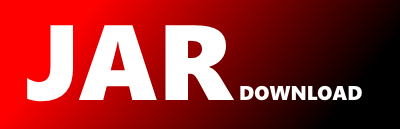
org.hibernate.sql.InFragment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.sql;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import org.hibernate.Internal;
import org.hibernate.internal.util.StringHelper;
/**
* A SQL {@code IN} expression.
*
* @author Gavin King
*/
@Internal
public class InFragment {
public static final String NULL = "null";
public static final String NOT_NULL = "not null";
protected String columnName;
protected List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy