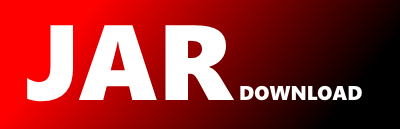
org.hibernate.cfg.Environment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.cfg;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import org.hibernate.HibernateException;
import org.hibernate.Internal;
import org.hibernate.Version;
import org.hibernate.internal.CoreMessageLogger;
import org.hibernate.internal.util.ConfigHelper;
import org.hibernate.internal.util.config.ConfigurationHelper;
import org.jboss.logging.Logger;
/**
* Provides access to configuration properties passed in {@link Properties} objects.
*
* Hibernate has two property scopes:
*
* - Factory-level properties are specified when a
* {@link org.hibernate.SessionFactory} is configured and instantiated. Each instance
* might have different property values.
*
- System-level properties are shared by all factory instances and are
* always determined by the {@code Environment} properties in {@link #getProperties()}.
*
*
* {@code Environment} properties are populated by calling {@link System#getProperties()}
* and then from a resource named {@code /hibernate.properties}, if it exists. System
* properties override properties specified in {@code hibernate.properties}.
*
* The {@link org.hibernate.SessionFactory} obtains properties from:
*
* - {@link System#getProperties() system properties},
*
- properties defined in a resource named {@code /hibernate.properties}, and
*
- any instance of {@link Properties} passed to {@link Configuration#addProperties}.
*
*
* Configuration properties
* Property Interpretation
*
* {@value #DIALECT}
* name of {@link org.hibernate.dialect.Dialect} subclass
*
*
* {@value #CONNECTION_PROVIDER}
* name of a {@link org.hibernate.engine.jdbc.connections.spi.ConnectionProvider}
* subclass (if not specified heuristics are used)
*
* {@value #USER} database username
* {@value #PASS} database password
*
* {@value #URL}
* JDBC URL (when using {@link java.sql.DriverManager})
*
*
* {@value #DRIVER}
* classname of JDBC driver
*
*
* {@value #ISOLATION}
* JDBC transaction isolation level (only when using
* {@link java.sql.DriverManager})
*
*
*
* {@value #POOL_SIZE}
* the maximum size of the connection pool (only when using
* {@link java.sql.DriverManager})
*
*
*
* {@value #DATASOURCE}
* datasource JNDI name (when using {@link javax.sql.DataSource})
*
*
* {@value #JNDI_URL} JNDI {@link javax.naming.InitialContext} URL
*
*
* {@value #JNDI_CLASS} JNDI {@link javax.naming.InitialContext} class name
*
*
* {@value #MAX_FETCH_DEPTH}
* maximum depth of outer join fetching
*
*
* {@value #STATEMENT_BATCH_SIZE}
* enable use of JDBC2 batch API for drivers which support it
*
*
* {@value #STATEMENT_FETCH_SIZE}
* set the JDBC fetch size
*
*
* {@value #USE_GET_GENERATED_KEYS}
* enable use of JDBC3 {@link java.sql.PreparedStatement#getGeneratedKeys()}
* to retrieve natively generated keys after insert. Requires JDBC3+ driver and
* JRE1.4+
*
*
* {@value #HBM2DDL_AUTO}
* enable auto DDL export
*
*
* {@value #DEFAULT_SCHEMA}
* use given schema name for unqualified tables (always optional)
*
*
* {@value #DEFAULT_CATALOG}
* use given catalog name for unqualified tables (always optional)
*
*
* {@value #JTA_PLATFORM}
* name of {@link org.hibernate.engine.transaction.jta.platform.spi.JtaPlatform}
* implementation
*
*
*
* @see org.hibernate.SessionFactory
*
* @apiNote This is really considered an internal contract, but leaving in place in this
* package as many applications use it historically. However, consider migrating to use
* {@link AvailableSettings} instead.
*
* @author Gavin King
*/
@Internal
public final class Environment implements AvailableSettings {
private static final CoreMessageLogger LOG = Logger.getMessageLogger( CoreMessageLogger.class, Environment.class.getName());
private static final Properties GLOBAL_PROPERTIES;
static {
Version.logVersion();
GLOBAL_PROPERTIES = new Properties();
try {
InputStream stream = ConfigHelper.getResourceAsStream( "/hibernate.properties" );
try {
GLOBAL_PROPERTIES.load(stream);
LOG.propertiesLoaded( ConfigurationHelper.maskOut( GLOBAL_PROPERTIES, PASS ) );
}
catch (Exception e) {
LOG.unableToLoadProperties();
}
finally {
try{
stream.close();
}
catch (IOException ioe){
LOG.unableToCloseStreamError( ioe );
}
}
}
catch (HibernateException he) {
LOG.propertiesNotFound();
}
try {
Properties systemProperties = System.getProperties();
// Must be thread-safe in case an application changes System properties during Hibernate initialization.
// See HHH-8383.
synchronized (systemProperties) {
GLOBAL_PROPERTIES.putAll(systemProperties);
}
}
catch (SecurityException se) {
LOG.unableToCopySystemProperties();
}
}
/**
* Disallow instantiation
*/
private Environment() {
//not to be constructed
}
/**
* The {@link System#getProperties() system properties}, extended with all
* additional properties specified in {@code hibernate.properties}.
*/
public static Properties getProperties() {
Properties copy = new Properties();
copy.putAll(GLOBAL_PROPERTIES);
return copy;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy