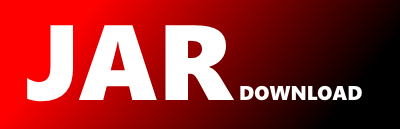
org.hibernate.graph.GraphParser Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.graph;
import jakarta.persistence.EntityGraph;
import jakarta.persistence.EntityManager;
import jakarta.persistence.EntityManagerFactory;
import jakarta.persistence.Subgraph;
import org.hibernate.engine.spi.SessionFactoryImplementor;
import org.hibernate.engine.spi.SessionImplementor;
import org.hibernate.graph.spi.GraphImplementor;
import org.hibernate.graph.spi.RootGraphImplementor;
/**
* Parser for string representations of JPA {@link jakarta.persistence.EntityGraph}
* ({@link RootGraph}) and {@link jakarta.persistence.Subgraph} ({@link SubGraph}),
* using a simple syntax defined by the `graph.g` Antlr grammar.
*
* The {@link #parse} methods all create a root {@link jakarta.persistence.EntityGraph}
* based on the passed entity class and parse the graph string into that root graph.
*
* The {@link #parseInto} methods parse the graph string into a passed graph, which may be a sub-graph
*
* Multiple graphs made for the same entity type can be merged. See {@link EntityGraphs#merge(SessionImplementor, Class, GraphImplementor...)}.
*
* @author asusnjar
*/
@SuppressWarnings("unused")
public final class GraphParser {
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Parse (creation)
/**
* Creates a root graph based on the passed `rootType` and parses `graphText` into
* the generated root graph
*
* @apiNote The passed EntityManager is expected to be a Hibernate implementation.
* Attempting to pass another provider's EntityManager implementation will fail
*
* @param rootType The root entity type
* @param graphText The textual representation of the graph
* @param entityManager The EntityManager
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
public static RootGraph parse(
final Class rootType,
final CharSequence graphText,
final EntityManager entityManager) {
return parse( rootType, graphText, (SessionImplementor) entityManager );
}
private static RootGraphImplementor parse(
final Class rootType,
final CharSequence graphText,
final SessionImplementor session) {
if ( graphText == null ) {
return null;
}
final RootGraphImplementor graph = session.createEntityGraph( rootType );
parseInto( (GraphImplementor) graph, graphText, session.getSessionFactory() );
return graph;
}
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Parse (into)
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManager The EntityManager
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
public static void parseInto(
final Graph graph,
final CharSequence graphText,
final EntityManager entityManager) {
parseInto(
(GraphImplementor) graph,
graphText,
( (SessionImplementor) entityManager ).getSessionFactory()
);
}
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManager The EntityManager
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
@SuppressWarnings("unchecked")
public static void parseInto(
final EntityGraph graph,
final CharSequence graphText,
final EntityManager entityManager) {
parseInto(
(GraphImplementor) graph,
graphText,
( (SessionImplementor) entityManager ).getSessionFactory()
);
}
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManager The EntityManager
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
@SuppressWarnings("unchecked")
public static void parseInto(
final Subgraph graph,
final CharSequence graphText,
final EntityManager entityManager) {
parseInto(
(GraphImplementor) graph,
graphText,
( (SessionImplementor) entityManager ).getSessionFactory()
);
}
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManagerFactory The EntityManagerFactory
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
public static void parseInto(
final Graph graph,
final CharSequence graphText,
final EntityManagerFactory entityManagerFactory) {
parseInto(
(GraphImplementor) graph,
graphText,
(SessionFactoryImplementor) entityManagerFactory
);
}
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManagerFactory The EntityManagerFactory
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
@SuppressWarnings("unchecked")
public static void parseInto(
final EntityGraph graph,
final CharSequence graphText,
final EntityManagerFactory entityManagerFactory) {
parseInto(
(GraphImplementor) graph,
graphText,
(SessionFactoryImplementor) entityManagerFactory
);
}
/**
* Parses the textual graph representation into the specified graph.
*
* @param graph The target graph. This is the graph that will be populated by this process
* @param graphText Textual representation of the graph
* @param entityManagerFactory The EntityManagerFactory
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
@SuppressWarnings("unchecked")
public static void parseInto(
final Subgraph graph,
final CharSequence graphText,
final EntityManagerFactory entityManagerFactory) {
parseInto(
(GraphImplementor) graph,
graphText,
(SessionFactoryImplementor) entityManagerFactory
);
}
/**
* Parses the textual graph representation as {@linkplain GraphParser described above}
* into the specified graph.
*
* @param The Java type for the ManagedType described by `graph`
*
* @param graph The target graph. This is the graph that will be populated
* by this process
* @param graphText Textual representation of the graph
* @param sessionFactory The SessionFactory reference
*
* @throws InvalidGraphException if the textual representation is invalid.
*/
private static void parseInto(
GraphImplementor graph,
final CharSequence graphText,
SessionFactoryImplementor sessionFactory) {
if ( graphText != null ) {
org.hibernate.graph.internal.parse.GraphParser.parseInto( graph, graphText, sessionFactory );
}
}
}