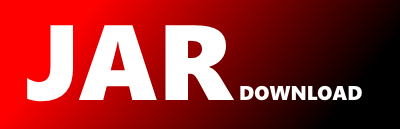
org.hibernate.graph.Graph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.graph;
import java.util.List;
import jakarta.persistence.metamodel.PluralAttribute;
import org.hibernate.metamodel.model.domain.ManagedDomainType;
import org.hibernate.metamodel.model.domain.PersistentAttribute;
/**
* A container for {@link AttributeNode} references.
*
* @apiNote Acts as an abstraction over the JPA-defined interfaces
* {@link jakarta.persistence.EntityGraph} and
* {@link jakarta.persistence.Subgraph}, which have no
* common supertype.
*
* @author Strong Liu
* @author Steve Ebersole
* @author Andrea Boriero
*
* @see RootGraph
* @see SubGraph
* @see jakarta.persistence.EntityGraph
* @see jakarta.persistence.Subgraph
*/
public interface Graph extends GraphNode {
/**
* Add a subgraph rooted at a plural attribute, allowing further
* nodes to be added to the subgraph.
*
* @apiNote This method is missing in JPA, and nodes cannot be
* added in a typesafe way to subgraphs representing
* fetched collections
*
* @since 6.3
*/
default SubGraph addPluralSubgraph(PluralAttribute extends J, ?, AJ> attribute) {
return addSubGraph( attribute.getName() );
}
/**
* Graphs apply only to {@link jakarta.persistence.metamodel.ManagedType}s.
*
* @return the {@code ManagedType} being graphed here.
*/
ManagedDomainType getGraphedType();
/**
* Create a named root {@link Graph} if the given name is not null.
*
* @param mutable controls whether the resulting {@code Graph} is mutable
*
* @throws CannotBecomeEntityGraphException If the named attribute is not entity-valued
*/
RootGraph makeRootGraph(String name, boolean mutable)
throws CannotBecomeEntityGraphException;
/**
* Create a new (mutable or immutable) {@link SubGraph} rooted at
* this {@link Graph}.
*/
SubGraph makeSubGraph(boolean mutable);
@Override
Graph makeCopy(boolean mutable);
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// AttributeNode handling
/**
* Ultimately only needed for implementing
* {@link jakarta.persistence.EntityGraph#getAttributeNodes()}
* and {@link jakarta.persistence.Subgraph#getAttributeNodes()}
*/
List> getGraphAttributeNodes();
/**
* Find an already existing AttributeNode by attributeName within
* this container
*/
AttributeNode findAttributeNode(String attributeName);
/**
* Find an already existing AttributeNode by corresponding attribute
* reference, within this container.
*/
AttributeNode findAttributeNode(PersistentAttribute extends J, AJ> attribute);
/**
* Get a list of all existing AttributeNodes within this container.
*/
List> getAttributeNodeList();
/**
* Add an {@link AttributeNode} (with no associated {@link SubGraph})
* to this container by attribute name.
*/
AttributeNode addAttributeNode(String attributeName);
/**
* Add an {@link AttributeNode} (with no associated {@link SubGraph})
* to this container by attribute reference.
*/
AttributeNode addAttributeNode(PersistentAttribute extends J,AJ> attribute);
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// sub graph nodes
/**
* Create and return a new (mutable) {@link SubGraph} associated with
* the named {@link AttributeNode}.
*
* @apiNote If no such AttributeNode exists yet, it is created.
*/
SubGraph addSubGraph(String attributeName)
throws CannotContainSubGraphException;
SubGraph addSubGraph(String attributeName, Class type)
throws CannotContainSubGraphException;
/**
* Create and return a new (mutable) {@link SubGraph} associated with
* the {@link AttributeNode} for the given attribute.
*
* @apiNote If no such AttributeNode exists yet, it is created.
*/
SubGraph addSubGraph(PersistentAttribute extends J, AJ> attribute)
throws CannotContainSubGraphException;
SubGraph extends AJ> addSubGraph(PersistentAttribute extends J, AJ> attribute, Class extends AJ> type)
throws CannotContainSubGraphException;
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// key sub graph nodes
SubGraph addKeySubGraph(String attributeName)
throws CannotContainSubGraphException;
SubGraph addKeySubGraph(String attributeName, Class type)
throws CannotContainSubGraphException;
SubGraph addKeySubGraph(PersistentAttribute extends J,AJ> attribute)
throws CannotContainSubGraphException;
SubGraph extends AJ> addKeySubGraph(PersistentAttribute extends J,AJ> attribute, Class extends AJ> type)
throws CannotContainSubGraphException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy