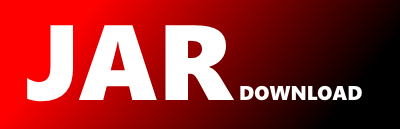
ietf.params.xml.ns.caldav.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.11.10 at 11:42:09 PM EST
//
package ietf.params.xml.ns.caldav;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the ietf.params.xml.ns.caldav package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _Allcomp_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "allcomp");
private final static QName _Allprop_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "allprop");
private final static QName _Prop_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "prop");
private final static QName _Comp_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "comp");
private final static QName _Expand_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "expand");
private final static QName _LimitRecurrenceSet_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "limit-recurrence-set");
private final static QName _LimitFreebusySet_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "limit-freebusy-set");
private final static QName _CalendarData_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "calendar-data");
private final static QName _TextMatch_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "text-match");
private final static QName _IsNotDefined_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "is-not-defined");
private final static QName _Timezone_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "timezone");
private final static QName _TimezoneId_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "timezone-id");
private final static QName _TimeRange_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "time-range");
private final static QName _ParamFilter_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "param-filter");
private final static QName _PropFilter_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "prop-filter");
private final static QName _CompFilter_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "comp-filter");
private final static QName _Filter_QNAME = new QName("urn:ietf:params:xml:ns:caldav", "filter");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: ietf.params.xml.ns.caldav
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AllcompType }
*
*/
public AllcompType createAllcompType() {
return new AllcompType();
}
/**
* Create an instance of {@link AllpropType }
*
*/
public AllpropType createAllpropType() {
return new AllpropType();
}
/**
* Create an instance of {@link PropType }
*
*/
public PropType createPropType() {
return new PropType();
}
/**
* Create an instance of {@link CompType }
*
*/
public CompType createCompType() {
return new CompType();
}
/**
* Create an instance of {@link ExpandType }
*
*/
public ExpandType createExpandType() {
return new ExpandType();
}
/**
* Create an instance of {@link LimitRecurrenceSetType }
*
*/
public LimitRecurrenceSetType createLimitRecurrenceSetType() {
return new LimitRecurrenceSetType();
}
/**
* Create an instance of {@link LimitFreebusySetType }
*
*/
public LimitFreebusySetType createLimitFreebusySetType() {
return new LimitFreebusySetType();
}
/**
* Create an instance of {@link CalendarDataType }
*
*/
public CalendarDataType createCalendarDataType() {
return new CalendarDataType();
}
/**
* Create an instance of {@link TextMatchType }
*
*/
public TextMatchType createTextMatchType() {
return new TextMatchType();
}
/**
* Create an instance of {@link IsNotDefinedType }
*
*/
public IsNotDefinedType createIsNotDefinedType() {
return new IsNotDefinedType();
}
/**
* Create an instance of {@link TimezoneType }
*
*/
public TimezoneType createTimezoneType() {
return new TimezoneType();
}
/**
* Create an instance of {@link TimezoneIdType }
*
*/
public TimezoneIdType createTimezoneIdType() {
return new TimezoneIdType();
}
/**
* Create an instance of {@link UTCTimeRangeType }
*
*/
public UTCTimeRangeType createUTCTimeRangeType() {
return new UTCTimeRangeType();
}
/**
* Create an instance of {@link ParamFilterType }
*
*/
public ParamFilterType createParamFilterType() {
return new ParamFilterType();
}
/**
* Create an instance of {@link PropFilterType }
*
*/
public PropFilterType createPropFilterType() {
return new PropFilterType();
}
/**
* Create an instance of {@link CompFilterType }
*
*/
public CompFilterType createCompFilterType() {
return new CompFilterType();
}
/**
* Create an instance of {@link FilterType }
*
*/
public FilterType createFilterType() {
return new FilterType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AllcompType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AllcompType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "allcomp")
public JAXBElement createAllcomp(AllcompType value) {
return new JAXBElement(_Allcomp_QNAME, AllcompType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AllpropType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AllpropType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "allprop")
public JAXBElement createAllprop(AllpropType value) {
return new JAXBElement(_Allprop_QNAME, AllpropType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PropType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PropType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "prop")
public JAXBElement createProp(PropType value) {
return new JAXBElement(_Prop_QNAME, PropType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CompType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CompType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "comp")
public JAXBElement createComp(CompType value) {
return new JAXBElement(_Comp_QNAME, CompType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExpandType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExpandType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "expand")
public JAXBElement createExpand(ExpandType value) {
return new JAXBElement(_Expand_QNAME, ExpandType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LimitRecurrenceSetType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LimitRecurrenceSetType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "limit-recurrence-set")
public JAXBElement createLimitRecurrenceSet(LimitRecurrenceSetType value) {
return new JAXBElement(_LimitRecurrenceSet_QNAME, LimitRecurrenceSetType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LimitFreebusySetType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LimitFreebusySetType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "limit-freebusy-set")
public JAXBElement createLimitFreebusySet(LimitFreebusySetType value) {
return new JAXBElement(_LimitFreebusySet_QNAME, LimitFreebusySetType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CalendarDataType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CalendarDataType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "calendar-data")
public JAXBElement createCalendarData(CalendarDataType value) {
return new JAXBElement(_CalendarData_QNAME, CalendarDataType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TextMatchType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TextMatchType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "text-match")
public JAXBElement createTextMatch(TextMatchType value) {
return new JAXBElement(_TextMatch_QNAME, TextMatchType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IsNotDefinedType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link IsNotDefinedType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "is-not-defined")
public JAXBElement createIsNotDefined(IsNotDefinedType value) {
return new JAXBElement(_IsNotDefined_QNAME, IsNotDefinedType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TimezoneType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TimezoneType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "timezone")
public JAXBElement createTimezone(TimezoneType value) {
return new JAXBElement(_Timezone_QNAME, TimezoneType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TimezoneIdType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TimezoneIdType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "timezone-id")
public JAXBElement createTimezoneId(TimezoneIdType value) {
return new JAXBElement(_TimezoneId_QNAME, TimezoneIdType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link UTCTimeRangeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link UTCTimeRangeType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "time-range")
public JAXBElement createTimeRange(UTCTimeRangeType value) {
return new JAXBElement(_TimeRange_QNAME, UTCTimeRangeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParamFilterType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParamFilterType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "param-filter")
public JAXBElement createParamFilter(ParamFilterType value) {
return new JAXBElement(_ParamFilter_QNAME, ParamFilterType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PropFilterType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PropFilterType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "prop-filter")
public JAXBElement createPropFilter(PropFilterType value) {
return new JAXBElement(_PropFilter_QNAME, PropFilterType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CompFilterType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CompFilterType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "comp-filter")
public JAXBElement createCompFilter(CompFilterType value) {
return new JAXBElement(_CompFilter_QNAME, CompFilterType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FilterType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FilterType }{@code >}
*/
@XmlElementDecl(namespace = "urn:ietf:params:xml:ns:caldav", name = "filter")
public JAXBElement createFilter(FilterType value) {
return new JAXBElement(_Filter_QNAME, FilterType.class, null, value);
}
}