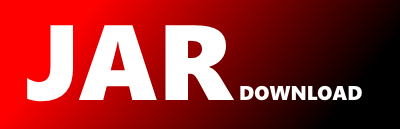
org.hibernate.annotations.OptimisticLocking Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import static java.lang.annotation.ElementType.TYPE;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
/**
* Specifies how optimistic lock checking works for the annotated entity.
*
* Optimistic lock checking may detect that an optimistic lock has failed,
* and that the transaction should be aborted, by comparing either:
*
* - the {@linkplain OptimisticLockType#VERSION version or timestamp},
*
- the {@linkplain OptimisticLockType#DIRTY dirty fields} of the
* entity instance, or
*
- {@linkplain OptimisticLockType#ALL all fields} of the entity.
*
*
* An optimistic lock is usually checked by including a restriction in a
* SQL {@code update} or {@code delete} statement. If the database reports
* that zero rows were updated, it is inferred that another transaction
* has already updated or deleted the row, and the failure of the optimistic
* lock is reported via an {@link jakarta.persistence.OptimisticLockException}.
*
* In an inheritance hierarchy, this annotation may only be applied to the
* root entity, since the optimistic lock checking strategy is inherited
* by entity subclasses.
*
* To exclude a particular attribute from optimistic locking, annotate the
* attribute {@link OptimisticLock @OptimisticLock(excluded=true)}. Then:
*
* - changes to that attribute will never trigger a version increment, and
*
- the attribute will not be included in the list of fields checked fields
* when {@link OptimisticLockType#ALL} or {@link OptimisticLockType#DIRTY}
* is used.
*
*
* @author Steve Ebersole
*
* @see org.hibernate.LockMode
* @see jakarta.persistence.LockModeType
*/
@Target( TYPE )
@Retention( RUNTIME )
public @interface OptimisticLocking {
/**
* The optimistic lock checking strategy.
*/
OptimisticLockType type() default OptimisticLockType.VERSION;
}