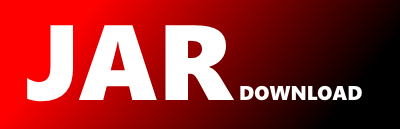
org.beangle.inject.spring.config.SpringBindRegistry Maven / Gradle / Ivy
/*
* Beangle, Agile Development Scaffold and Toolkits.
*
* Copyright © 2005, The Beangle Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.beangle.inject.spring.config;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.beangle.commons.collection.CollectUtils;
import org.beangle.commons.inject.bind.BindRegistry;
import org.beangle.commons.lang.Assert;
import org.beangle.commons.lang.time.Stopwatch;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.PropertyValue;
import org.springframework.beans.factory.FactoryBean;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.config.BeanDefinitionHolder;
import org.springframework.beans.factory.support.BeanDefinitionRegistry;
import org.springframework.util.ClassUtils;
/**
*
* SpringBindRegistry class.
*
*
* @author chaostone
* @version $Id: $
*/
public class SpringBindRegistry implements BindRegistry {
private static final Logger logger = LoggerFactory.getLogger(SpringBindRegistry.class);
protected Map> nameTypes = CollectUtils.newHashMap();
protected Map, List> typeNames = CollectUtils.newHashMap();
protected final BeanDefinitionRegistry definitionRegistry;
/**
*
* getBeanNames.
*
*
* @return a {@link java.util.Set} object.
*/
public Set getBeanNames() {
return nameTypes.keySet();
}
/**
*
* Constructor for SpringBindRegistry.
*
*
* @param registry a {@link org.springframework.beans.factory.support.BeanDefinitionRegistry}
* object.
*/
public SpringBindRegistry(BeanDefinitionRegistry registry) {
Stopwatch watch = new Stopwatch(true);
definitionRegistry = registry;
for (String name : registry.getBeanDefinitionNames()) {
BeanDefinition bd = registry.getBeanDefinition(name);
if (bd.isAbstract()) continue;
// find classname
String className = bd.getBeanClassName();
if (null == className) {
String parentName = bd.getParentName();
if (null == parentName) continue;
else {
BeanDefinition parentDef = registry.getBeanDefinition(parentName);
className = parentDef.getBeanClassName();
}
}
if (null == className) continue;
try {
Class> beanClass = ClassUtils.forName(className, ClassUtils.getDefaultClassLoader());
if (FactoryBean.class.isAssignableFrom(beanClass)) {
register(beanClass, "&" + name);
PropertyValue pv = bd.getPropertyValues().getPropertyValue("target");
if (null == pv) {
Class> artifactClass = ((FactoryBean>) beanClass.newInstance()).getObjectType();
if (null != artifactClass) register(artifactClass, name);
} else {
if (pv.getValue() instanceof BeanDefinitionHolder) {
String nestedClassName = ((BeanDefinitionHolder) pv.getValue()).getBeanDefinition()
.getBeanClassName();
if (null != nestedClassName) {
register(ClassUtils.forName(nestedClassName, ClassUtils.getDefaultClassLoader()), name);
}
}
}
} else {
register(beanClass, name);
}
} catch (Exception e) {
logger.error("class not found", e);
continue;
}
}
logger.info("Init registry ({} beans) in {}", nameTypes.size(), watch);
}
/**
* Get bean name list according given type
*/
public List getBeanNames(Class> type) {
if (typeNames.containsKey(type)) { return typeNames.get(type); }
List names = CollectUtils.newArrayList();
for (Map.Entry> entry : nameTypes.entrySet()) {
if (type.isAssignableFrom(entry.getValue())) {
names.add(entry.getKey());
}
}
typeNames.put(type, names);
return names;
}
/** {@inheritDoc} */
public Class> getBeanType(String beanName) {
return nameTypes.get(beanName);
}
/** {@inheritDoc} */
public boolean contains(String beanName) {
return nameTypes.containsKey(beanName);
}
/**
*
* register.
*
*
* @param type a {@link java.lang.Class} object.
* @param name a {@link java.lang.String} object.
* @param args a {@link java.lang.Object} object.
*/
public void register(Class> type, String name, Object... args) {
Assert.notNull(name, "class'name is null");
if (0 == args.length) {
nameTypes.put(name, type);
} else {
// 注册bean的name和别名
BeanDefinition bd = (BeanDefinition) args[0];
definitionRegistry.registerBeanDefinition(name, bd);
// for list(a.class,b.class) binding usage
String alias = bd.getBeanClassName();
if (bd.isSingleton() && !name.equals(alias) && !definitionRegistry.isBeanNameInUse(alias)) {
definitionRegistry.registerAlias(name, alias);
}
if (null == type) {
if (!bd.isAbstract()) throw new RuntimeException("Concrete bean should has class.");
} else nameTypes.put(name, type);
}
}
public boolean isPrimary(String name) {
return definitionRegistry.getBeanDefinition(name).isPrimary();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy