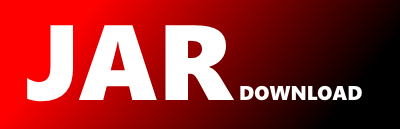
org.hibernate.internal.TransactionManagement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beangle-hibernate-core Show documentation
Show all versions of beangle-hibernate-core Show documentation
Hibernate Orm Core Shade,Support Scala Collection
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.internal;
import org.hibernate.Transaction;
import org.hibernate.TransactionManagementException;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Methods used by {@link org.hibernate.SessionFactory} to manage transactions.
*/
public class TransactionManagement {
public static void manageTransaction(S session, Transaction transaction, Consumer consumer) {
try {
consumer.accept( session );
commit( transaction );
}
catch ( RuntimeException exception ) {
rollback( transaction, exception );
throw exception;
}
}
public static R manageTransaction(S session, Transaction transaction, Function function) {
try {
R result = function.apply( session );
commit( transaction );
return result;
}
catch ( RuntimeException exception ) {
// an error happened in the action or during commit()
rollback( transaction, exception );
throw exception;
}
}
private static void rollback(Transaction transaction, RuntimeException exception) {
// an error happened in the action or during commit()
if ( transaction.isActive() ) {
try {
transaction.rollback();
}
catch ( RuntimeException e ) {
exception.addSuppressed( e );
}
}
}
private static void commit(Transaction transaction) {
if ( !transaction.isActive() ) {
throw new TransactionManagementException(
"Execution of action caused managed transaction to be completed" );
}
// The action completed without throwing an exception,
// so we attempt to commit the transaction, allowing
// any RollbackException to propagate. Note that when
// we get here we know that the transaction is active
transaction.commit();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy