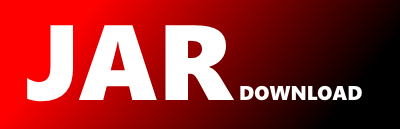
org.hibernate.query.Order Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.query;
import jakarta.persistence.metamodel.SingularAttribute;
import org.hibernate.Incubating;
import java.util.List;
import java.util.Objects;
import static java.util.stream.Collectors.toList;
import static org.hibernate.query.SortDirection.ASCENDING;
import static org.hibernate.query.SortDirection.DESCENDING;
/**
* A rule for sorting a query result set.
*
* This is a convenience class which allows query result ordering
* rules to be passed around the system before being applied to
* a {@link Query} by calling {@link SelectionQuery#setOrder}.
*
* A parameter of a {@linkplain org.hibernate.annotations.processing.Find
* finder method} or {@linkplain org.hibernate.annotations.processing.HQL
* HQL query method} may be declared with type {@code Order super E>},
* {@code List>}, or {@code Order super E>...} (varargs)
* where {@code E} is the entity type returned by the query.
*
* @param The result type of the query to be sorted
*
* @see SelectionQuery#setOrder(Order)
* @see SelectionQuery#setOrder(java.util.List)
*
* @author Gavin King
*
* @since 6.3
*/
@Incubating
public class Order {
private final SortDirection order;
private final SingularAttribute attribute;
private final Class entityClass;
private final String attributeName;
private final NullPrecedence nullPrecedence;
private final int element;
private final boolean ignoreCase;
private Order(SortDirection order, NullPrecedence nullPrecedence, SingularAttribute attribute) {
this.order = order;
this.attribute = attribute;
this.attributeName = attribute.getName();
this.entityClass = attribute.getDeclaringType().getJavaType();
this.nullPrecedence = nullPrecedence;
this.element = 1;
this.ignoreCase = false;
}
private Order(SortDirection order, NullPrecedence nullPrecedence, SingularAttribute attribute, boolean ignoreCase) {
this.order = order;
this.attribute = attribute;
this.attributeName = attribute.getName();
this.entityClass = attribute.getDeclaringType().getJavaType();
this.nullPrecedence = nullPrecedence;
this.element = 1;
this.ignoreCase = ignoreCase;
}
private Order(SortDirection order, NullPrecedence nullPrecedence, Class entityClass, String attributeName) {
this.order = order;
this.entityClass = entityClass;
this.attributeName = attributeName;
this.attribute = null;
this.nullPrecedence = nullPrecedence;
this.element = 1;
this.ignoreCase = false;
}
private Order(SortDirection order, NullPrecedence nullPrecedence, int element) {
this.order = order;
this.entityClass = null;
this.attributeName = null;
this.attribute = null;
this.nullPrecedence = nullPrecedence;
this.element = element;
this.ignoreCase = false;
}
private Order(SortDirection order, NullPrecedence nullPrecedence, Class entityClass, String attributeName, boolean ignoreCase) {
this.order = order;
this.entityClass = entityClass;
this.attributeName = attributeName;
this.attribute = null;
this.nullPrecedence = nullPrecedence;
this.element = 1;
this.ignoreCase = ignoreCase;
}
private Order(SortDirection order, NullPrecedence nullPrecedence, int element, boolean ignoreCase) {
this.order = order;
this.entityClass = null;
this.attributeName = null;
this.attribute = null;
this.nullPrecedence = nullPrecedence;
this.element = element;
this.ignoreCase = ignoreCase;
}
private Order(Order other, SortDirection order) {
this.order = order;
this.attribute = other.attribute;
this.entityClass = other.entityClass;
this.attributeName = other.attributeName;
this.nullPrecedence = other.nullPrecedence;
this.element = other.element;
this.ignoreCase = other.ignoreCase;
}
private Order(Order other, boolean ignoreCase) {
this.order = other.order;
this.attribute = other.attribute;
this.entityClass = other.entityClass;
this.attributeName = other.attributeName;
this.nullPrecedence = other.nullPrecedence;
this.element = other.element;
this.ignoreCase = ignoreCase;
}
private Order(Order other, NullPrecedence nullPrecedence) {
this.order = other.order;
this.attribute = other.attribute;
this.entityClass = other.entityClass;
this.attributeName = other.attributeName;
this.nullPrecedence = nullPrecedence;
this.element = other.element;
this.ignoreCase = other.ignoreCase;
}
/**
* An order where an entity is sorted by the given attribute,
* with smaller values first. If the give attribute is of textual
* type, the ordering is case-sensitive.
*/
public static Order asc(SingularAttribute attribute) {
return new Order<>(ASCENDING, NullPrecedence.NONE, attribute);
}
/**
* An order where an entity is sorted by the given attribute,
* with larger values first. If the give attribute is of textual
* type, the ordering is case-sensitive.
*/
public static Order desc(SingularAttribute attribute) {
return new Order<>(DESCENDING, NullPrecedence.NONE, attribute);
}
/**
* An order where an entity is sorted by the given attribute,
* in the given direction. If the give attribute is of textual
* type, the ordering is case-sensitive.
*/
public static Order by(SingularAttribute attribute, SortDirection direction) {
return new Order<>(direction, NullPrecedence.NONE, attribute);
}
/**
* An order where an entity is sorted by the given attribute,
* in the given direction, with the specified case-sensitivity.
*/
public static Order by(SingularAttribute attribute, SortDirection direction, boolean ignoreCase) {
return new Order<>(direction, NullPrecedence.NONE, attribute, ignoreCase);
}
/**
* An order where an entity is sorted by the given attribute,
* in the given direction, with the specified precedence for
* null values. If the give attribute is of textual type, the
* ordering is case-sensitive.
*/
public static Order by(SingularAttribute attribute, SortDirection direction, NullPrecedence nullPrecedence) {
return new Order<>(direction, nullPrecedence, attribute);
}
/**
* An order where an entity of the given class is sorted by the
* attribute with the given name, with smaller values first. If
* the named attribute is of textual type, the ordering is
* case-sensitive.
*/
public static Order asc(Class entityClass, String attributeName) {
return new Order<>( ASCENDING, NullPrecedence.NONE, entityClass, attributeName );
}
/**
* An order where an entity of the given class is sorted by the
* attribute with the given name, with larger values first. If
* the named attribute is of textual type, the ordering is
* case-sensitive.
*/
public static Order desc(Class entityClass, String attributeName) {
return new Order<>( DESCENDING, NullPrecedence.NONE, entityClass, attributeName );
}
/**
* An order where an entity of the given class is sorted by the
* attribute with the given name, in the given direction. If the
* named attribute is of textual type, the ordering is
* case-sensitive.
*/
public static Order by(Class entityClass, String attributeName, SortDirection direction) {
return new Order<>( direction, NullPrecedence.NONE, entityClass, attributeName );
}
/**
* An order where an entity of the given class is sorted by the
* attribute with the given name, in the given direction, with
* the specified case-sensitivity.
*/
public static Order by(Class entityClass, String attributeName, SortDirection direction, boolean ignoreCase) {
return new Order<>( direction, NullPrecedence.NONE, entityClass, attributeName, ignoreCase );
}
/**
* An order where an entity of the given class is sorted by the
* attribute with the given name, in the given direction. If the
* named attribute is of textual type, with the specified
* precedence for null values. If the named attribute is of
* textual type, the ordering is case-sensitive.
*/
public static Order by(Class entityClass, String attributeName, SortDirection direction, NullPrecedence nullPrecedence) {
return new Order<>( direction, nullPrecedence, entityClass, attributeName );
}
/**
* An order where the result set is sorted by the select item
* in the given position with smaller values first. If the
* item is of textual type, the ordering is case-sensitive.
*/
public static Order