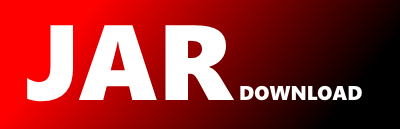
org.hibernate.annotations.ParamDef Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.annotations;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import java.util.function.Supplier;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
/**
* Details about a parameter declared in a {@link FilterDef}.
*
* Mainly used to support cases where the proper {@link #type type}
* cannot be deduced by Hibernate.
*
* @see FilterDef#parameters()
*
* @author Steve Ebersole
* @author Emmanuel Bernard
*/
@Target({})
@Retention(RUNTIME)
public @interface ParamDef {
/**
* The name of the parameter.
*/
String name();
/**
* The type to use when binding the parameter value.
*
* Generally deduced from the bind value. Allows to
* specify a specific type to use.
*
* The supplied {@link Class} may be one of the following:
* -
* a {@link org.hibernate.usertype.UserType}
*
* -
* an {@link jakarta.persistence.AttributeConverter}
*
* -
* a {@link org.hibernate.type.descriptor.java.JavaType}
*
* -
* any Java type resolvable from
* {@link org.hibernate.type.descriptor.java.spi.JavaTypeRegistry}
*
*
*/
Class> type();
/**
* A class implementing {@link Supplier} which provides arguments
* to this parameter. This is especially useful in the case of
* {@linkplain FilterDef#autoEnabled auto-enabled} filters.
*
* When a resolver is specified for a filter parameter, it's not
* necessary to provide an argument for the parameter by calling
* {@link org.hibernate.Filter#setParameter(String, Object)}.
*/
Class extends Supplier> resolver() default Supplier.class;
}