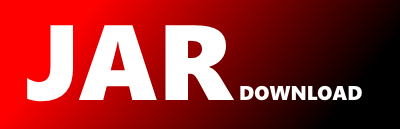
org.beanio.parser.BeanDefinition Maven / Gradle / Ivy
/*
* Copyright 2011 Kevin Seim
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.beanio.parser;
import java.beans.PropertyDescriptor;
import java.lang.reflect.*;
import java.util.*;
import org.beanio.*;
/**
* Property definition that defines a bean class and holds its own list of properties
* for setting on the bean class.
*
* @author Kevin Seim
* @since 1.0
*/
public abstract class BeanDefinition extends PropertyDefinition {
private List propertyList = new ArrayList();
private boolean propertyTypeMap;
/**
* Creates a new BeanDefinition.
*/
public BeanDefinition() { }
/**
* Returns true indicating this property definition defines a
* bean object.
* @return true
*/
@Override
public final boolean isBean() {
return true;
}
/**
* Test if a record matches this record definition.
* @param record the record to test
* @return true if all key fields are matched by this record
*/
public boolean matches(Record record) {
// if the record does not have any key fields, its always a match
for (PropertyDefinition prop : propertyList) {
if (!prop.matches(record)) {
return false;
}
}
return true;
}
/**
* Validates and parses a record into a Java bean.
* @param record the record to parse
* @return the parsed Java bean
* @throws InvalidRecordException
*/
@SuppressWarnings("unchecked")
protected Object parsePropertyValue(Record record) throws InvalidRecordException {
Object[] propertyValue = new Object[propertyList.size()];
// validate and parse each property of this bean
int index = 0;
boolean exists = isBeanExistenceKnown();
for (PropertyDefinition property : propertyList) {
Object value = property.parseValue(record);
if (value != MISSING && !property.isCollection()) {
exists = true;
}
propertyValue[index++] = value;
}
// determine if any bean field was in the input stream
index = 0;
if (!exists) {
// still need to check collections...
for (PropertyDefinition property : propertyList) {
if (property.isCollection()) {
Object value = propertyValue[index];
if (value == null || value == MISSING) {
continue;
}
else if (value == INVALID) {
exists = true;
break;
}
else if (property.isArray()) {
if (Array.getLength(value) > 0) {
exists = true;
break;
}
}
else {
if (!((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy