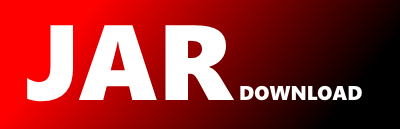
org.hibernate.sql.Insert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.sql;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import org.hibernate.Internal;
import org.hibernate.MappingException;
import org.hibernate.dialect.Dialect;
import org.hibernate.dialect.identity.IdentityColumnSupport;
import org.hibernate.engine.spi.SessionFactoryImplementor;
import org.hibernate.generator.OnExecutionGenerator;
import org.hibernate.sql.ast.spi.ParameterMarkerStrategy;
/**
* A SQL {@code INSERT} statement.
*
* @author Gavin King
*/
@Internal
public class Insert {
protected String tableName;
protected String comment;
protected Map columns = new LinkedHashMap<>();
private final Dialect dialect;
private final ParameterMarkerStrategy parameterMarkerStrategy;
private int parameterCount;
public Insert(SessionFactoryImplementor sessionFactory) {
this(
sessionFactory.getFastSessionServices().dialect,
sessionFactory.getFastSessionServices().parameterMarkerStrategy
);
}
public Insert(Dialect dialect, ParameterMarkerStrategy parameterMarkerStrategy) {
this.dialect = dialect;
this.parameterMarkerStrategy = parameterMarkerStrategy;
}
protected Dialect getDialect() {
return dialect;
}
public Insert setComment(String comment) {
this.comment = comment;
return this;
}
public Map getColumns() {
return columns;
}
public Insert addColumn(String columnName) {
return addColumn( columnName, "?" );
}
public Insert addColumns(String[] columnNames) {
for ( String columnName : columnNames ) {
addColumn( columnName );
}
return this;
}
public Insert addColumns(String[] columnNames, boolean[] insertable) {
for ( int i=0; i itr = columns.keySet().iterator();
while ( itr.hasNext() ) {
buf.append( itr.next() );
if ( itr.hasNext() ) {
buf.append( ", " );
}
}
}
private void renderRowValues(StringBuilder buf) {
final Iterator itr = columns.values().iterator();
while ( itr.hasNext() ) {
buf.append( normalizeExpressionFragment( itr.next() ) );
if ( itr.hasNext() ) {
buf.append( ", " );
}
}
}
private String normalizeExpressionFragment(String rhs) {
return rhs.equals( "?" )
? parameterMarkerStrategy.createMarker( ++parameterCount, null )
: rhs;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy