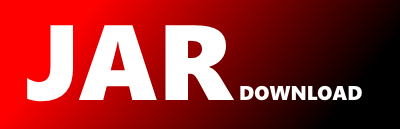
org.hibernate.query.NativeQuery Maven / Gradle / Ivy
Show all versions of beangle-hibernate-core Show documentation
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later
* See the lgpl.txt file in the root directory or http://www.gnu.org/licenses/lgpl-2.1.html
*/
package org.hibernate.query;
import java.time.Instant;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.Map;
import jakarta.persistence.AttributeConverter;
import jakarta.persistence.CacheRetrieveMode;
import jakarta.persistence.CacheStoreMode;
import jakarta.persistence.FlushModeType;
import jakarta.persistence.LockModeType;
import jakarta.persistence.Parameter;
import jakarta.persistence.TemporalType;
import jakarta.persistence.metamodel.SingularAttribute;
import org.hibernate.CacheMode;
import org.hibernate.FlushMode;
import org.hibernate.LockMode;
import org.hibernate.LockOptions;
import org.hibernate.MappingException;
import org.hibernate.metamodel.mapping.EntityMappingType;
import org.hibernate.metamodel.mapping.PluralAttributeMapping;
import org.hibernate.metamodel.model.domain.BasicDomainType;
import org.hibernate.spi.NavigablePath;
import org.hibernate.transform.ResultTransformer;
import org.hibernate.type.BasicTypeReference;
/**
* Within the context of an active {@linkplain org.hibernate.Session session},
* an instance of this type represents an executable query written in the
* native SQL dialect of the underlying database. Since Hibernate does not
* actually understand SQL, it often requires some help in interpreting the
* semantics of a native SQL query.
*
* Along with the operations inherited from {@link Query}, this interface
* provides control over:
*
* - mapping the result set of the native SQL query, and
*
- synchronization of the database with state held in memory before
* execution of the query, via automatic flushing of the session.
*
*
* A {@code NativeQuery} may be obtained from the {@link org.hibernate.Session}
* by calling:
*
* - {@link QueryProducer#createNativeQuery(String, Class)}, passing
* native SQL as a string, or
*
- {@link QueryProducer#createNativeQuery(String, String, Class)}
* passing the native SQL string and the name of a result set mapping
* defined using {@link jakarta.persistence.SqlResultSetMapping}.
*
*
* A result set mapping may be specified by:
*
* - a named {@link jakarta.persistence.SqlResultSetMapping} passed to
* {@link QueryProducer#createNativeQuery(String, String, Class)},
*
- a named {@link jakarta.persistence.SqlResultSetMapping} specified
* using {@link jakarta.persistence.NamedNativeQuery#resultSetMapping}
* for a named query, or
*
- by calling the various {@link #addEntity}, {@link #addRoot},
* {@link #addJoin}, {@link #addFetch} and {@link #addScalar} methods
* of this object.
*
*
* The third option is a legacy of much older versions of Hibernate and is
* currently disfavored.
*
* To determine if an automatic {@linkplain org.hibernate.Session#flush flush}
* is required before execution of the query, Hibernate must know which tables
* affect the query result set. JPA provides no standard way to do this.
* Instead, this information may be provided via:
*
* - {@link org.hibernate.annotations.NamedNativeQuery#querySpaces} for
* a named query, or
*
- by calling {@link #addSynchronizedEntityClass},
* {@link #addSynchronizedEntityName}, or
* {@link #addSynchronizedQuerySpace}.
*
*
* @author Gavin King
* @author Steve Ebersole
*
* @see Query
* @see SynchronizeableQuery
* @see QueryProducer
*/
public interface NativeQuery extends Query, SynchronizeableQuery {
/**
* Declare a scalar query result. Hibernate will attempt to automatically
* detect the underlying type.
*
* Functions like {@code } in {@code hbm.xml} or
* {@link jakarta.persistence.ColumnResult} in annotations
*
* @param columnAlias The column alias in the result set to be
* processed as a scalar result
*
* @return {@code this}, for method chaining
*/
NativeQuery addScalar(String columnAlias);
/**
* Declare a scalar query result.
*
* Functions like {@code } in {@code hbm.xml} or
* {@link jakarta.persistence.ColumnResult} in annotations.
*
* @param columnAlias The column alias in the result set to be
* processed as a scalar result
* @param type The Hibernate type as which to treat the value.
*
* @return {@code this}, for method chaining
*/
NativeQuery addScalar(String columnAlias, @SuppressWarnings("rawtypes") BasicTypeReference type);
/**
* Declare a scalar query result.
*
* Functions like {@code } in {@code hbm.xml} or
* {@link jakarta.persistence.ColumnResult} in annotations.
*
* @param columnAlias The column alias in the result set to be
* processed as a scalar result
* @param type The Hibernate type as which to treat the value.
*
* @return {@code this}, for method chaining
*/
NativeQuery addScalar(String columnAlias, @SuppressWarnings("rawtypes") BasicDomainType type);
/**
* Declare a scalar query result using the specified result type.
*
* Hibernate will implicitly determine an appropriate conversion,
* if it can. Otherwise, an exception will be thrown.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addScalar(String columnAlias, @SuppressWarnings("rawtypes") Class javaType);
/**
* Declare a scalar query result with an explicit conversion.
*
* @param relationalJavaType The Java type expected by the converter as its
* "relational" type.
* @param converter The conversion to apply. Consumes the JDBC value based
* on {@code relationalJavaType}.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addScalar(String columnAlias, Class relationalJavaType, AttributeConverter,C> converter);
/**
* Declare a scalar query result with an explicit conversion.
*
* @param jdbcJavaType The Java type expected by the converter as its
* "relational model" type.
* @param domainJavaType The Java type expected by the converter as its
* "object model" type.
* @param converter The conversion to apply. Consumes the JDBC value based
* on {@code relationalJavaType}.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addScalar(String columnAlias, Class domainJavaType, Class jdbcJavaType, AttributeConverter converter);
/**
* Declare a scalar query result with an explicit conversion.
*
* @param relationalJavaType The Java type expected by the converter as its
* "relational" type.
* @param converter The conversion to apply. Consumes the JDBC value based
* on {@code relationalJavaType}.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addScalar(String columnAlias, Class relationalJavaType, Class extends AttributeConverter,C>> converter);
/**
* Declare a scalar query result with an explicit conversion.
*
* @param jdbcJavaType The Java type expected by the converter as its
* "relational model" type.
* @param domainJavaType The Java type expected by the converter as its
* "object model" type.
* @param converter The conversion to apply. Consumes the JDBC value
* based on {@code relationalJavaType}.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addScalar(
String columnAlias,
Class domainJavaType,
Class jdbcJavaType,
Class extends AttributeConverter> converter);
InstantiationResultNode addInstantiation(Class targetJavaType);
/**
* Defines a result based on a specified attribute. Differs from adding
* a scalar in that any conversions or other semantics defined on the
* attribute are automatically applied to the mapping.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addAttributeResult(String columnAlias, @SuppressWarnings("rawtypes") Class entityJavaType, String attributePath);
/**
* Defines a result based on a specified attribute. Differs from adding
* a scalar in that any conversions or other semantics defined on the
* attribute are automatically applied to the mapping.
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addAttributeResult(String columnAlias, String entityName, String attributePath);
/**
* Defines a result based on a specified attribute. Differs from adding a
* scalar in that any conversions or other semantics defined on the attribute
* are automatically applied to the mapping.
*
* This form accepts the JPA Attribute mapping describing the attribute
*
* @return {@code this}, for method chaining
*
* @since 6.0
*/
NativeQuery addAttributeResult(String columnAlias, @SuppressWarnings("rawtypes") SingularAttribute attribute);
/**
* Add a new root return mapping, returning a {@link RootReturn} to allow
* further definition.
*
* @param tableAlias The SQL table alias to map to this entity
* @param entityName The name of the entity
*
* @return The return config object for further control.
*
* @since 3.6
*/
RootReturn addRoot(String tableAlias, String entityName);
/**
* Add a new root return mapping, returning a {@link RootReturn} to allow
* further definition.
*
* @param tableAlias The SQL table alias to map to this entity
* @param entityType The java type of the entity
*
* @return The return config object for further control.
*
* @since 3.6
*/
RootReturn addRoot(String tableAlias, @SuppressWarnings("rawtypes") Class entityType);
/**
* Declare a "root" entity, without specifying an alias. The expectation
* here is that the table alias is the same as the unqualified entity name.
*
* Use {@link #addRoot} if you need further control of the mapping
*
* @param entityName The entity name that is the root return of the query
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(String entityName);
/**
* Declare a "root" entity.
*
* @param tableAlias The SQL table alias
* @param entityName The entity name
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(String tableAlias, String entityName);
/**
* Declare a "root" entity, specifying a lock mode.
*
* @param tableAlias The SQL table alias
* @param entityName The entity name
* @param lockMode The lock mode for this return.
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(String tableAlias, String entityName, LockMode lockMode);
/**
* Declare a "root" entity, without specifying an alias. The expectation here
* is that the table alias is the same as the unqualified entity name.
*
* @param entityType The java type of the entity to add as a root
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(@SuppressWarnings("rawtypes") Class entityType);
/**
* Declare a "root" entity.
*
* @param tableAlias The SQL table alias
* @param entityType The java type of the entity to add as a root
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(String tableAlias, @SuppressWarnings("rawtypes") Class entityType);
/**
* Declare a "root" entity, specifying a lock mode.
*
* @param tableAlias The SQL table alias
* @param entityClass The entity {@link Class}
* @param lockMode The lock mode for this return
*
* @return {@code this}, for method chaining
*/
NativeQuery addEntity(String tableAlias, @SuppressWarnings("rawtypes") Class entityClass, LockMode lockMode);
/**
* Declare a join fetch result.
*
* @param tableAlias The SQL table alias for the data to be mapped to this fetch.
* @param ownerTableAlias Identify the table alias of the owner of this association.
* Should match the alias of a previously added root or fetch.
* @param joinPropertyName The name of the property being join fetched.
*
* @return The return config object for further control.
*
* @since 3.6
*/
FetchReturn addFetch(String tableAlias, String ownerTableAlias, String joinPropertyName);
/**
* Declare a join fetch result.
*
* @param tableAlias The SQL table alias for the data to be mapped to this fetch.
* @param path The association path of form {@code [owner-alias].[property-name]}.
*
* @return {@code this}, for method chaining
*/
NativeQuery addJoin(String tableAlias, String path);
/**
* Declare a join fetch result.
*
* @param tableAlias The SQL table alias for the data to be mapped to this fetch
* @param ownerTableAlias Identify the table alias of the owner of this association.
* Should match the alias of a previously added root or fetch.
* @param joinPropertyName The name of the property being join fetched.
*
* @return {@code this}, for method chaining
*
* @since 3.6
*/
NativeQuery addJoin(String tableAlias, String ownerTableAlias, String joinPropertyName);
/**
* Declare a join fetch result, specifying a lock mode.
*
* @param tableAlias The SQL table alias for the data to be mapped to this fetch
* @param path The association path of form {@code [owner-alias].[property-name]}.
* @param lockMode The lock mode for this return.
*
* @return {@code this}, for method chaining
*/
NativeQuery addJoin(String tableAlias, String path, LockMode lockMode);
/**
* Simple unification interface for all returns from the various {@code addXYZ()}
* methods. Allows control over the "shape" of that particular part of the fetch
* graph.
*
* Some nodes can be query results, while others simply describe a part of one of
* the results.
*/
interface ResultNode {
}
/**
* A {@link ResultNode} which can be a query result.
*/
interface ReturnableResultNode extends ResultNode {
}
interface InstantiationResultNode extends ReturnableResultNode {
default InstantiationResultNode addBasicArgument(String columnAlias) {
return addBasicArgument( columnAlias, null );
}
InstantiationResultNode addBasicArgument(String columnAlias, String argumentAlias);
}
/**
* Allows access to further control how properties within a root or join
* fetch are mapped back from the result set. Generally used in composite
* value scenarios.
*/
interface ReturnProperty extends ResultNode {
/**
* Add a column alias to this property mapping.
*
* @param columnAlias The column alias.
*
* @return {@code this}, for method chaining
*/
ReturnProperty addColumnAlias(String columnAlias);
}
/**
* Allows access to further control how root returns are mapped back from
* result sets.
*/
interface RootReturn extends ReturnableResultNode {
String getTableAlias();
String getDiscriminatorAlias();
EntityMappingType getEntityMapping();
NavigablePath getNavigablePath();
LockMode getLockMode();
/**
* Set the lock mode for this return.
*
* @param lockMode The new lock mode.
*
* @return {@code this}, for method chaining
*/
RootReturn setLockMode(LockMode lockMode);
RootReturn addIdColumnAliases(String... aliases);
/**
* Name the column alias that identifies the entity's discriminator.
*
* @param columnAlias The discriminator column alias
*
* @return {@code this}, for method chaining
*/
RootReturn setDiscriminatorAlias(String columnAlias);
/**
* Add a simple property-to-one-column mapping.
*
* @param propertyName The name of the property.
* @param columnAlias The name of the column
*
* @return {@code this}, for method chaining
*/
RootReturn addProperty(String propertyName, String columnAlias);
/**
* Add a property, presumably with more than one column.
*
* @param propertyName The name of the property.
*
* @return The config object for further control.
*/
ReturnProperty addProperty(String propertyName);
}
/**
* Allows access to further control how collection returns are mapped back
* from result sets.
*/
interface CollectionReturn extends ReturnableResultNode {
String getTableAlias();
PluralAttributeMapping getPluralAttribute();
NavigablePath getNavigablePath();
}
/**
* Allows access to further control how join fetch returns are mapped back
* from result sets.
*/
interface FetchReturn extends ResultNode {
String getTableAlias();
String getOwnerAlias();
String getFetchableName();
/**
* Set the lock mode for this return.
*
* @param lockMode The new lock mode.
*
* @return {@code this}, for method chaining
*/
FetchReturn setLockMode(LockMode lockMode);
/**
* Add a simple property-to-one-column mapping.
*
* @param propertyName The name of the property.
* @param columnAlias The name of the column
*
* @return {@code this}, for method chaining
*/
FetchReturn addProperty(String propertyName, String columnAlias);
/**
* Add a property, presumably with more than one column.
*
* @param propertyName The name of the property.
*
* @return The config object for further control.
*/
ReturnProperty addProperty(String propertyName);
}
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// covariant overrides - SynchronizeableQuery
@Override
NativeQuery addSynchronizedQuerySpace(String querySpace);
@Override
NativeQuery addSynchronizedEntityName(String entityName) throws MappingException;
@Override
NativeQuery addSynchronizedEntityClass(@SuppressWarnings("rawtypes") Class entityClass) throws MappingException;
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// covariant overrides - Query
@Override
NativeQuery setHibernateFlushMode(FlushMode flushMode);
@Override
NativeQuery setFlushMode(FlushModeType flushMode);
@Override
NativeQuery setCacheMode(CacheMode cacheMode);
@Override
NativeQuery setCacheStoreMode(CacheStoreMode cacheStoreMode);
@Override
NativeQuery setCacheRetrieveMode(CacheRetrieveMode cacheRetrieveMode);
@Override
NativeQuery setCacheable(boolean cacheable);
@Override
NativeQuery setCacheRegion(String cacheRegion);
@Override
NativeQuery setTimeout(int timeout);
@Override
NativeQuery setFetchSize(int fetchSize);
@Override
NativeQuery setReadOnly(boolean readOnly);
/**
* @inheritDoc
*
* This operation is supported even for native queries.
* Note that specifying an explicit lock mode might
* result in changes to the native SQL query that is
* actually executed.
*/
@Override
LockOptions getLockOptions();
/**
* @inheritDoc
*
* This operation is supported even for native queries.
* Note that specifying an explicit lock mode might
* result in changes to the native SQL query that is
* actually executed.
*/
@Override
NativeQuery setLockOptions(LockOptions lockOptions);
/**
* Not applicable to native SQL queries.
*
* @throws IllegalStateException for consistency with JPA
*/
@Override
NativeQuery setLockMode(String alias, LockMode lockMode);
@Override
NativeQuery setComment(String comment);
@Override
NativeQuery addQueryHint(String hint);
@Override
NativeQuery setMaxResults(int maxResult);
@Override
NativeQuery setFirstResult(int startPosition);
@Override
NativeQuery setHint(String hintName, Object value);
/**
* Not applicable to native SQL queries, due to an unfortunate
* requirement of the JPA specification.
*
* Use {@link #getHibernateLockMode()} to obtain the lock mode.
*
* @throws IllegalStateException as required by JPA
*/
@Override
LockModeType getLockMode();
/**
* @inheritDoc
*
* This operation is supported even for native queries.
* Note that specifying an explicit lock mode might
* result in changes to the native SQL query that is
* actually executed.
*/
@Override
LockMode getHibernateLockMode();
/**
* Not applicable to native SQL queries, due to an unfortunate
* requirement of the JPA specification.
*
* Use {@link #setHibernateLockMode(LockMode)} or the hint named
* {@value org.hibernate.jpa.HibernateHints#HINT_NATIVE_LOCK_MODE}
* to set the lock mode.
*
* @throws IllegalStateException as required by JPA
*/
@Override
NativeQuery setLockMode(LockModeType lockMode);
/**
* @inheritDoc
*
* This operation is supported even for native queries.
* Note that specifying an explicit lock mode might
* result in changes to the native SQL query that is
* actually executed.
*/
@Override
NativeQuery setHibernateLockMode(LockMode lockMode);
@Override
NativeQuery setTupleTransformer(TupleTransformer transformer);
@Override
NativeQuery setResultListTransformer(ResultListTransformer transformer);
@Override @Deprecated @SuppressWarnings("deprecation")
NativeQuery setResultTransformer(ResultTransformer transformer);
@Override
NativeQuery setParameter(String name, Object value);
@Override
NativeQuery setParameter(String name, P val, Class type);
@Override
NativeQuery setParameter(String name, P val, BindableType type);
@Override
NativeQuery setParameter(String name, Instant value, TemporalType temporalType);
@Override
NativeQuery setParameter(String name, Calendar value, TemporalType temporalType);
@Override
NativeQuery setParameter(String name, Date value, TemporalType temporalType);
@Override
NativeQuery setParameter(int position, Object value);
@Override
NativeQuery setParameter(int position, P val, Class type);
@Override
NativeQuery setParameter(int position, P val, BindableType type);
@Override
NativeQuery setParameter(int position, Instant value, TemporalType temporalType);
@Override
NativeQuery setParameter(int position, Calendar value, TemporalType temporalType);
@Override
NativeQuery setParameter(int position, Date value, TemporalType temporalType);
@Override
NativeQuery setParameter(QueryParameter parameter, P val);
@Override
NativeQuery setParameter(QueryParameter parameter, P val, Class
type);
@Override
NativeQuery setParameter(QueryParameter parameter, P val, BindableType
type);
@Override
NativeQuery setParameter(Parameter param, P value);
@Override
NativeQuery setParameter(Parameter param, Calendar value, TemporalType temporalType);
@Override
NativeQuery setParameter(Parameter param, Date value, TemporalType temporalType);
@Override
NativeQuery setParameterList(String name, @SuppressWarnings("rawtypes") Collection values);
@Override
NativeQuery setParameterList(String name, Collection extends P> values, Class type);
@Override
NativeQuery setParameterList(String name, Collection extends P> values, BindableType type);
@Override
NativeQuery setParameterList(String name, Object[] values);
@Override
NativeQuery setParameterList(String name, P[] values, Class type);
@Override
NativeQuery setParameterList(String name, P[] values, BindableType type);
@Override
NativeQuery setParameterList(int position, @SuppressWarnings("rawtypes") Collection values);
@Override
NativeQuery setParameterList(int position, Collection extends P> values, Class type);
@Override
NativeQuery setParameterList(int position, Collection extends P> values, BindableType javaType);
@Override
NativeQuery setParameterList(int position, Object[] values);
@Override
NativeQuery setParameterList(int position, P[] values, Class javaType);
@Override
NativeQuery setParameterList(int position, P[] values, BindableType javaType);
@Override
NativeQuery setParameterList(QueryParameter parameter, Collection extends P> values);
@Override
NativeQuery setParameterList(QueryParameter parameter, Collection extends P> values, Class
javaType);
@Override
NativeQuery setParameterList(QueryParameter parameter, Collection extends P> values, BindableType
type);
@Override
NativeQuery setParameterList(QueryParameter parameter, P[] values);
@Override
NativeQuery setParameterList(QueryParameter parameter, P[] values, Class
javaType);
@Override
NativeQuery setParameterList(QueryParameter parameter, P[] values, BindableType
type);
@Override
NativeQuery setProperties(Object bean);
@Override
NativeQuery setProperties(@SuppressWarnings("rawtypes") Map bean);
}