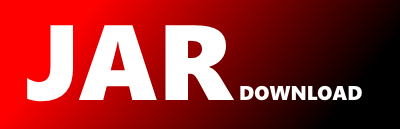
org.hibernate.mapping.MappingHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.mapping;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.function.Supplier;
import org.hibernate.Internal;
import org.hibernate.MappingException;
import org.hibernate.boot.BootLogging;
import org.hibernate.boot.model.internal.DelayedParameterizedTypeBean;
import org.hibernate.boot.registry.classloading.spi.ClassLoaderService;
import org.hibernate.boot.spi.MetadataBuildingContext;
import org.hibernate.boot.spi.MetadataImplementor;
import org.hibernate.internal.util.collections.CollectionHelper;
import org.hibernate.resource.beans.internal.FallbackBeanInstanceProducer;
import org.hibernate.resource.beans.spi.ManagedBean;
import org.hibernate.resource.beans.spi.ManagedBeanRegistry;
import org.hibernate.resource.beans.spi.ProvidedInstanceManagedBeanImpl;
import org.hibernate.type.AnyType;
import org.hibernate.type.CollectionType;
import org.hibernate.type.CustomCollectionType;
import org.hibernate.type.ForeignKeyDirection;
import org.hibernate.type.ManyToOneType;
import org.hibernate.type.MetaType;
import org.hibernate.type.OneToOneType;
import org.hibernate.type.SpecialOneToOneType;
import org.hibernate.type.Type;
import org.hibernate.usertype.ParameterizedType;
import org.hibernate.usertype.UserCollectionType;
import static org.hibernate.metamodel.mapping.MappingModelCreationLogging.MAPPING_MODEL_CREATION_MESSAGE_LOGGER;
/**
* @author Steve Ebersole
*/
@Internal
public final class MappingHelper {
private final static Properties EMPTY_PROPERTIES = new Properties();
private MappingHelper() {
}
public static CollectionType customCollection(
String typeName,
Properties typeParameters,
String role,
String propertyRef,
MetadataImplementor metadata) {
final ClassLoaderService cls = metadata.getMetadataBuildingOptions().getServiceRegistry().getService( ClassLoaderService.class );
final Class extends UserCollectionType> userCollectionTypeClass = cls.classForName( typeName );
final boolean hasParameters = CollectionHelper.isNotEmpty( typeParameters );
final ManagedBean extends UserCollectionType> userTypeBean;
if ( !metadata.getMetadataBuildingOptions().isAllowExtensionsInCdi() ) {
//noinspection unchecked,rawtypes
userTypeBean = createLocalUserCollectionTypeBean(
role,
userCollectionTypeClass,
hasParameters,
(Map) typeParameters
);
}
else {
final ManagedBeanRegistry beanRegistry = metadata
.getMetadataBuildingOptions()
.getServiceRegistry()
.getService( ManagedBeanRegistry.class );
final ManagedBean extends UserCollectionType> userCollectionTypeBean = beanRegistry.getBean( userCollectionTypeClass );
if ( hasParameters ) {
if ( ParameterizedType.class.isAssignableFrom( userCollectionTypeBean.getBeanClass() ) ) {
// create a copy of the parameters and create a bean wrapper to delay injecting
// the parameters, thereby delaying the need to resolve the instance from the
// wrapped bean
final Properties copy = new Properties();
copy.putAll( typeParameters );
userTypeBean = new DelayedParameterizedTypeBean<>( userCollectionTypeBean, copy );
}
else {
// there were parameters, but the custom-type does not implement the interface
// used to inject them - log a "warning"
BootLogging.BOOT_LOGGER.debugf(
"`@CollectionType` (%s) specified parameters, but the" +
" implementation does not implement `%s` which is used to inject them - `%s`",
role,
ParameterizedType.class.getName(),
userCollectionTypeClass.getName()
);
userTypeBean = userCollectionTypeBean;
}
}
else {
userTypeBean = userCollectionTypeBean;
}
}
return new CustomCollectionType( userTypeBean, role, propertyRef );
}
public static void injectParameters(Object type, Properties parameters) {
if ( type instanceof ParameterizedType ) {
( (ParameterizedType) type ).setParameterValues( parameters == null ? EMPTY_PROPERTIES : parameters );
}
else if ( parameters != null && !parameters.isEmpty() ) {
MAPPING_MODEL_CREATION_MESSAGE_LOGGER.debugf(
"UserCollectionType impl does not implement ParameterizedType but parameters were present : `%s`",
type.getClass().getName()
);
}
}
public static void injectParameters(Object type, Supplier parameterAccess) {
injectParameters( type, parameterAccess.get() );
}
public static AnyType anyMapping(
Type metaType,
Type identifierType,
Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy