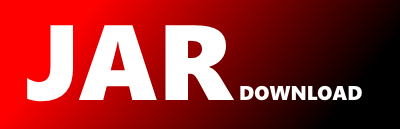
org.beanio.internal.parser.CollectionParser Maven / Gradle / Ivy
/*
* Copyright 2011-2012 Kevin Seim
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.beanio.internal.parser;
import java.io.IOException;
import java.util.*;
import org.beanio.BeanIOException;
/**
* A CollectionParser provides iteration support for a {@link Segment} or {@link Field},
* and is optionally bound to a {@link Collection} type property value.
*
* A CollectionParser must contain exactly one child {@link ParserComponent}.
*
* @author Kevin Seim
* @since 2.0
*/
public class CollectionParser extends Aggregation {
// the collection type
private Class extends Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy