph;
import jakarta.persistence.metamodel.Attribute;
import org.hibernate.metamodel.model.domain.PersistentAttribute;
/**
* Extends the JPA-defined {@link EntityGraph} with additional operations.
*
* @author Steve Ebersole
* @author Andrea Boriero
*
* @see SubGraph
*/
public interface RootGraph extends Graph, EntityGraph {
@Override
RootGraph makeRootGraph(String name, boolean mutable);
SubGraph makeSubGraph(boolean mutable);
@Override
SubGraph extends T1> addSubclassSubgraph(Class extends T1> type);
@Override
@SuppressWarnings({"unchecked", "rawtypes"})
default List> getAttributeNodes() {
return (List) getAttributeNodeList();
}
@Override
default void addAttributeNodes(String... names) {
if ( names != null ) {
for ( String name : names ) {
addAttributeNode( name );
}
}
}
@Override
@SuppressWarnings("unchecked")
default void addAttributeNodes(Attribute... attributes) {
if ( attributes != null ) {
for ( Attribute attribute : attributes ) {
addAttributeNode( (PersistentAttribute) attribute );
}
}
}
@Override
default SubGraph addSubgraph(Attribute attribute) {
return addSubGraph( (PersistentAttribute) attribute );
}
@Override
default SubGraph extends X> addSubgraph(Attribute attribute, Class extends X> type) {
return addSubGraph( (PersistentAttribute) attribute, type );
}
@Override
default SubGraph addSubgraph(String name) {
return addSubGraph( name );
}
@Override
default SubGraph addSubgraph(String name, Class type) {
return addSubGraph( name, type );
}
@Override
default SubGraph addKeySubgraph(Attribute attribute) {
return addKeySubGraph( (PersistentAttribute) attribute );
}
@Override
default SubGraph extends X> addKeySubgraph(Attribute attribute, Class extends X> type) {
return addKeySubGraph( (PersistentAttribute) attribute, type );
}
@Override
default SubGraph addKeySubgraph(String name) {
return addKeySubGraph( name );
}
@Override
default Subgraph addKeySubgraph(String name, Class type) {
return addKeySubGraph( name, type );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy